mirror of
https://github.com/holub/mame
synced 2025-07-11 20:54:11 +03:00
Updated BGFX, BX and GENie to latest (nw)
This commit is contained in:
parent
20b68364f7
commit
8afe66fc63
1
3rdparty/bgfx/.gitignore
vendored
1
3rdparty/bgfx/.gitignore
vendored
@ -4,4 +4,3 @@
|
||||
.git
|
||||
.svn
|
||||
tags
|
||||
src/renderer_d3d12.*
|
||||
|
1704
3rdparty/bgfx/3rdparty/ocornut-imgui/imgui.cpp
vendored
1704
3rdparty/bgfx/3rdparty/ocornut-imgui/imgui.cpp
vendored
File diff suppressed because it is too large
Load Diff
177
3rdparty/bgfx/3rdparty/ocornut-imgui/imgui.h
vendored
177
3rdparty/bgfx/3rdparty/ocornut-imgui/imgui.h
vendored
@ -1,5 +1,5 @@
|
||||
// ImGui library v1.30
|
||||
// See .cpp file for commentary.
|
||||
// ImGui library v1.32 wip
|
||||
// See .cpp file for documentation.
|
||||
// See ImGui::ShowTestWindow() for sample code.
|
||||
// Read 'Programmer guide' in .cpp for notes on how to setup ImGui in your codebase.
|
||||
// Get latest version at https://github.com/ocornut/imgui
|
||||
@ -43,7 +43,7 @@ typedef int ImGuiColorEditMode; // enum ImGuiColorEditMode_
|
||||
typedef int ImGuiWindowFlags; // enum ImGuiWindowFlags_
|
||||
typedef int ImGuiSetCondition; // enum ImGuiSetCondition_
|
||||
typedef int ImGuiInputTextFlags; // enum ImGuiInputTextFlags_
|
||||
struct ImGuiTextEditCallbackData; // for advanced uses of InputText()
|
||||
struct ImGuiTextEditCallbackData; // for advanced uses of InputText()
|
||||
|
||||
struct ImVec2
|
||||
{
|
||||
@ -69,13 +69,12 @@ struct ImVec4
|
||||
|
||||
namespace ImGui
|
||||
{
|
||||
// Proxy functions to access the MemAllocFn/MemFreeFn/MemReallocFn pointers in ImGui::GetIO(). The only reason they exist here is to allow ImVector<> to compile inline.
|
||||
// Proxy functions to access the MemAllocFn/MemFreeFn pointers in ImGui::GetIO(). The only reason they exist here is to allow ImVector<> to compile inline.
|
||||
IMGUI_API void* MemAlloc(size_t sz);
|
||||
IMGUI_API void MemFree(void* ptr);
|
||||
IMGUI_API void* MemRealloc(void* ptr, size_t sz);
|
||||
}
|
||||
|
||||
// std::vector<> like class to avoid dragging dependencies (also: windows implementation of STL with debug enabled is absurdly slow, so let's bypass it so our code runs fast in debug).
|
||||
// std::vector<> like class to avoid dragging dependencies (also: windows implementation of STL with debug enabled is absurdly slow, so let's bypass it so our code runs fast in debug).
|
||||
// Use '#define ImVector std::vector' if you want to use the STL type or your own type.
|
||||
// Our implementation does NOT call c++ constructors! because the data types we use don't need them (but that could be added as well). Only provide the minimum functionalities we need.
|
||||
#ifndef ImVector
|
||||
@ -115,8 +114,16 @@ public:
|
||||
inline const value_type& back() const { IM_ASSERT(Size > 0); return Data[Size-1]; }
|
||||
inline void swap(ImVector<T>& rhs) { const size_t rhs_size = rhs.Size; rhs.Size = Size; Size = rhs_size; const size_t rhs_cap = rhs.Capacity; rhs.Capacity = Capacity; Capacity = rhs_cap; value_type* rhs_data = rhs.Data; rhs.Data = Data; Data = rhs_data; }
|
||||
|
||||
inline void reserve(size_t new_capacity) { Data = (value_type*)ImGui::MemRealloc(Data, new_capacity * sizeof(value_type)); Capacity = new_capacity; }
|
||||
inline void resize(size_t new_size) { if (new_size > Capacity) reserve(new_size); Size = new_size; }
|
||||
inline void reserve(size_t new_capacity)
|
||||
{
|
||||
if (new_capacity <= Capacity) return;
|
||||
T* new_data = (value_type*)ImGui::MemAlloc(new_capacity * sizeof(value_type));
|
||||
memcpy(new_data, Data, Size * sizeof(value_type));
|
||||
ImGui::MemFree(Data);
|
||||
Data = new_data;
|
||||
Capacity = new_capacity;
|
||||
}
|
||||
|
||||
inline void push_back(const value_type& v) { if (Size == Capacity) reserve(Capacity ? Capacity * 2 : 4); Data[Size++] = v; }
|
||||
inline void pop_back() { IM_ASSERT(Size > 0); Size--; }
|
||||
@ -152,25 +159,26 @@ namespace ImGui
|
||||
IMGUI_API bool Begin(const char* name = "Debug", bool* p_opened = NULL, ImVec2 size = ImVec2(0,0), float fill_alpha = -1.0f, ImGuiWindowFlags flags = 0);// return false when window is collapsed, so you can early out in your code. passing 'bool* p_opened' displays a Close button on the upper-right corner of the window, the pointed value will be set to false when the button is pressed.
|
||||
IMGUI_API void End();
|
||||
IMGUI_API void BeginChild(const char* str_id, ImVec2 size = ImVec2(0,0), bool border = false, ImGuiWindowFlags extra_flags = 0); // size==0.0f: use remaining window size, size<0.0f: use remaining window size minus abs(size). on each axis.
|
||||
IMGUI_API void BeginChild(ImGuiID id, ImVec2 size = ImVec2(0,0), bool border = false, ImGuiWindowFlags extra_flags = 0); // "
|
||||
IMGUI_API void EndChild();
|
||||
IMGUI_API bool GetWindowIsFocused();
|
||||
IMGUI_API ImVec2 GetContentRegionMax(); // window or current column boundaries
|
||||
IMGUI_API ImVec2 GetWindowContentRegionMin(); // window boundaries
|
||||
IMGUI_API ImVec2 GetContentRegionMax(); // window or current column boundaries, in windows coordinates
|
||||
IMGUI_API ImVec2 GetWindowContentRegionMin(); // window boundaries, in windows coordinates
|
||||
IMGUI_API ImVec2 GetWindowContentRegionMax();
|
||||
IMGUI_API ImDrawList* GetWindowDrawList(); // get rendering command-list if you want to append your own draw primitives.
|
||||
IMGUI_API ImFont* GetWindowFont();
|
||||
IMGUI_API float GetWindowFontSize();
|
||||
IMGUI_API float GetWindowFontSize(); // size (also height in pixels) of current font with current scale applied
|
||||
IMGUI_API void SetWindowFontScale(float scale); // per-window font scale. Adjust IO.FontGlobalScale if you want to scale all windows.
|
||||
IMGUI_API ImVec2 GetWindowPos(); // you should rarely need/care about the window position, but it can be useful if you want to do your own drawing.
|
||||
IMGUI_API ImVec2 GetWindowSize(); // get current window position.
|
||||
IMGUI_API float GetWindowWidth();
|
||||
IMGUI_API bool GetWindowCollapsed();
|
||||
IMGUI_API void SetWindowPos(const ImVec2& pos, ImGuiSetCondition cond = 0); // set current window position - call within Begin()/End().
|
||||
IMGUI_API void SetWindowSize(const ImVec2& size, ImGuiSetCondition cond = 0); // set current window size. set to ImVec2(0,0) to force an auto-fit
|
||||
IMGUI_API void SetWindowCollapsed(bool collapsed, ImGuiSetCondition cond = 0); // set current window collapsed state.
|
||||
IMGUI_API void SetNextWindowPos(const ImVec2& pos, ImGuiSetCondition cond = 0); // set next window position - call before Begin().
|
||||
IMGUI_API void SetNextWindowSize(const ImVec2& size, ImGuiSetCondition cond = 0); // set next window size. set to ImVec2(0,0) to force an auto-fit
|
||||
IMGUI_API void SetNextWindowSize(const ImVec2& size, ImGuiSetCondition cond = 0); // set next window size. set to ImVec2(0,0) to force an auto-fit.
|
||||
IMGUI_API void SetNextWindowCollapsed(bool collapsed, ImGuiSetCondition cond = 0); // set next window collapsed state.
|
||||
IMGUI_API void SetWindowPos(const ImVec2& pos, ImGuiSetCondition cond = 0); // set current window position - call within Begin()/End(). may incur tearing.
|
||||
IMGUI_API void SetWindowSize(const ImVec2& size, ImGuiSetCondition cond = 0); // set current window size. set to ImVec2(0,0) to force an auto-fit. may incur tearing.
|
||||
IMGUI_API void SetWindowCollapsed(bool collapsed, ImGuiSetCondition cond = 0); // set current window collapsed state.
|
||||
|
||||
IMGUI_API void SetScrollPosHere(); // adjust scrolling position to center into the current cursor position.
|
||||
IMGUI_API void SetKeyboardFocusHere(int offset = 0); // focus keyboard on the next widget. Use positive 'offset' to access sub components of a multiple component widget.
|
||||
@ -187,9 +195,9 @@ namespace ImGui
|
||||
IMGUI_API void PopStyleVar(int count = 1);
|
||||
|
||||
// Parameters stacks (current window)
|
||||
IMGUI_API void PushItemWidth(float item_width); // width of items for the common item+label case. default to ~2/3 of windows width.
|
||||
IMGUI_API void PushItemWidth(float item_width); // width of items for the common item+label case, pixels. 0.0f = default to ~2/3 of windows width, >0.0f: width in pixels, <0.0f align xx pixels to the right of window (so -0.01f always align width to the right side)
|
||||
IMGUI_API void PopItemWidth();
|
||||
IMGUI_API float GetItemWidth();
|
||||
IMGUI_API float CalcItemWidth(); // width of item given pushed settings and current cursor position
|
||||
IMGUI_API void PushAllowKeyboardFocus(bool v); // allow focusing using TAB/Shift-TAB, enabled by default but you can disable it for certain widgets.
|
||||
IMGUI_API void PopAllowKeyboardFocus();
|
||||
IMGUI_API void PushTextWrapPos(float wrap_pos_x = 0.0f); // word-wrapping for Text*() commands. < 0.0f: no wrapping; 0.0f: wrap to end of window (or column); > 0.0f: wrap at 'wrap_pos_x' position in window local space.
|
||||
@ -211,14 +219,16 @@ namespace ImGui
|
||||
IMGUI_API void SetColumnOffset(int column_index, float offset);
|
||||
IMGUI_API float GetColumnWidth(int column_index = -1);
|
||||
IMGUI_API ImVec2 GetCursorPos(); // cursor position is relative to window position
|
||||
IMGUI_API float GetCursorPosX(); // "
|
||||
IMGUI_API float GetCursorPosY(); // "
|
||||
IMGUI_API void SetCursorPos(const ImVec2& pos); // "
|
||||
IMGUI_API void SetCursorPosX(float x); // "
|
||||
IMGUI_API void SetCursorPosY(float y); // "
|
||||
IMGUI_API ImVec2 GetCursorScreenPos(); // cursor position in absolute screen coordinates (0..io.DisplaySize)
|
||||
IMGUI_API void SetCursorScreenPos(const ImVec2& pos); // cursor position in absolute screen coordinates (0..io.DisplaySize)
|
||||
IMGUI_API void AlignFirstTextHeightToWidgets(); // call once if the first item on the line is a Text() item and you want to vertically lower it to match subsequent (bigger) widgets.
|
||||
IMGUI_API float GetTextLineSpacing();
|
||||
IMGUI_API float GetTextLineHeight();
|
||||
IMGUI_API float GetTextLineHeight(); // height of font == GetWindowFontSize()
|
||||
IMGUI_API float GetTextLineHeightWithSpacing(); // spacing (in pixels) between 2 consecutive lines of text == GetWindowFontSize() + GetStyle().ItemSpacing.y
|
||||
|
||||
// ID scopes
|
||||
// If you are creating repeated widgets in a loop you most likely want to push a unique identifier so ImGui can differentiate them.
|
||||
@ -238,7 +248,7 @@ namespace ImGui
|
||||
IMGUI_API void TextWrapped(const char* fmt, ...); // shortcut for PushTextWrapPos(0.0f); Text(fmt, ...); PopTextWrapPos();
|
||||
IMGUI_API void TextWrappedV(const char* fmt, va_list args);
|
||||
IMGUI_API void TextUnformatted(const char* text, const char* text_end = NULL); // doesn't require null terminated string if 'text_end' is specified. no copy done to any bounded stack buffer, recommended for long chunks of text.
|
||||
IMGUI_API void LabelText(const char* label, const char* fmt, ...); // display text+label aligned the same way as value+label widgets
|
||||
IMGUI_API void LabelText(const char* label, const char* fmt, ...); // display text+label aligned the same way as value+label widgets
|
||||
IMGUI_API void LabelTextV(const char* label, const char* fmt, va_list args);
|
||||
IMGUI_API void BulletText(const char* fmt, ...);
|
||||
IMGUI_API void BulletTextV(const char* fmt, va_list args);
|
||||
@ -246,7 +256,7 @@ namespace ImGui
|
||||
IMGUI_API bool SmallButton(const char* label);
|
||||
IMGUI_API bool InvisibleButton(const char* str_id, const ImVec2& size);
|
||||
IMGUI_API void Image(ImTextureID user_texture_id, const ImVec2& size, const ImVec2& uv0 = ImVec2(0,0), const ImVec2& uv1 = ImVec2(1,1), const ImVec4& tint_col = ImVec4(1,1,1,1), const ImVec4& border_col = ImVec4(0,0,0,0));
|
||||
IMGUI_API bool ImageButton(ImTextureID user_texture_id, const ImVec2& size, const ImVec2& uv0 = ImVec2(0,0), const ImVec2& uv1 = ImVec2(1,1), int frame_padding = -1, const ImVec4& bg_col = ImVec4(0,0,0,1)); // <0 frame_padding uses default frame padding settings. 0 for no paddnig.
|
||||
IMGUI_API bool ImageButton(ImTextureID user_texture_id, const ImVec2& size, const ImVec2& uv0 = ImVec2(0,0), const ImVec2& uv1 = ImVec2(1,1), int frame_padding = -1, const ImVec4& bg_col = ImVec4(0,0,0,1), const ImVec4& tint_col = ImVec4(1,1,1,1)); // <0 frame_padding uses default frame padding settings. 0 for no paddnig.
|
||||
IMGUI_API bool CollapsingHeader(const char* label, const char* str_id = NULL, const bool display_frame = true, const bool default_open = false);
|
||||
IMGUI_API bool SliderFloat(const char* label, float* v, float v_min, float v_max, const char* display_format = "%.3f", float power = 1.0f); // adjust display_format to decorate the value with a prefix or a suffix. Use power!=1.0 for logarithmic sliders.
|
||||
IMGUI_API bool SliderFloat2(const char* label, float v[2], float v_min, float v_max, const char* display_format = "%.3f", float power = 1.0f);
|
||||
@ -271,9 +281,9 @@ namespace ImGui
|
||||
IMGUI_API bool InputFloat3(const char* label, float v[3], int decimal_precision = -1);
|
||||
IMGUI_API bool InputFloat4(const char* label, float v[4], int decimal_precision = -1);
|
||||
IMGUI_API bool InputInt(const char* label, int* v, int step = 1, int step_fast = 100, ImGuiInputTextFlags extra_flags = 0);
|
||||
IMGUI_API bool Combo(const char* label, int* current_item, const char** items, int items_count, int popup_height_items = 7);
|
||||
IMGUI_API bool Combo(const char* label, int* current_item, const char* items_separated_by_zeros, int popup_height_items = 7); // separate items with \0, end item-list with \0\0
|
||||
IMGUI_API bool Combo(const char* label, int* current_item, bool (*items_getter)(void* data, int idx, const char** out_text), void* data, int items_count, int popup_height_items = 7);
|
||||
IMGUI_API bool Combo(const char* label, int* current_item, const char** items, int items_count, int height_in_items = -1);
|
||||
IMGUI_API bool Combo(const char* label, int* current_item, const char* items_separated_by_zeros, int height_in_items = -1); // separate items with \0, end item-list with \0\0
|
||||
IMGUI_API bool Combo(const char* label, int* current_item, bool (*items_getter)(void* data, int idx, const char** out_text), void* data, int items_count, int height_in_items = -1);
|
||||
IMGUI_API bool ColorButton(const ImVec4& col, bool small_height = false, bool outline_border = true);
|
||||
IMGUI_API bool ColorEdit3(const char* label, float col[3]);
|
||||
IMGUI_API bool ColorEdit4(const char* label, float col[4], bool show_alpha = true);
|
||||
@ -288,6 +298,15 @@ namespace ImGui
|
||||
IMGUI_API void TreePop();
|
||||
IMGUI_API void OpenNextNode(bool open); // force open/close the next TreeNode or CollapsingHeader
|
||||
|
||||
// Selectable / Lists
|
||||
IMGUI_API bool Selectable(const char* label, bool selected, const ImVec2& size = ImVec2(0,0));
|
||||
IMGUI_API bool Selectable(const char* label, bool* p_selected, const ImVec2& size = ImVec2(0,0));
|
||||
IMGUI_API bool ListBox(const char* label, int* current_item, const char** items, int items_count, int height_in_items = -1);
|
||||
IMGUI_API bool ListBox(const char* label, int* current_item, bool (*items_getter)(void* data, int idx, const char** out_text), void* data, int items_count, int height_in_items = -1);
|
||||
IMGUI_API bool ListBoxHeader(const char* label, const ImVec2& size = ImVec2(0,0)); // use if you want to reimplement ListBox() will custom data or interactions. make sure to call ListBoxFooter() afterwards.
|
||||
IMGUI_API bool ListBoxHeader(const char* label, int items_count, int height_in_items = -1); // "
|
||||
IMGUI_API void ListBoxFooter(); // terminate the scrolling region
|
||||
|
||||
// Value() Helpers: output single value in "name: value" format. Tip: freely declare your own within the ImGui namespace!
|
||||
IMGUI_API void Value(const char* prefix, bool b);
|
||||
IMGUI_API void Value(const char* prefix, int v);
|
||||
@ -322,11 +341,20 @@ namespace ImGui
|
||||
IMGUI_API int GetFrameCount();
|
||||
IMGUI_API const char* GetStyleColName(ImGuiCol idx);
|
||||
IMGUI_API ImVec2 CalcTextSize(const char* text, const char* text_end = NULL, bool hide_text_after_double_hash = false, float wrap_width = -1.0f);
|
||||
IMGUI_API void CalcListClipping(int items_count, float items_height, int* out_items_display_start, int* out_items_display_end); // helper to manually clip large list of items. see comments in implementation.
|
||||
|
||||
IMGUI_API void BeginChildFrame(ImGuiID id, const ImVec2& size); // helper to create a child window / scrolling region that looks like a normal widget frame.
|
||||
IMGUI_API void EndChildFrame();
|
||||
|
||||
IMGUI_API ImU32 ColorConvertFloat4ToU32(const ImVec4& in);
|
||||
IMGUI_API void ColorConvertRGBtoHSV(float r, float g, float b, float& out_h, float& out_s, float& out_v);
|
||||
IMGUI_API void ColorConvertHSVtoRGB(float h, float s, float v, float& out_r, float& out_g, float& out_b);
|
||||
|
||||
// Internal state access - if you want to share ImGui state between modules (e.g. DLL) or allocate it yourself
|
||||
IMGUI_API void* GetInternalState();
|
||||
IMGUI_API size_t GetInternalStateSize();
|
||||
IMGUI_API void SetInternalState(void* state, bool construct = false);
|
||||
|
||||
// Obsolete (will be removed)
|
||||
IMGUI_API void GetDefaultFontData(const void** fnt_data, unsigned int* fnt_size, const void** png_data, unsigned int* png_size);
|
||||
|
||||
@ -336,19 +364,21 @@ namespace ImGui
|
||||
enum ImGuiWindowFlags_
|
||||
{
|
||||
// Default: 0
|
||||
ImGuiWindowFlags_ShowBorders = 1 << 0,
|
||||
ImGuiWindowFlags_NoTitleBar = 1 << 1,
|
||||
ImGuiWindowFlags_NoResize = 1 << 2,
|
||||
ImGuiWindowFlags_NoMove = 1 << 3,
|
||||
ImGuiWindowFlags_NoScrollbar = 1 << 4,
|
||||
ImGuiWindowFlags_NoScrollWithMouse = 1 << 5,
|
||||
ImGuiWindowFlags_AlwaysAutoResize = 1 << 6,
|
||||
ImGuiWindowFlags_NoSavedSettings = 1 << 7, // Never load/save settings in .ini file
|
||||
ImGuiWindowFlags_ChildWindow = 1 << 8, // For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ChildWindowAutoFitX = 1 << 9, // For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ChildWindowAutoFitY = 1 << 10, // For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ComboBox = 1 << 11, // For internal use by ComboBox()
|
||||
ImGuiWindowFlags_Tooltip = 1 << 12 // For internal use by Render() when using Tooltip
|
||||
ImGuiWindowFlags_NoTitleBar = 1 << 0, // Disable title-bar
|
||||
ImGuiWindowFlags_NoResize = 1 << 1, // Disable user resizing with the lower-right grip
|
||||
ImGuiWindowFlags_NoMove = 1 << 2, // Disable user moving the window
|
||||
ImGuiWindowFlags_NoScrollbar = 1 << 3, // Disable scroll bar (window can still scroll with mouse or programatically)
|
||||
ImGuiWindowFlags_NoScrollWithMouse = 1 << 4, // Disable user scrolling with mouse wheel
|
||||
ImGuiWindowFlags_NoCollapse = 1 << 5, // Disable user collapsing window by double-clicking on it
|
||||
ImGuiWindowFlags_AlwaysAutoResize = 1 << 6, // Resize every window to its content every frame
|
||||
ImGuiWindowFlags_ShowBorders = 1 << 7, // Show borders around windows and items
|
||||
ImGuiWindowFlags_NoSavedSettings = 1 << 8, // Never load/save settings in .ini file
|
||||
// [Internal]
|
||||
ImGuiWindowFlags_ChildWindow = 1 << 9, // For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ChildWindowAutoFitX = 1 << 10, // For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ChildWindowAutoFitY = 1 << 11, // For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ComboBox = 1 << 12, // For internal use by ComboBox()
|
||||
ImGuiWindowFlags_Tooltip = 1 << 13 // For internal use by BeginTooltip()
|
||||
};
|
||||
|
||||
// Flags for ImGui::InputText()
|
||||
@ -393,6 +423,7 @@ enum ImGuiCol_
|
||||
{
|
||||
ImGuiCol_Text,
|
||||
ImGuiCol_WindowBg,
|
||||
ImGuiCol_ChildWindowBg,
|
||||
ImGuiCol_Border,
|
||||
ImGuiCol_BorderShadow,
|
||||
ImGuiCol_FrameBg, // Background of checkbox, radio button, plot, slider, text input
|
||||
@ -436,14 +467,15 @@ enum ImGuiCol_
|
||||
// NB: the enum only refers to fields of ImGuiStyle() which makes sense to be pushed/poped in UI code. Feel free to add others.
|
||||
enum ImGuiStyleVar_
|
||||
{
|
||||
ImGuiStyleVar_Alpha, // float
|
||||
ImGuiStyleVar_WindowPadding, // ImVec2
|
||||
ImGuiStyleVar_WindowRounding, // float
|
||||
ImGuiStyleVar_FramePadding, // ImVec2
|
||||
ImGuiStyleVar_FrameRounding, // float
|
||||
ImGuiStyleVar_ItemSpacing, // ImVec2
|
||||
ImGuiStyleVar_ItemInnerSpacing, // ImVec2
|
||||
ImGuiStyleVar_TreeNodeSpacing // float
|
||||
ImGuiStyleVar_Alpha, // float
|
||||
ImGuiStyleVar_WindowPadding, // ImVec2
|
||||
ImGuiStyleVar_WindowRounding, // float
|
||||
ImGuiStyleVar_ChildWindowRounding, // float
|
||||
ImGuiStyleVar_FramePadding, // ImVec2
|
||||
ImGuiStyleVar_FrameRounding, // float
|
||||
ImGuiStyleVar_ItemSpacing, // ImVec2
|
||||
ImGuiStyleVar_ItemInnerSpacing, // ImVec2
|
||||
ImGuiStyleVar_TreeNodeSpacing // float
|
||||
};
|
||||
|
||||
// Enumeration for ColorEditMode()
|
||||
@ -471,6 +503,7 @@ struct ImGuiStyle
|
||||
ImVec2 WindowPadding; // Padding within a window
|
||||
ImVec2 WindowMinSize; // Minimum window size
|
||||
float WindowRounding; // Radius of window corners rounding. Set to 0.0f to have rectangular windows
|
||||
float ChildWindowRounding; // Radius of child window corners rounding. Set to 0.0f to have rectangular windows
|
||||
ImVec2 FramePadding; // Padding within a framed rectangle (used by most widgets)
|
||||
float FrameRounding; // Radius of frame corners rounding. Set to 0.0f to have rectangular frame (used by most widgets).
|
||||
ImVec2 ItemSpacing; // Horizontal and vertical spacing between widgets/lines
|
||||
@ -514,18 +547,17 @@ struct ImGuiIO
|
||||
// User Functions
|
||||
//------------------------------------------------------------------
|
||||
|
||||
// REQUIRED: rendering function.
|
||||
// REQUIRED: rendering function.
|
||||
// See example code if you are unsure of how to implement this.
|
||||
void (*RenderDrawListsFn)(ImDrawList** const draw_lists, int count);
|
||||
void (*RenderDrawListsFn)(ImDrawList** const draw_lists, int count);
|
||||
|
||||
// Optional: access OS clipboard (default to use native Win32 clipboard on Windows, otherwise use a ImGui private clipboard)
|
||||
// Override to access OS clipboard on other architectures.
|
||||
const char* (*GetClipboardTextFn)();
|
||||
void (*SetClipboardTextFn)(const char* text);
|
||||
|
||||
// Optional: override memory allocations (default to posix malloc/realloc/free)
|
||||
// Optional: override memory allocations (default to posix malloc/free). MemFreeFn() may be called with a NULL pointer.
|
||||
void* (*MemAllocFn)(size_t sz);
|
||||
void* (*MemReallocFn)(void* ptr, size_t sz);
|
||||
void (*MemFreeFn)(void* ptr);
|
||||
|
||||
// Optional: notify OS Input Method Editor of the screen position of your cursor for text input position (e.g. when using Japanese/Chinese inputs in Windows)
|
||||
@ -537,7 +569,7 @@ struct ImGuiIO
|
||||
|
||||
ImVec2 MousePos; // Mouse position, in pixels (set to -1,-1 if no mouse / on another screen, etc.)
|
||||
bool MouseDown[5]; // Mouse buttons. ImGui itself only uses button 0 (left button) but you can use others as storage for convenience.
|
||||
float MouseWheel; // Mouse wheel: 1 unit scrolls about 5 lines text.
|
||||
float MouseWheel; // Mouse wheel: 1 unit scrolls about 5 lines text.
|
||||
bool MouseDrawCursor; // Request ImGui to draw a mouse cursor for you (if you are on a platform without a mouse cursor).
|
||||
bool KeyCtrl; // Keyboard modifier pressed: Control
|
||||
bool KeyShift; // Keyboard modifier pressed: Shift
|
||||
@ -558,14 +590,15 @@ struct ImGuiIO
|
||||
// [Internal] ImGui will maintain those fields for you
|
||||
//------------------------------------------------------------------
|
||||
|
||||
ImVec2 MousePosPrev;
|
||||
ImVec2 MouseDelta;
|
||||
bool MouseClicked[5];
|
||||
ImVec2 MouseClickedPos[5];
|
||||
float MouseClickedTime[5];
|
||||
bool MouseDoubleClicked[5];
|
||||
float MouseDownTime[5];
|
||||
float KeysDownTime[512];
|
||||
ImVec2 MousePosPrev; //
|
||||
ImVec2 MouseDelta; // Mouse delta. Note that this is zero if either current or previous position are negative to allow mouse enabling/disabling.
|
||||
bool MouseClicked[5]; // Mouse button went from !Down to Down
|
||||
ImVec2 MouseClickedPos[5]; // Position at time of clicking
|
||||
float MouseClickedTime[5]; // Time of last click (used to figure out double-click)
|
||||
bool MouseDoubleClicked[5]; // Has mouse button been double-clicked?
|
||||
bool MouseDownOwned[5]; // Track if button was clicked inside a window. We don't request mouse capture from the application if click started outside ImGui bounds.
|
||||
float MouseDownTime[5]; // Time the mouse button has been down
|
||||
float KeysDownTime[512]; // Time the keyboard key has been down
|
||||
|
||||
IMGUI_API ImGuiIO();
|
||||
};
|
||||
@ -647,13 +680,13 @@ struct ImGuiTextBuffer
|
||||
// - You want to store custom debug data easily without adding or editing structures in your code.
|
||||
struct ImGuiStorage
|
||||
{
|
||||
struct Pair
|
||||
{
|
||||
ImGuiID key;
|
||||
union { int val_i; float val_f; void* val_p; };
|
||||
Pair(ImGuiID _key, int _val_i) { key = _key; val_i = _val_i; }
|
||||
Pair(ImGuiID _key, float _val_f) { key = _key; val_f = _val_f; }
|
||||
Pair(ImGuiID _key, void* _val_p) { key = _key; val_p = _val_p; }
|
||||
struct Pair
|
||||
{
|
||||
ImGuiID key;
|
||||
union { int val_i; float val_f; void* val_p; };
|
||||
Pair(ImGuiID _key, int _val_i) { key = _key; val_i = _val_i; }
|
||||
Pair(ImGuiID _key, float _val_f) { key = _key; val_f = _val_f; }
|
||||
Pair(ImGuiID _key, void* _val_p) { key = _key; val_p = _val_p; }
|
||||
};
|
||||
ImVector<Pair> Data;
|
||||
|
||||
@ -668,7 +701,7 @@ struct ImGuiStorage
|
||||
IMGUI_API void* GetVoidPtr(ImGuiID key) const; // default_val is NULL
|
||||
IMGUI_API void SetVoidPtr(ImGuiID key, void* val);
|
||||
|
||||
// - Get***Ref() functions finds pair, insert on demand if missing, return pointer. Useful if you intend to do Get+Set.
|
||||
// - Get***Ref() functions finds pair, insert on demand if missing, return pointer. Useful if you intend to do Get+Set.
|
||||
// - References are only valid until a new value is added to the storage. Calling a Set***() function or a Get***Ref() function invalidates the pointer.
|
||||
// - A typical use case where this is convenient:
|
||||
// float* pvar = ImGui::GetFloatRef(key); ImGui::SliderFloat("var", pvar, 0, 100.0f); some_var += *pvar;
|
||||
@ -683,7 +716,7 @@ struct ImGuiStorage
|
||||
// Shared state of InputText(), passed to callback when a ImGuiInputTextFlags_Callback* flag is used.
|
||||
struct ImGuiTextEditCallbackData
|
||||
{
|
||||
ImGuiKey EventKey; // Key pressed (Up/Down/TAB) // Read-only
|
||||
ImGuiKey EventKey; // Key pressed (Up/Down/TAB) // Read-only
|
||||
char* Buf; // Current text // Read-write (pointed data only)
|
||||
size_t BufSize; // // Read-only
|
||||
bool BufDirty; // Set if you modify Buf directly // Write
|
||||
@ -704,7 +737,7 @@ struct ImColor
|
||||
{
|
||||
ImVec4 Value;
|
||||
|
||||
ImColor(int r, int g, int b, int a = 255) { Value.x = r / 255.0f; Value.y = g / 255.0f; Value.z = b / 255.0f; Value.w = a / 255.0f; }
|
||||
ImColor(int r, int g, int b, int a = 255) { Value.x = (float)r / 255.0f; Value.y = (float)g / 255.0f; Value.z = (float)b / 255.0f; Value.w = (float)a / 255.0f; }
|
||||
ImColor(float r, float g, float b, float a = 1.0f) { Value.x = r; Value.y = g; Value.z = b; Value.w = a; }
|
||||
ImColor(const ImVec4& col) { Value = col; }
|
||||
|
||||
@ -769,17 +802,17 @@ struct ImDrawList
|
||||
|
||||
// [Internal to ImGui]
|
||||
ImVector<ImVec4> clip_rect_stack; // [Internal]
|
||||
ImVector<ImTextureID> texture_id_stack; // [Internal]
|
||||
ImVector<ImTextureID> texture_id_stack; // [Internal]
|
||||
ImDrawVert* vtx_write; // [Internal] point within vtx_buffer after each add command (to avoid using the ImVector<> operators too much)
|
||||
|
||||
ImDrawList() { Clear(); }
|
||||
IMGUI_API void Clear();
|
||||
IMGUI_API void PushClipRect(const ImVec4& clip_rect);
|
||||
IMGUI_API void PushClipRect(const ImVec4& clip_rect); // Scissoring. The values are x1, y1, x2, y2.
|
||||
IMGUI_API void PopClipRect();
|
||||
IMGUI_API void PushTextureID(const ImTextureID& texture_id);
|
||||
IMGUI_API void PopTextureID();
|
||||
|
||||
// Primitives
|
||||
// Primitives
|
||||
IMGUI_API void AddLine(const ImVec2& a, const ImVec2& b, ImU32 col);
|
||||
IMGUI_API void AddRect(const ImVec2& a, const ImVec2& b, ImU32 col, float rounding = 0.0f, int rounding_corners=0x0F);
|
||||
IMGUI_API void AddRectFilled(const ImVec2& a, const ImVec2& b, ImU32 col, float rounding = 0.0f, int rounding_corners=0x0F);
|
||||
@ -787,7 +820,7 @@ struct ImDrawList
|
||||
IMGUI_API void AddCircle(const ImVec2& centre, float radius, ImU32 col, int num_segments = 12);
|
||||
IMGUI_API void AddCircleFilled(const ImVec2& centre, float radius, ImU32 col, int num_segments = 12);
|
||||
IMGUI_API void AddArc(const ImVec2& center, float rad, ImU32 col, int a_min, int a_max, bool tris = false, const ImVec2& third_point_offset = ImVec2(0,0));
|
||||
IMGUI_API void AddText(ImFont* font, float font_size, const ImVec2& pos, ImU32 col, const char* text_begin, const char* text_end = NULL, float wrap_width = 0.0f);
|
||||
IMGUI_API void AddText(ImFont* font, float font_size, const ImVec2& pos, ImU32 col, const char* text_begin, const char* text_end = NULL, float wrap_width = 0.0f, const ImVec2* cpu_clip_max = NULL);
|
||||
IMGUI_API void AddImage(ImTextureID user_texture_id, const ImVec2& a, const ImVec2& b, const ImVec2& uv0, const ImVec2& uv1, ImU32 col = 0xFFFFFFFF);
|
||||
|
||||
// Advanced
|
||||
@ -892,7 +925,7 @@ struct ImFont
|
||||
// 'wrap_width' enable automatic word-wrapping across multiple lines to fit into given width. 0.0f to disable.
|
||||
IMGUI_API ImVec2 CalcTextSizeA(float size, float max_width, float wrap_width, const char* text_begin, const char* text_end = NULL, const char** remaining = NULL) const; // utf8
|
||||
IMGUI_API ImVec2 CalcTextSizeW(float size, float max_width, const ImWchar* text_begin, const ImWchar* text_end, const ImWchar** remaining = NULL) const; // wchar
|
||||
IMGUI_API void RenderText(float size, ImVec2 pos, ImU32 col, const ImVec4& clip_rect, const char* text_begin, const char* text_end, ImDrawVert*& out_vertices, float wrap_width = 0.0f) const;
|
||||
IMGUI_API void RenderText(float size, ImVec2 pos, ImU32 col, const ImVec4& clip_rect, const char* text_begin, const char* text_end, ImDrawVert*& out_vertices, float wrap_width = 0.0f, const ImVec2* cpu_clip_max = NULL) const;
|
||||
IMGUI_API const char* CalcWordWrapPositionA(float scale, const char* text, const char* text_end, float wrap_width) const;
|
||||
};
|
||||
|
||||
|
17
3rdparty/bgfx/README.md
vendored
17
3rdparty/bgfx/README.md
vendored
@ -52,11 +52,9 @@ Who is using it?
|
||||
http://airmech.com/ AirMech is a free-to-play futuristic action real-time
|
||||
strategy video game developed and published by Carbon Games.
|
||||
|
||||
http://theengine.co/ Loom Game Engine developed by The Engine Company. Loom
|
||||
http://loomsdk.com/ Loom Game Engine developed by The Engine Company. Loom
|
||||
is a powerful 2D game engine with live reloading of code and assets, a friendly
|
||||
scripting language, and an efficient command-line workflow. Here is video where
|
||||
they explain why they choose bgfx over alternatives:
|
||||
<a href="https://www.youtube.com/watch?feature=player_embedded&v=PHY_XHkMGIM&t=1m53s" target="_blank"><img src="https://img.youtube.com/vi/PHY_XHkMGIM/0.jpg" alt="Why did you choose bgfx?" width="240" height="180" border="10" /></a>
|
||||
scripting language, and an efficient command-line workflow.
|
||||
|
||||
https://github.com/dariomanesku/cmftStudio cmftStudio - cubemap filtering tool.
|
||||

|
||||
@ -71,6 +69,10 @@ very early development and primary focusing on Mac as primary target. This is
|
||||
how it currently looks.
|
||||
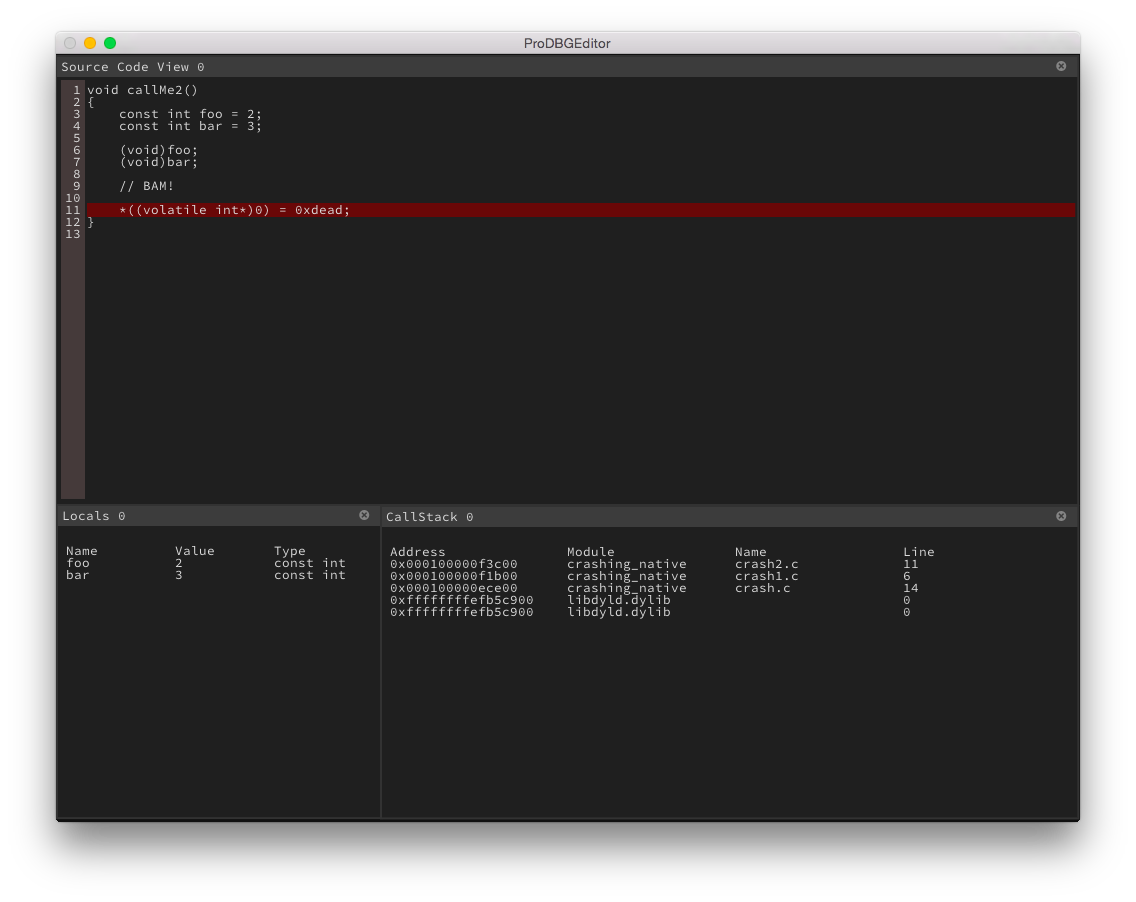
|
||||
|
||||
|
||||
http://www.dogbytegames.com/ Dogbyte Games is an indie mobile developer studio
|
||||
focusing on racing games.
|
||||
|
||||
Examples
|
||||
--------
|
||||
|
||||
@ -306,6 +308,9 @@ Dependencies
|
||||
Building
|
||||
--------
|
||||
|
||||
Steps bellow are for default build system inside bgfx repository. There is
|
||||
alterative way to build bgfx and examples with [fips](https://github.com/floooh/fips-bgfx/#fips-bgfx).
|
||||
|
||||
### Prerequisites
|
||||
|
||||
Windows users download GnuWin32 utilities from:
|
||||
@ -741,7 +746,9 @@ language API bindings, WinRT/WinPhone support.
|
||||
Kai Jourdan ([@questor](https://github.com/questor)) - 23-vectordisplay example
|
||||
Stanlo Slasinski ([@stanlo](https://github.com/stanlo)) - 24-nbody example
|
||||
Daniel Collin ([@emoon](https://github.com/emoon)) - Port of Ocornut's ImGui
|
||||
to bgfx.
|
||||
to bgfx.
|
||||
Andre Weissflog ([@floooh](https://github.com/floooh)) - Alternative build
|
||||
system fips.
|
||||
|
||||
[License (BSD 2-clause)](https://github.com/bkaradzic/bgfx/blob/master/LICENSE)
|
||||
-------------------------------------------------------------------------------
|
||||
|
8
3rdparty/bgfx/examples/08-update/update.cpp
vendored
8
3rdparty/bgfx/examples/08-update/update.cpp
vendored
@ -84,7 +84,7 @@ static const uint16_t s_cubeIndices[36] =
|
||||
9, 10, 11,
|
||||
|
||||
12, 14, 13, // 6
|
||||
14, 15, 13,
|
||||
14, 15, 13,
|
||||
|
||||
16, 18, 17, // 8
|
||||
18, 19, 17,
|
||||
@ -96,7 +96,7 @@ static const uint16_t s_cubeIndices[36] =
|
||||
static void updateTextureCubeRectBgra8(bgfx::TextureHandle _handle, uint8_t _side, uint32_t _x, uint32_t _y, uint32_t _width, uint32_t _height, uint8_t _r, uint8_t _g, uint8_t _b, uint8_t _a = 0xff)
|
||||
{
|
||||
bgfx::TextureInfo ti;
|
||||
bgfx::calcTextureSize(ti, _width, _height, 1, 1, bgfx::TextureFormat::BGRA8);
|
||||
bgfx::calcTextureSize(ti, _width, _height, 1, 1, false, bgfx::TextureFormat::BGRA8);
|
||||
|
||||
const bgfx::Memory* mem = bgfx::alloc(ti.storageSize);
|
||||
uint8_t* data = (uint8_t*)mem->data;
|
||||
@ -285,7 +285,7 @@ int _main_(int /*_argc*/, char** /*_argv*/)
|
||||
|
||||
float at[3] = { 0.0f, 0.0f, 0.0f };
|
||||
float eye[3] = { 0.0f, 0.0f, -5.0f };
|
||||
|
||||
|
||||
float view[16];
|
||||
float proj[16];
|
||||
bx::mtxLookAt(view, eye, at);
|
||||
@ -394,7 +394,7 @@ int _main_(int /*_argc*/, char** /*_argv*/)
|
||||
bgfx::submit(1);
|
||||
}
|
||||
|
||||
// Advance to next frame. Rendering thread will be kicked to
|
||||
// Advance to next frame. Rendering thread will be kicked to
|
||||
// process submitted rendering primitives.
|
||||
bgfx::frame();
|
||||
}
|
||||
|
10
3rdparty/bgfx/examples/18-ibl/ibl.cpp
vendored
10
3rdparty/bgfx/examples/18-ibl/ibl.cpp
vendored
@ -285,7 +285,7 @@ int _main_(int /*_argc*/, char** /*_argv*/)
|
||||
bool m_specularIbl;
|
||||
bool m_showDiffColorWheel;
|
||||
bool m_showSpecColorWheel;
|
||||
bool m_crossCubemapPreview;
|
||||
ImguiCubemap::Enum m_crossCubemapPreview;
|
||||
};
|
||||
|
||||
Settings settings;
|
||||
@ -305,7 +305,7 @@ int _main_(int /*_argc*/, char** /*_argv*/)
|
||||
settings.m_specularIbl = true;
|
||||
settings.m_showDiffColorWheel = true;
|
||||
settings.m_showSpecColorWheel = false;
|
||||
settings.m_crossCubemapPreview = false;
|
||||
settings.m_crossCubemapPreview = ImguiCubemap::Cross;
|
||||
|
||||
float time = 0.0f;
|
||||
|
||||
@ -324,7 +324,7 @@ int _main_(int /*_argc*/, char** /*_argv*/)
|
||||
);
|
||||
|
||||
static int32_t rightScrollArea = 0;
|
||||
imguiBeginScrollArea("Settings", width - 256 - 10, 10, 256, 590, &rightScrollArea);
|
||||
imguiBeginScrollArea("Settings", width - 256 - 10, 10, 256, 540, &rightScrollArea);
|
||||
|
||||
imguiLabel("Shade:");
|
||||
imguiSeparator();
|
||||
@ -350,9 +350,9 @@ int _main_(int /*_argc*/, char** /*_argv*/)
|
||||
, "Grace"
|
||||
) );
|
||||
static float lod = 0.0f;
|
||||
if (imguiCube(lightProbes[currentLightProbe].m_tex, lod, settings.m_crossCubemapPreview))
|
||||
if (imguiCube(lightProbes[currentLightProbe].m_tex, lod, settings.m_crossCubemapPreview, true) )
|
||||
{
|
||||
settings.m_crossCubemapPreview = !settings.m_crossCubemapPreview;
|
||||
settings.m_crossCubemapPreview = ImguiCubemap::Enum( (settings.m_crossCubemapPreview+1) % ImguiCubemap::Count);
|
||||
}
|
||||
imguiSlider("Texture LOD", lod, float(0.0f), 10.1f, 0.1f);
|
||||
|
||||
|
2
3rdparty/bgfx/examples/common/bgfx_utils.cpp
vendored
2
3rdparty/bgfx/examples/common/bgfx_utils.cpp
vendored
@ -5,6 +5,8 @@
|
||||
|
||||
#include <string.h> // strlen
|
||||
|
||||
#include "common.h"
|
||||
|
||||
#include <tinystl/allocator.h>
|
||||
#include <tinystl/vector.h>
|
||||
#include <tinystl/string.h>
|
||||
|
5
3rdparty/bgfx/examples/common/common.h
vendored
5
3rdparty/bgfx/examples/common/common.h
vendored
@ -3,7 +3,12 @@
|
||||
* License: http://www.opensource.org/licenses/BSD-2-Clause
|
||||
*/
|
||||
|
||||
#ifndef COMMON_H_HEADER_GUARD
|
||||
#define COMMON_H_HEADER_GUARD
|
||||
|
||||
#include <bx/timer.h>
|
||||
#include <bx/fpumath.h>
|
||||
|
||||
#include "entry/entry.h"
|
||||
|
||||
#endif // COMMON_H_HEADER_GUARD
|
||||
|
19
3rdparty/bgfx/examples/common/entry/cmd.cpp
vendored
19
3rdparty/bgfx/examples/common/entry/cmd.cpp
vendored
@ -7,11 +7,14 @@
|
||||
#include <stdint.h>
|
||||
#include <stdlib.h> // size_t
|
||||
#include <string.h> // strlen
|
||||
|
||||
#include <bx/allocator.h>
|
||||
#include <bx/hash.h>
|
||||
#include <bx/tokenizecmd.h>
|
||||
|
||||
#include "dbg.h"
|
||||
#include "cmd.h"
|
||||
#include "entry_p.h"
|
||||
|
||||
#include <tinystl/allocator.h>
|
||||
#include <tinystl/string.h>
|
||||
@ -89,14 +92,24 @@ struct CmdContext
|
||||
CmdLookup m_lookup;
|
||||
};
|
||||
|
||||
static CmdContext s_cmdContext;
|
||||
static CmdContext* s_cmdContext;
|
||||
|
||||
void cmdInit()
|
||||
{
|
||||
s_cmdContext = BX_NEW(entry::getAllocator(), CmdContext);
|
||||
}
|
||||
|
||||
void cmdShutdown()
|
||||
{
|
||||
BX_DELETE(entry::getAllocator(), s_cmdContext);
|
||||
}
|
||||
|
||||
void cmdAdd(const char* _name, ConsoleFn _fn, void* _userData)
|
||||
{
|
||||
s_cmdContext.add(_name, _fn, _userData);
|
||||
s_cmdContext->add(_name, _fn, _userData);
|
||||
}
|
||||
|
||||
void cmdExec(const char* _cmd)
|
||||
{
|
||||
s_cmdContext.exec(_cmd);
|
||||
s_cmdContext->exec(_cmd);
|
||||
}
|
||||
|
9
3rdparty/bgfx/examples/common/entry/cmd.h
vendored
9
3rdparty/bgfx/examples/common/entry/cmd.h
vendored
@ -9,7 +9,16 @@
|
||||
struct CmdContext;
|
||||
typedef int (*ConsoleFn)(CmdContext* _context, void* _userData, int _argc, char const* const* _argv);
|
||||
|
||||
///
|
||||
void cmdInit();
|
||||
|
||||
///
|
||||
void cmdShutdown();
|
||||
|
||||
///
|
||||
void cmdAdd(const char* _name, ConsoleFn _fn, void* _userData = NULL);
|
||||
|
||||
///
|
||||
void cmdExec(const char* _cmd);
|
||||
|
||||
#endif // CMD_H_HEADER_GUARD
|
||||
|
34
3rdparty/bgfx/examples/common/entry/entry.cpp
vendored
34
3rdparty/bgfx/examples/common/entry/entry.cpp
vendored
@ -22,7 +22,17 @@ namespace entry
|
||||
static bool s_exit = false;
|
||||
static bx::FileReaderI* s_fileReader = NULL;
|
||||
static bx::FileWriterI* s_fileWriter = NULL;
|
||||
static bx::CrtAllocator s_allocator;
|
||||
|
||||
extern bx::ReallocatorI* getDefaultAllocator();
|
||||
static bx::ReallocatorI* s_allocator = getDefaultAllocator();
|
||||
|
||||
#if ENTRY_CONFIG_IMPLEMENT_DEFAULT_ALLOCATOR
|
||||
bx::ReallocatorI* getDefaultAllocator()
|
||||
{
|
||||
static bx::CrtAllocator s_allocator;
|
||||
return &s_allocator;
|
||||
}
|
||||
#endif // ENTRY_CONFIG_IMPLEMENT_DEFAULT_ALLOCATOR
|
||||
|
||||
bool setOrToggle(uint32_t& _flags, const char* _name, uint32_t _bit, int _first, int _argc, char const* const* _argv)
|
||||
{
|
||||
@ -137,10 +147,12 @@ namespace entry
|
||||
s_fileWriter = new bx::CrtFileWriter;
|
||||
#endif // BX_CONFIG_CRT_FILE_READER_WRITER
|
||||
|
||||
cmdInit();
|
||||
cmdAdd("mouselock", cmdMouseLock);
|
||||
cmdAdd("graphics", cmdGraphics );
|
||||
cmdAdd("exit", cmdExit );
|
||||
|
||||
inputInit();
|
||||
inputAddBindings("bindings", s_bindings);
|
||||
|
||||
entry::WindowHandle defaultWindow = { 0 };
|
||||
@ -148,6 +160,11 @@ namespace entry
|
||||
|
||||
int32_t result = ::_main_(_argc, _argv);
|
||||
|
||||
inputRemoveBindings("bindings");
|
||||
inputShutdown();
|
||||
|
||||
cmdShutdown();
|
||||
|
||||
#if BX_CONFIG_CRT_FILE_READER_WRITER
|
||||
delete s_fileReader;
|
||||
s_fileReader = NULL;
|
||||
@ -448,7 +465,20 @@ namespace entry
|
||||
|
||||
bx::ReallocatorI* getAllocator()
|
||||
{
|
||||
return &s_allocator;
|
||||
return s_allocator;
|
||||
}
|
||||
|
||||
void* TinyStlAllocator::static_allocate(size_t _bytes)
|
||||
{
|
||||
return BX_ALLOC(getAllocator(), _bytes);
|
||||
}
|
||||
|
||||
void TinyStlAllocator::static_deallocate(void* _ptr, size_t /*_bytes*/)
|
||||
{
|
||||
if (NULL != _ptr)
|
||||
{
|
||||
BX_FREE(getAllocator(), _ptr);
|
||||
}
|
||||
}
|
||||
|
||||
} // namespace entry
|
||||
|
30
3rdparty/bgfx/examples/common/entry/entry_osx.mm
vendored
30
3rdparty/bgfx/examples/common/entry/entry_osx.mm
vendored
@ -57,21 +57,21 @@ namespace entry
|
||||
|
||||
static int32_t threadFunc(void* _userData)
|
||||
{
|
||||
CFBundleRef mainBundle = CFBundleGetMainBundle();
|
||||
if ( mainBundle != nil )
|
||||
{
|
||||
CFURLRef resourcesURL = CFBundleCopyResourcesDirectoryURL(mainBundle);
|
||||
if ( resourcesURL != nil )
|
||||
{
|
||||
char path[PATH_MAX];
|
||||
if (CFURLGetFileSystemRepresentation(resourcesURL, TRUE, (UInt8 *)path, PATH_MAX) )
|
||||
{
|
||||
chdir(path);
|
||||
}
|
||||
CFRelease(resourcesURL);
|
||||
}
|
||||
}
|
||||
|
||||
CFBundleRef mainBundle = CFBundleGetMainBundle();
|
||||
if ( mainBundle != nil )
|
||||
{
|
||||
CFURLRef resourcesURL = CFBundleCopyResourcesDirectoryURL(mainBundle);
|
||||
if ( resourcesURL != nil )
|
||||
{
|
||||
char path[PATH_MAX];
|
||||
if (CFURLGetFileSystemRepresentation(resourcesURL, TRUE, (UInt8 *)path, PATH_MAX) )
|
||||
{
|
||||
chdir(path);
|
||||
}
|
||||
CFRelease(resourcesURL);
|
||||
}
|
||||
}
|
||||
|
||||
MainThreadEntry* self = (MainThreadEntry*)_userData;
|
||||
return main(self->m_argc, self->m_argv);
|
||||
}
|
||||
|
12
3rdparty/bgfx/examples/common/entry/entry_p.h
vendored
12
3rdparty/bgfx/examples/common/entry/entry_p.h
vendored
@ -6,6 +6,8 @@
|
||||
#ifndef ENTRY_PRIVATE_H_HEADER_GUARD
|
||||
#define ENTRY_PRIVATE_H_HEADER_GUARD
|
||||
|
||||
#define TINYSTL_ALLOCATOR entry::TinyStlAllocator
|
||||
|
||||
#include <bx/spscqueue.h>
|
||||
|
||||
#include "entry.h"
|
||||
@ -37,11 +39,21 @@
|
||||
# error "Both ENTRY_DEFAULT_WIDTH and ENTRY_DEFAULT_HEIGHT must be defined."
|
||||
#endif // ENTRY_DEFAULT_WIDTH
|
||||
|
||||
#ifndef ENTRY_CONFIG_IMPLEMENT_DEFAULT_ALLOCATOR
|
||||
# define ENTRY_CONFIG_IMPLEMENT_DEFAULT_ALLOCATOR 1
|
||||
#endif // ENTRY_CONFIG_IMPLEMENT_DEFAULT_ALLOCATOR
|
||||
|
||||
#define ENTRY_IMPLEMENT_EVENT(_class, _type) \
|
||||
_class(WindowHandle _handle) : Event(_type, _handle) {}
|
||||
|
||||
namespace entry
|
||||
{
|
||||
struct TinyStlAllocator
|
||||
{
|
||||
static void* static_allocate(size_t _bytes);
|
||||
static void static_deallocate(void* _ptr, size_t /*_bytes*/);
|
||||
};
|
||||
|
||||
int main(int _argc, char** _argv);
|
||||
|
||||
struct Event
|
||||
|
@ -10,7 +10,8 @@
|
||||
#define XK_MISCELLANY
|
||||
#define XK_LATIN1
|
||||
#include <X11/keysymdef.h>
|
||||
#include <bgfxplatform.h> // will include X11 which #defines None... Don't mess with order of includes.
|
||||
#include <X11/Xlib.h> // will include X11 which #defines None... Don't mess with order of includes.
|
||||
#include <bgfxplatform.h>
|
||||
|
||||
#undef None
|
||||
#include <bx/thread.h>
|
||||
|
61
3rdparty/bgfx/examples/common/entry/input.cpp
vendored
61
3rdparty/bgfx/examples/common/entry/input.cpp
vendored
@ -8,6 +8,7 @@
|
||||
#include "entry_p.h"
|
||||
#include "input.h"
|
||||
|
||||
#include <bx/allocator.h>
|
||||
#include <bx/ringbuffer.h>
|
||||
#include <tinystl/allocator.h>
|
||||
#include <tinystl/unordered_map.h>
|
||||
@ -249,95 +250,105 @@ struct Input
|
||||
Gamepad m_gamepad[ENTRY_CONFIG_MAX_GAMEPADS];
|
||||
};
|
||||
|
||||
static Input s_input;
|
||||
static Input* s_input;
|
||||
|
||||
void inputInit()
|
||||
{
|
||||
s_input = BX_NEW(entry::getAllocator(), Input);
|
||||
}
|
||||
|
||||
void inputShutdown()
|
||||
{
|
||||
BX_DELETE(entry::getAllocator(), s_input);
|
||||
}
|
||||
|
||||
void inputAddBindings(const char* _name, const InputBinding* _bindings)
|
||||
{
|
||||
s_input.addBindings(_name, _bindings);
|
||||
s_input->addBindings(_name, _bindings);
|
||||
}
|
||||
|
||||
void inputRemoveBindings(const char* _name)
|
||||
{
|
||||
s_input.removeBindings(_name);
|
||||
s_input->removeBindings(_name);
|
||||
}
|
||||
|
||||
void inputProcess()
|
||||
{
|
||||
s_input.process();
|
||||
s_input->process();
|
||||
}
|
||||
|
||||
void inputSetMouseResolution(uint16_t _width, uint16_t _height)
|
||||
{
|
||||
s_input.m_mouse.setResolution(_width, _height);
|
||||
s_input->m_mouse.setResolution(_width, _height);
|
||||
}
|
||||
|
||||
void inputSetKeyState(entry::Key::Enum _key, uint8_t _modifiers, bool _down)
|
||||
{
|
||||
s_input.m_keyboard.setKeyState(_key, _modifiers, _down);
|
||||
s_input->m_keyboard.setKeyState(_key, _modifiers, _down);
|
||||
}
|
||||
|
||||
void inputChar(uint8_t _len, const uint8_t _char[4])
|
||||
{
|
||||
s_input.m_keyboard.pushChar(_len, _char);
|
||||
s_input->m_keyboard.pushChar(_len, _char);
|
||||
}
|
||||
|
||||
const uint8_t* inputGetChar()
|
||||
{
|
||||
return s_input.m_keyboard.popChar();
|
||||
return s_input->m_keyboard.popChar();
|
||||
}
|
||||
|
||||
void inputCharFlush()
|
||||
{
|
||||
s_input.m_keyboard.charFlush();
|
||||
s_input->m_keyboard.charFlush();
|
||||
}
|
||||
|
||||
void inputSetMousePos(int32_t _mx, int32_t _my, int32_t _mz)
|
||||
{
|
||||
s_input.m_mouse.setPos(_mx, _my, _mz);
|
||||
s_input->m_mouse.setPos(_mx, _my, _mz);
|
||||
}
|
||||
|
||||
void inputSetMouseButtonState(entry::MouseButton::Enum _button, uint8_t _state)
|
||||
{
|
||||
s_input.m_mouse.setButtonState(_button, _state);
|
||||
s_input->m_mouse.setButtonState(_button, _state);
|
||||
}
|
||||
|
||||
void inputGetMouse(float _mouse[3])
|
||||
{
|
||||
_mouse[0] = s_input.m_mouse.m_norm[0];
|
||||
_mouse[1] = s_input.m_mouse.m_norm[1];
|
||||
_mouse[2] = s_input.m_mouse.m_norm[2];
|
||||
s_input.m_mouse.m_norm[0] = 0.0f;
|
||||
s_input.m_mouse.m_norm[1] = 0.0f;
|
||||
s_input.m_mouse.m_norm[2] = 0.0f;
|
||||
_mouse[0] = s_input->m_mouse.m_norm[0];
|
||||
_mouse[1] = s_input->m_mouse.m_norm[1];
|
||||
_mouse[2] = s_input->m_mouse.m_norm[2];
|
||||
s_input->m_mouse.m_norm[0] = 0.0f;
|
||||
s_input->m_mouse.m_norm[1] = 0.0f;
|
||||
s_input->m_mouse.m_norm[2] = 0.0f;
|
||||
}
|
||||
|
||||
bool inputIsMouseLocked()
|
||||
{
|
||||
return s_input.m_mouse.m_lock;
|
||||
return s_input->m_mouse.m_lock;
|
||||
}
|
||||
|
||||
void inputSetMouseLock(bool _lock)
|
||||
{
|
||||
if (s_input.m_mouse.m_lock != _lock)
|
||||
if (s_input->m_mouse.m_lock != _lock)
|
||||
{
|
||||
s_input.m_mouse.m_lock = _lock;
|
||||
s_input->m_mouse.m_lock = _lock;
|
||||
entry::WindowHandle defaultWindow = { 0 };
|
||||
entry::setMouseLock(defaultWindow, _lock);
|
||||
if (_lock)
|
||||
{
|
||||
s_input.m_mouse.m_norm[0] = 0.0f;
|
||||
s_input.m_mouse.m_norm[1] = 0.0f;
|
||||
s_input.m_mouse.m_norm[2] = 0.0f;
|
||||
s_input->m_mouse.m_norm[0] = 0.0f;
|
||||
s_input->m_mouse.m_norm[1] = 0.0f;
|
||||
s_input->m_mouse.m_norm[2] = 0.0f;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
void inputSetGamepadAxis(entry::GamepadHandle _handle, entry::GamepadAxis::Enum _axis, int32_t _value)
|
||||
{
|
||||
s_input.m_gamepad[_handle.idx].setAxis(_axis, _value);
|
||||
s_input->m_gamepad[_handle.idx].setAxis(_axis, _value);
|
||||
}
|
||||
|
||||
int32_t inputGetGamepadAxis(entry::GamepadHandle _handle, entry::GamepadAxis::Enum _axis)
|
||||
{
|
||||
return s_input.m_gamepad[_handle.idx].getAxis(_axis);
|
||||
return s_input->m_gamepad[_handle.idx].getAxis(_axis);
|
||||
}
|
||||
|
6
3rdparty/bgfx/examples/common/entry/input.h
vendored
6
3rdparty/bgfx/examples/common/entry/input.h
vendored
@ -21,6 +21,12 @@ struct InputBinding
|
||||
|
||||
#define INPUT_BINDING_END { entry::Key::None, entry::Modifier::None, 0, NULL, NULL }
|
||||
|
||||
///
|
||||
void inputInit();
|
||||
|
||||
///
|
||||
void inputShutdown();
|
||||
|
||||
///
|
||||
void inputAddBindings(const char* _name, const InputBinding* _bindings);
|
||||
|
||||
|
123
3rdparty/bgfx/examples/common/imgui/fs_imgui_latlong.bin.h
vendored
Normal file
123
3rdparty/bgfx/examples/common/imgui/fs_imgui_latlong.bin.h
vendored
Normal file
@ -0,0 +1,123 @@
|
||||
static const uint8_t fs_imgui_latlong_glsl[646] =
|
||||
{
|
||||
0x46, 0x53, 0x48, 0x03, 0x6f, 0x1e, 0x3e, 0x3c, 0x02, 0x00, 0x11, 0x75, 0x5f, 0x69, 0x6d, 0x61, // FSH.o.><...u_ima
|
||||
0x67, 0x65, 0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x07, 0x01, 0x00, 0x00, // geLodEnabled....
|
||||
0x01, 0x00, 0x0a, 0x73, 0x5f, 0x74, 0x65, 0x78, 0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x00, 0x01, 0x00, // ...s_texColor...
|
||||
0x00, 0x01, 0x00, 0x4e, 0x02, 0x00, 0x00, 0x76, 0x61, 0x72, 0x79, 0x69, 0x6e, 0x67, 0x20, 0x68, // ...N...varying h
|
||||
0x69, 0x67, 0x68, 0x70, 0x20, 0x76, 0x65, 0x63, 0x32, 0x20, 0x76, 0x5f, 0x74, 0x65, 0x78, 0x63, // ighp vec2 v_texc
|
||||
0x6f, 0x6f, 0x72, 0x64, 0x30, 0x3b, 0x0a, 0x75, 0x6e, 0x69, 0x66, 0x6f, 0x72, 0x6d, 0x20, 0x68, // oord0;.uniform h
|
||||
0x69, 0x67, 0x68, 0x70, 0x20, 0x76, 0x65, 0x63, 0x34, 0x20, 0x75, 0x5f, 0x69, 0x6d, 0x61, 0x67, // ighp vec4 u_imag
|
||||
0x65, 0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x3b, 0x0a, 0x75, 0x6e, 0x69, // eLodEnabled;.uni
|
||||
0x66, 0x6f, 0x72, 0x6d, 0x20, 0x6c, 0x6f, 0x77, 0x70, 0x20, 0x73, 0x61, 0x6d, 0x70, 0x6c, 0x65, // form lowp sample
|
||||
0x72, 0x43, 0x75, 0x62, 0x65, 0x20, 0x73, 0x5f, 0x74, 0x65, 0x78, 0x43, 0x6f, 0x6c, 0x6f, 0x72, // rCube s_texColor
|
||||
0x3b, 0x0a, 0x76, 0x6f, 0x69, 0x64, 0x20, 0x6d, 0x61, 0x69, 0x6e, 0x20, 0x28, 0x29, 0x0a, 0x7b, // ;.void main ().{
|
||||
0x0a, 0x20, 0x20, 0x68, 0x69, 0x67, 0x68, 0x70, 0x20, 0x76, 0x65, 0x63, 0x33, 0x20, 0x72, 0x65, // . highp vec3 re
|
||||
0x73, 0x75, 0x6c, 0x74, 0x5f, 0x31, 0x3b, 0x0a, 0x20, 0x20, 0x68, 0x69, 0x67, 0x68, 0x70, 0x20, // sult_1;. highp
|
||||
0x66, 0x6c, 0x6f, 0x61, 0x74, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x32, 0x3b, 0x0a, // float tmpvar_2;.
|
||||
0x20, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x32, 0x20, 0x3d, 0x20, 0x28, 0x76, 0x5f, // tmpvar_2 = (v_
|
||||
0x74, 0x65, 0x78, 0x63, 0x6f, 0x6f, 0x72, 0x64, 0x30, 0x2e, 0x78, 0x20, 0x2a, 0x20, 0x36, 0x2e, // texcoord0.x * 6.
|
||||
0x32, 0x38, 0x33, 0x31, 0x39, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x68, 0x69, 0x67, 0x68, 0x70, 0x20, // 28319);. highp
|
||||
0x66, 0x6c, 0x6f, 0x61, 0x74, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x33, 0x3b, 0x0a, // float tmpvar_3;.
|
||||
0x20, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x33, 0x20, 0x3d, 0x20, 0x28, 0x76, 0x5f, // tmpvar_3 = (v_
|
||||
0x74, 0x65, 0x78, 0x63, 0x6f, 0x6f, 0x72, 0x64, 0x30, 0x2e, 0x79, 0x20, 0x2a, 0x20, 0x33, 0x2e, // texcoord0.y * 3.
|
||||
0x31, 0x34, 0x31, 0x35, 0x39, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, // 14159);. result
|
||||
0x5f, 0x31, 0x2e, 0x78, 0x20, 0x3d, 0x20, 0x28, 0x73, 0x69, 0x6e, 0x28, 0x74, 0x6d, 0x70, 0x76, // _1.x = (sin(tmpv
|
||||
0x61, 0x72, 0x5f, 0x33, 0x29, 0x20, 0x2a, 0x20, 0x63, 0x6f, 0x73, 0x28, 0x74, 0x6d, 0x70, 0x76, // ar_3) * cos(tmpv
|
||||
0x61, 0x72, 0x5f, 0x32, 0x29, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, // ar_2));. result
|
||||
0x5f, 0x31, 0x2e, 0x79, 0x20, 0x3d, 0x20, 0x63, 0x6f, 0x73, 0x28, 0x74, 0x6d, 0x70, 0x76, 0x61, // _1.y = cos(tmpva
|
||||
0x72, 0x5f, 0x33, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x72, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x5f, 0x31, // r_3);. result_1
|
||||
0x2e, 0x7a, 0x20, 0x3d, 0x20, 0x28, 0x2d, 0x28, 0x73, 0x69, 0x6e, 0x28, 0x74, 0x6d, 0x70, 0x76, // .z = (-(sin(tmpv
|
||||
0x61, 0x72, 0x5f, 0x33, 0x29, 0x29, 0x20, 0x2a, 0x20, 0x73, 0x69, 0x6e, 0x28, 0x74, 0x6d, 0x70, // ar_3)) * sin(tmp
|
||||
0x76, 0x61, 0x72, 0x5f, 0x32, 0x29, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x6c, 0x6f, 0x77, 0x70, 0x20, // var_2));. lowp
|
||||
0x76, 0x65, 0x63, 0x34, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x34, 0x3b, 0x0a, 0x20, // vec4 tmpvar_4;.
|
||||
0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x34, 0x2e, 0x78, 0x79, 0x7a, 0x20, 0x3d, 0x20, // tmpvar_4.xyz =
|
||||
0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x43, 0x75, 0x62, 0x65, 0x4c, 0x6f, 0x64, 0x20, 0x20, // textureCubeLod
|
||||
0x20, 0x20, 0x28, 0x73, 0x5f, 0x74, 0x65, 0x78, 0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x2c, 0x20, 0x72, // (s_texColor, r
|
||||
0x65, 0x73, 0x75, 0x6c, 0x74, 0x5f, 0x31, 0x2c, 0x20, 0x75, 0x5f, 0x69, 0x6d, 0x61, 0x67, 0x65, // esult_1, u_image
|
||||
0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x2e, 0x78, 0x29, 0x2e, 0x78, 0x79, // LodEnabled.x).xy
|
||||
0x7a, 0x3b, 0x0a, 0x20, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x34, 0x2e, 0x77, 0x20, // z;. tmpvar_4.w
|
||||
0x3d, 0x20, 0x28, 0x30, 0x2e, 0x32, 0x20, 0x2b, 0x20, 0x28, 0x30, 0x2e, 0x38, 0x20, 0x2a, 0x20, // = (0.2 + (0.8 *
|
||||
0x75, 0x5f, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, // u_imageLodEnable
|
||||
0x64, 0x2e, 0x79, 0x29, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x67, 0x6c, 0x5f, 0x46, 0x72, 0x61, 0x67, // d.y));. gl_Frag
|
||||
0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x3d, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x34, // Color = tmpvar_4
|
||||
0x3b, 0x0a, 0x7d, 0x0a, 0x0a, 0x00, // ;.}...
|
||||
};
|
||||
static const uint8_t fs_imgui_latlong_dx9[553] =
|
||||
{
|
||||
0x46, 0x53, 0x48, 0x03, 0x6f, 0x1e, 0x3e, 0x3c, 0x01, 0x00, 0x11, 0x75, 0x5f, 0x69, 0x6d, 0x61, // FSH.o.><...u_ima
|
||||
0x67, 0x65, 0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x15, 0x01, 0x00, 0x00, // geLodEnabled....
|
||||
0x01, 0x00, 0x04, 0x02, 0x00, 0x03, 0xff, 0xff, 0xfe, 0xff, 0x30, 0x00, 0x43, 0x54, 0x41, 0x42, // ..........0.CTAB
|
||||
0x1c, 0x00, 0x00, 0x00, 0x8b, 0x00, 0x00, 0x00, 0x00, 0x03, 0xff, 0xff, 0x02, 0x00, 0x00, 0x00, // ................
|
||||
0x1c, 0x00, 0x00, 0x00, 0x00, 0x81, 0x00, 0x00, 0x84, 0x00, 0x00, 0x00, 0x44, 0x00, 0x00, 0x00, // ............D...
|
||||
0x03, 0x00, 0x00, 0x00, 0x01, 0x00, 0x02, 0x00, 0x50, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // ........P.......
|
||||
0x60, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x74, 0x00, 0x00, 0x00, // `...........t...
|
||||
0x00, 0x00, 0x00, 0x00, 0x73, 0x5f, 0x74, 0x65, 0x78, 0x43, 0x6f, 0x6c, 0x6f, 0x72, 0x00, 0xab, // ....s_texColor..
|
||||
0x04, 0x00, 0x0e, 0x00, 0x01, 0x00, 0x01, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // ................
|
||||
0x75, 0x5f, 0x69, 0x6d, 0x61, 0x67, 0x65, 0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, // u_imageLodEnable
|
||||
0x64, 0x00, 0xab, 0xab, 0x01, 0x00, 0x03, 0x00, 0x01, 0x00, 0x04, 0x00, 0x01, 0x00, 0x00, 0x00, // d...............
|
||||
0x00, 0x00, 0x00, 0x00, 0x70, 0x73, 0x5f, 0x33, 0x5f, 0x30, 0x00, 0x4d, 0x69, 0x63, 0x72, 0x6f, // ....ps_3_0.Micro
|
||||
0x73, 0x6f, 0x66, 0x74, 0x20, 0x28, 0x52, 0x29, 0x20, 0x48, 0x4c, 0x53, 0x4c, 0x20, 0x53, 0x68, // soft (R) HLSL Sh
|
||||
0x61, 0x64, 0x65, 0x72, 0x20, 0x43, 0x6f, 0x6d, 0x70, 0x69, 0x6c, 0x65, 0x72, 0x20, 0x39, 0x2e, // ader Compiler 9.
|
||||
0x32, 0x39, 0x2e, 0x39, 0x35, 0x32, 0x2e, 0x33, 0x31, 0x31, 0x31, 0x00, 0x51, 0x00, 0x00, 0x05, // 29.952.3111.Q...
|
||||
0x01, 0x00, 0x0f, 0xa0, 0x00, 0x00, 0x00, 0x3f, 0xdb, 0x0f, 0xc9, 0x40, 0xdb, 0x0f, 0x49, 0xc0, // .......?...@..I.
|
||||
0x00, 0x00, 0x00, 0x00, 0x51, 0x00, 0x00, 0x05, 0x02, 0x00, 0x0f, 0xa0, 0xcd, 0xcc, 0x4c, 0x3f, // ....Q.........L?
|
||||
0xcd, 0xcc, 0x4c, 0x3e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x02, // ..L>............
|
||||
0x05, 0x00, 0x00, 0x80, 0x00, 0x00, 0x03, 0x90, 0x1f, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x98, // ................
|
||||
0x00, 0x08, 0x0f, 0xa0, 0x02, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x01, 0x00, 0x00, 0xa0, // ................
|
||||
0x00, 0x00, 0x00, 0x90, 0x13, 0x00, 0x00, 0x02, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x80, // ................
|
||||
0x04, 0x00, 0x00, 0x04, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x80, 0x01, 0x00, 0x55, 0xa0, // ..............U.
|
||||
0x01, 0x00, 0xaa, 0xa0, 0x25, 0x00, 0x00, 0x02, 0x01, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00, 0x80, // ....%...........
|
||||
0x04, 0x00, 0x00, 0x04, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x55, 0x90, 0x01, 0x00, 0x00, 0xa0, // ..........U.....
|
||||
0x01, 0x00, 0x00, 0xa0, 0x13, 0x00, 0x00, 0x02, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x80, // ................
|
||||
0x04, 0x00, 0x00, 0x04, 0x00, 0x00, 0x01, 0x80, 0x00, 0x00, 0x00, 0x80, 0x01, 0x00, 0x55, 0xa0, // ..............U.
|
||||
0x01, 0x00, 0xaa, 0xa0, 0x25, 0x00, 0x00, 0x02, 0x02, 0x00, 0x03, 0x80, 0x00, 0x00, 0x00, 0x80, // ....%...........
|
||||
0x05, 0x00, 0x00, 0x03, 0x00, 0x00, 0x01, 0x80, 0x01, 0x00, 0x00, 0x80, 0x02, 0x00, 0x55, 0x80, // ..............U.
|
||||
0x05, 0x00, 0x00, 0x03, 0x00, 0x00, 0x04, 0x80, 0x01, 0x00, 0x55, 0x80, 0x02, 0x00, 0x55, 0x81, // ..........U...U.
|
||||
0x01, 0x00, 0x00, 0x02, 0x00, 0x00, 0x02, 0x80, 0x02, 0x00, 0x00, 0x80, 0x01, 0x00, 0x00, 0x02, // ................
|
||||
0x00, 0x00, 0x08, 0x80, 0x00, 0x00, 0x00, 0xa0, 0x5f, 0x00, 0x00, 0x03, 0x00, 0x00, 0x0f, 0x80, // ........_.......
|
||||
0x00, 0x00, 0xe4, 0x80, 0x00, 0x08, 0xe4, 0xa0, 0x01, 0x00, 0x00, 0x02, 0x00, 0x08, 0x07, 0x80, // ................
|
||||
0x00, 0x00, 0xe4, 0x80, 0x01, 0x00, 0x00, 0x02, 0x00, 0x00, 0x02, 0x80, 0x00, 0x00, 0x55, 0xa0, // ..............U.
|
||||
0x04, 0x00, 0x00, 0x04, 0x00, 0x08, 0x08, 0x80, 0x00, 0x00, 0x55, 0x80, 0x02, 0x00, 0x00, 0xa0, // ..........U.....
|
||||
0x02, 0x00, 0x55, 0xa0, 0xff, 0xff, 0x00, 0x00, 0x00, // ..U......
|
||||
};
|
||||
static const uint8_t fs_imgui_latlong_dx11[600] =
|
||||
{
|
||||
0x46, 0x53, 0x48, 0x03, 0x6f, 0x1e, 0x3e, 0x3c, 0x01, 0x00, 0x11, 0x75, 0x5f, 0x69, 0x6d, 0x61, // FSH.o.><...u_ima
|
||||
0x67, 0x65, 0x4c, 0x6f, 0x64, 0x45, 0x6e, 0x61, 0x62, 0x6c, 0x65, 0x64, 0x15, 0x00, 0x30, 0x0a, // geLodEnabled..0.
|
||||
0x01, 0x00, 0x30, 0x02, 0x44, 0x58, 0x42, 0x43, 0x89, 0x11, 0x25, 0xa6, 0xf5, 0x66, 0x12, 0x3f, // ..0.DXBC..%..f.?
|
||||
0xc0, 0x1f, 0x67, 0x9b, 0x6e, 0x4e, 0xac, 0x03, 0x01, 0x00, 0x00, 0x00, 0x30, 0x02, 0x00, 0x00, // ..g.nN......0...
|
||||
0x03, 0x00, 0x00, 0x00, 0x2c, 0x00, 0x00, 0x00, 0x84, 0x00, 0x00, 0x00, 0xb8, 0x00, 0x00, 0x00, // ....,...........
|
||||
0x49, 0x53, 0x47, 0x4e, 0x50, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, // ISGNP...........
|
||||
0x38, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, // 8...............
|
||||
0x00, 0x00, 0x00, 0x00, 0x0f, 0x00, 0x00, 0x00, 0x44, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // ........D.......
|
||||
0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x03, 0x03, 0x00, 0x00, // ................
|
||||
0x53, 0x56, 0x5f, 0x50, 0x4f, 0x53, 0x49, 0x54, 0x49, 0x4f, 0x4e, 0x00, 0x54, 0x45, 0x58, 0x43, // SV_POSITION.TEXC
|
||||
0x4f, 0x4f, 0x52, 0x44, 0x00, 0xab, 0xab, 0xab, 0x4f, 0x53, 0x47, 0x4e, 0x2c, 0x00, 0x00, 0x00, // OORD....OSGN,...
|
||||
0x01, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // ........ .......
|
||||
0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0x00, 0x00, 0x00, // ................
|
||||
0x53, 0x56, 0x5f, 0x54, 0x41, 0x52, 0x47, 0x45, 0x54, 0x00, 0xab, 0xab, 0x53, 0x48, 0x44, 0x52, // SV_TARGET...SHDR
|
||||
0x70, 0x01, 0x00, 0x00, 0x40, 0x00, 0x00, 0x00, 0x5c, 0x00, 0x00, 0x00, 0x59, 0x00, 0x00, 0x04, // p...@.......Y...
|
||||
0x46, 0x8e, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0xa4, 0x00, 0x00, 0x00, 0x5a, 0x00, 0x00, 0x03, // F. .........Z...
|
||||
0x00, 0x60, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x58, 0x30, 0x00, 0x04, 0x00, 0x70, 0x10, 0x00, // .`......X0...p..
|
||||
0x00, 0x00, 0x00, 0x00, 0x55, 0x55, 0x00, 0x00, 0x62, 0x10, 0x00, 0x03, 0x32, 0x10, 0x10, 0x00, // ....UU..b...2...
|
||||
0x01, 0x00, 0x00, 0x00, 0x65, 0x00, 0x00, 0x03, 0xf2, 0x20, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, // ....e.... ......
|
||||
0x68, 0x00, 0x00, 0x02, 0x03, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00, 0x0a, 0x32, 0x00, 0x10, 0x00, // h.......8...2...
|
||||
0x00, 0x00, 0x00, 0x00, 0x46, 0x10, 0x10, 0x00, 0x01, 0x00, 0x00, 0x00, 0x02, 0x40, 0x00, 0x00, // ....F........@..
|
||||
0xdb, 0x0f, 0xc9, 0x40, 0xdb, 0x0f, 0x49, 0x40, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // ...@..I@........
|
||||
0x4d, 0x00, 0x00, 0x07, 0x42, 0x00, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xd0, 0x00, 0x00, // M...B...........
|
||||
0x1a, 0x00, 0x10, 0x80, 0x41, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x4d, 0x00, 0x00, 0x06, // ....A.......M...
|
||||
0x32, 0x00, 0x10, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0xd0, 0x00, 0x00, 0x16, 0x05, 0x10, 0x00, // 2...............
|
||||
0x00, 0x00, 0x00, 0x00, 0x4d, 0x00, 0x00, 0x06, 0x00, 0xd0, 0x00, 0x00, 0x62, 0x00, 0x10, 0x00, // ....M.......b...
|
||||
0x02, 0x00, 0x00, 0x00, 0x06, 0x01, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00, 0x07, // ............8...
|
||||
0x82, 0x00, 0x10, 0x00, 0x02, 0x00, 0x00, 0x00, 0x2a, 0x00, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, // ........*.......
|
||||
0x1a, 0x00, 0x10, 0x00, 0x01, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00, 0x07, 0x12, 0x00, 0x10, 0x00, // ........8.......
|
||||
0x02, 0x00, 0x00, 0x00, 0x0a, 0x00, 0x10, 0x00, 0x01, 0x00, 0x00, 0x00, 0x1a, 0x00, 0x10, 0x00, // ................
|
||||
0x02, 0x00, 0x00, 0x00, 0x48, 0x00, 0x00, 0x0c, 0xf2, 0x00, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, // ....H...........
|
||||
0x86, 0x03, 0x10, 0x00, 0x02, 0x00, 0x00, 0x00, 0x46, 0x7e, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, // ........F~......
|
||||
0x00, 0x60, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0a, 0x80, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, // .`........ .....
|
||||
0xa3, 0x00, 0x00, 0x00, 0x36, 0x00, 0x00, 0x05, 0x72, 0x20, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, // ....6...r ......
|
||||
0x46, 0x02, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x32, 0x00, 0x00, 0x0a, 0x82, 0x20, 0x10, 0x00, // F.......2.... ..
|
||||
0x00, 0x00, 0x00, 0x00, 0x1a, 0x80, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0xa3, 0x00, 0x00, 0x00, // ...... .........
|
||||
0x01, 0x40, 0x00, 0x00, 0xcd, 0xcc, 0x4c, 0x3f, 0x01, 0x40, 0x00, 0x00, 0xcd, 0xcc, 0x4c, 0x3e, // .@....L?.@....L>
|
||||
0x3e, 0x00, 0x00, 0x01, 0x00, 0x00, 0x40, 0x0a, // >.....@.
|
||||
};
|
38
3rdparty/bgfx/examples/common/imgui/fs_imgui_latlong.sc
vendored
Normal file
38
3rdparty/bgfx/examples/common/imgui/fs_imgui_latlong.sc
vendored
Normal file
@ -0,0 +1,38 @@
|
||||
$input v_texcoord0
|
||||
|
||||
/*
|
||||
* Copyright 2014-2015 Dario Manesku. All rights reserved.
|
||||
* License: http://www.opensource.org/licenses/BSD-2-Clause
|
||||
*/
|
||||
|
||||
#include <bgfx_shader.sh>
|
||||
|
||||
uniform vec4 u_imageLodEnabled;
|
||||
SAMPLERCUBE(s_texColor, 0);
|
||||
|
||||
#define u_imageLod u_imageLodEnabled.x
|
||||
#define u_imageEnabled u_imageLodEnabled.y
|
||||
|
||||
vec3 vecFromLatLong(vec2 _uv)
|
||||
{
|
||||
float pi = 3.14159265;
|
||||
float twoPi = 2.0*pi;
|
||||
float phi = _uv.x * twoPi;
|
||||
float theta = _uv.y *pi;
|
||||
|
||||
vec3 result;
|
||||
result.x = sin(theta)*cos(phi);
|
||||
result.y = cos(theta);
|
||||
result.z = -sin(theta)*sin(phi);
|
||||
|
||||
return result;
|
||||
}
|
||||
|
||||
void main()
|
||||
{
|
||||
vec3 dir = vecFromLatLong(v_texcoord0);
|
||||
vec3 color = textureCubeLod(s_texColor, dir, u_imageLod).xyz;
|
||||
float alpha = 0.2 + 0.8*u_imageEnabled;
|
||||
|
||||
gl_FragColor = vec4(color, alpha);
|
||||
}
|
260
3rdparty/bgfx/examples/common/imgui/imgui.cpp
vendored
260
3rdparty/bgfx/examples/common/imgui/imgui.cpp
vendored
@ -41,6 +41,8 @@
|
||||
#include "fs_imgui_texture.bin.h"
|
||||
#include "vs_imgui_cubemap.bin.h"
|
||||
#include "fs_imgui_cubemap.bin.h"
|
||||
#include "vs_imgui_latlong.bin.h"
|
||||
#include "fs_imgui_latlong.bin.h"
|
||||
#include "vs_imgui_image.bin.h"
|
||||
#include "fs_imgui_image.bin.h"
|
||||
#include "fs_imgui_image_swizz.bin.h"
|
||||
@ -65,15 +67,25 @@ static const int32_t SCROLL_AREA_PADDING = 6;
|
||||
static const int32_t AREA_HEADER = 20;
|
||||
static const float s_tabStops[4] = {150, 210, 270, 330};
|
||||
|
||||
static void* imguiMalloc(size_t size, void* /*_userptr*/)
|
||||
{
|
||||
return malloc(size);
|
||||
}
|
||||
// For a custom allocator, define this and implement imguiMalloc and imguiFree somewhere in the project.
|
||||
#ifndef IMGUI_CONFIG_CUSTOM_ALLOCATOR
|
||||
# define IMGUI_CONFIG_CUSTOM_ALLOCATOR 0
|
||||
#endif // ENTRY_CONFIG_USE_TINYSTL
|
||||
|
||||
static void imguiFree(void* _ptr, void* /*_userptr*/)
|
||||
{
|
||||
free(_ptr);
|
||||
}
|
||||
#if IMGUI_CONFIG_CUSTOM_ALLOCATOR
|
||||
void* imguiMalloc(size_t size, void* /*_userptr*/);
|
||||
void imguiFree(void* _ptr, void* /*_userptr*/);
|
||||
#else
|
||||
static void* imguiMalloc(size_t _size, void* /*_userptr*/)
|
||||
{
|
||||
return malloc(_size);
|
||||
}
|
||||
|
||||
static void imguiFree(void* _ptr, void* /*_userptr*/)
|
||||
{
|
||||
free(_ptr);
|
||||
}
|
||||
#endif //IMGUI_CONFIG_CUSTOM_ALLOCATOR
|
||||
|
||||
#define IMGUI_MIN(_a, _b) (_a)<(_b)?(_a):(_b)
|
||||
#define IMGUI_MAX(_a, _b) (_a)>(_b)?(_a):(_b)
|
||||
@ -393,6 +405,7 @@ struct Imgui
|
||||
m_colorProgram.idx = bgfx::invalidHandle;
|
||||
m_textureProgram.idx = bgfx::invalidHandle;
|
||||
m_cubeMapProgram.idx = bgfx::invalidHandle;
|
||||
m_latlongProgram.idx = bgfx::invalidHandle;
|
||||
m_imageProgram.idx = bgfx::invalidHandle;
|
||||
m_imageSwizzProgram.idx = bgfx::invalidHandle;
|
||||
}
|
||||
@ -481,6 +494,8 @@ struct Imgui
|
||||
const bgfx::Memory* fs_imgui_texture;
|
||||
const bgfx::Memory* vs_imgui_cubemap;
|
||||
const bgfx::Memory* fs_imgui_cubemap;
|
||||
const bgfx::Memory* vs_imgui_latlong;
|
||||
const bgfx::Memory* fs_imgui_latlong;
|
||||
const bgfx::Memory* vs_imgui_image;
|
||||
const bgfx::Memory* fs_imgui_image;
|
||||
const bgfx::Memory* fs_imgui_image_swizz;
|
||||
@ -494,6 +509,8 @@ struct Imgui
|
||||
fs_imgui_texture = bgfx::makeRef(fs_imgui_texture_dx9, sizeof(fs_imgui_texture_dx9) );
|
||||
vs_imgui_cubemap = bgfx::makeRef(vs_imgui_cubemap_dx9, sizeof(vs_imgui_cubemap_dx9) );
|
||||
fs_imgui_cubemap = bgfx::makeRef(fs_imgui_cubemap_dx9, sizeof(fs_imgui_cubemap_dx9) );
|
||||
vs_imgui_latlong = bgfx::makeRef(vs_imgui_latlong_dx9, sizeof(vs_imgui_latlong_dx9) );
|
||||
fs_imgui_latlong = bgfx::makeRef(fs_imgui_latlong_dx9, sizeof(fs_imgui_latlong_dx9) );
|
||||
vs_imgui_image = bgfx::makeRef(vs_imgui_image_dx9, sizeof(vs_imgui_image_dx9) );
|
||||
fs_imgui_image = bgfx::makeRef(fs_imgui_image_dx9, sizeof(fs_imgui_image_dx9) );
|
||||
fs_imgui_image_swizz = bgfx::makeRef(fs_imgui_image_swizz_dx9, sizeof(fs_imgui_image_swizz_dx9) );
|
||||
@ -507,6 +524,8 @@ struct Imgui
|
||||
fs_imgui_texture = bgfx::makeRef(fs_imgui_texture_dx11, sizeof(fs_imgui_texture_dx11) );
|
||||
vs_imgui_cubemap = bgfx::makeRef(vs_imgui_cubemap_dx11, sizeof(vs_imgui_cubemap_dx11) );
|
||||
fs_imgui_cubemap = bgfx::makeRef(fs_imgui_cubemap_dx11, sizeof(fs_imgui_cubemap_dx11) );
|
||||
vs_imgui_latlong = bgfx::makeRef(vs_imgui_latlong_dx11, sizeof(vs_imgui_latlong_dx11) );
|
||||
fs_imgui_latlong = bgfx::makeRef(fs_imgui_latlong_dx11, sizeof(fs_imgui_latlong_dx11) );
|
||||
vs_imgui_image = bgfx::makeRef(vs_imgui_image_dx11, sizeof(vs_imgui_image_dx11) );
|
||||
fs_imgui_image = bgfx::makeRef(fs_imgui_image_dx11, sizeof(fs_imgui_image_dx11) );
|
||||
fs_imgui_image_swizz = bgfx::makeRef(fs_imgui_image_swizz_dx11, sizeof(fs_imgui_image_swizz_dx11) );
|
||||
@ -519,6 +538,8 @@ struct Imgui
|
||||
fs_imgui_texture = bgfx::makeRef(fs_imgui_texture_glsl, sizeof(fs_imgui_texture_glsl) );
|
||||
vs_imgui_cubemap = bgfx::makeRef(vs_imgui_cubemap_glsl, sizeof(vs_imgui_cubemap_glsl) );
|
||||
fs_imgui_cubemap = bgfx::makeRef(fs_imgui_cubemap_glsl, sizeof(fs_imgui_cubemap_glsl) );
|
||||
vs_imgui_latlong = bgfx::makeRef(vs_imgui_latlong_glsl, sizeof(vs_imgui_latlong_glsl) );
|
||||
fs_imgui_latlong = bgfx::makeRef(fs_imgui_latlong_glsl, sizeof(fs_imgui_latlong_glsl) );
|
||||
vs_imgui_image = bgfx::makeRef(vs_imgui_image_glsl, sizeof(vs_imgui_image_glsl) );
|
||||
fs_imgui_image = bgfx::makeRef(fs_imgui_image_glsl, sizeof(fs_imgui_image_glsl) );
|
||||
fs_imgui_image_swizz = bgfx::makeRef(fs_imgui_image_swizz_glsl, sizeof(fs_imgui_image_swizz_glsl) );
|
||||
@ -546,6 +567,12 @@ struct Imgui
|
||||
bgfx::destroyShader(vsh);
|
||||
bgfx::destroyShader(fsh);
|
||||
|
||||
vsh = bgfx::createShader(vs_imgui_latlong);
|
||||
fsh = bgfx::createShader(fs_imgui_latlong);
|
||||
m_latlongProgram = bgfx::createProgram(vsh, fsh);
|
||||
bgfx::destroyShader(vsh);
|
||||
bgfx::destroyShader(fsh);
|
||||
|
||||
vsh = bgfx::createShader(vs_imgui_image);
|
||||
fsh = bgfx::createShader(fs_imgui_image);
|
||||
m_imageProgram = bgfx::createProgram(vsh, fsh);
|
||||
@ -586,6 +613,7 @@ struct Imgui
|
||||
bgfx::destroyProgram(m_colorProgram);
|
||||
bgfx::destroyProgram(m_textureProgram);
|
||||
bgfx::destroyProgram(m_cubeMapProgram);
|
||||
bgfx::destroyProgram(m_latlongProgram);
|
||||
bgfx::destroyProgram(m_imageProgram);
|
||||
bgfx::destroyProgram(m_imageSwizzProgram);
|
||||
nvgDelete(m_nvg);
|
||||
@ -1594,24 +1622,27 @@ struct Imgui
|
||||
const int32_t yy = area.m_widgetY;
|
||||
area.m_widgetY += _height + DEFAULT_SPACING;
|
||||
|
||||
const bool enabled = _enabled && isEnabled(m_areaId);
|
||||
const bool over = enabled && inRect(xx, yy, _width, _height);
|
||||
const bool res = buttonLogic(id, over);
|
||||
if (screenQuad(xx, yy, _width, _height, _originBottomLeft) )
|
||||
{
|
||||
const bool enabled = _enabled && isEnabled(m_areaId);
|
||||
const bool over = enabled && inRect(xx, yy, _width, _height);
|
||||
const bool res = buttonLogic(id, over);
|
||||
|
||||
const float lodEnabled[4] = { _lod, float(enabled), 0.0f, 0.0f };
|
||||
const float lodEnabled[4] = { _lod, float(enabled), 0.0f, 0.0f };
|
||||
bgfx::setUniform(u_imageLodEnabled, lodEnabled);
|
||||
bgfx::setTexture(0, s_texColor, bgfx::isValid(_image) ? _image : m_missingTexture);
|
||||
bgfx::setState(BGFX_STATE_RGB_WRITE
|
||||
|BGFX_STATE_ALPHA_WRITE
|
||||
|BGFX_STATE_BLEND_FUNC(BGFX_STATE_BLEND_SRC_ALPHA, BGFX_STATE_BLEND_INV_SRC_ALPHA)
|
||||
);
|
||||
bgfx::setProgram(m_imageProgram);
|
||||
setCurrentScissor();
|
||||
bgfx::submit(m_view);
|
||||
|
||||
screenQuad(xx, yy, _width, _height, _originBottomLeft);
|
||||
bgfx::setUniform(u_imageLodEnabled, lodEnabled);
|
||||
bgfx::setTexture(0, s_texColor, bgfx::isValid(_image) ? _image : m_missingTexture);
|
||||
bgfx::setState(BGFX_STATE_RGB_WRITE
|
||||
|BGFX_STATE_ALPHA_WRITE
|
||||
|BGFX_STATE_BLEND_FUNC(BGFX_STATE_BLEND_SRC_ALPHA, BGFX_STATE_BLEND_INV_SRC_ALPHA)
|
||||
);
|
||||
bgfx::setProgram(m_imageProgram);
|
||||
setCurrentScissor();
|
||||
bgfx::submit(m_view);
|
||||
return res;
|
||||
}
|
||||
|
||||
return res;
|
||||
return false;
|
||||
}
|
||||
|
||||
bool image(bgfx::TextureHandle _image, float _lod, float _width, float _aspect, ImguiAlign::Enum _align, bool _enabled, bool _originBottomLeft)
|
||||
@ -1654,29 +1685,32 @@ struct Imgui
|
||||
const int32_t yy = area.m_widgetY;
|
||||
area.m_widgetY += _height + DEFAULT_SPACING;
|
||||
|
||||
const bool enabled = _enabled && isEnabled(m_areaId);
|
||||
const bool over = enabled && inRect(xx, yy, _width, _height);
|
||||
const bool res = buttonLogic(id, over);
|
||||
if (screenQuad(xx, yy, _width, _height) )
|
||||
{
|
||||
const bool enabled = _enabled && isEnabled(m_areaId);
|
||||
const bool over = enabled && inRect(xx, yy, _width, _height);
|
||||
const bool res = buttonLogic(id, over);
|
||||
|
||||
screenQuad(xx, yy, _width, _height);
|
||||
const float lodEnabled[4] = { _lod, float(enabled), 0.0f, 0.0f };
|
||||
bgfx::setUniform(u_imageLodEnabled, lodEnabled);
|
||||
|
||||
const float lodEnabled[4] = { _lod, float(enabled), 0.0f, 0.0f };
|
||||
bgfx::setUniform(u_imageLodEnabled, lodEnabled);
|
||||
float swizz[4] = { 0.0f, 0.0f, 0.0f, 0.0f };
|
||||
swizz[_channel] = 1.0f;
|
||||
bgfx::setUniform(u_imageSwizzle, swizz);
|
||||
|
||||
float swizz[4] = { 0.0f, 0.0f, 0.0f, 0.0f };
|
||||
swizz[_channel] = 1.0f;
|
||||
bgfx::setUniform(u_imageSwizzle, swizz);
|
||||
bgfx::setTexture(0, s_texColor, bgfx::isValid(_image) ? _image : m_missingTexture);
|
||||
bgfx::setState(BGFX_STATE_RGB_WRITE
|
||||
|BGFX_STATE_ALPHA_WRITE
|
||||
|BGFX_STATE_BLEND_FUNC(BGFX_STATE_BLEND_SRC_ALPHA, BGFX_STATE_BLEND_INV_SRC_ALPHA)
|
||||
);
|
||||
bgfx::setProgram(m_imageSwizzProgram);
|
||||
setCurrentScissor();
|
||||
bgfx::submit(m_view);
|
||||
|
||||
bgfx::setTexture(0, s_texColor, bgfx::isValid(_image) ? _image : m_missingTexture);
|
||||
bgfx::setState(BGFX_STATE_RGB_WRITE
|
||||
|BGFX_STATE_ALPHA_WRITE
|
||||
|BGFX_STATE_BLEND_FUNC(BGFX_STATE_BLEND_SRC_ALPHA, BGFX_STATE_BLEND_INV_SRC_ALPHA)
|
||||
);
|
||||
bgfx::setProgram(m_imageSwizzProgram);
|
||||
setCurrentScissor();
|
||||
bgfx::submit(m_view);
|
||||
return res;
|
||||
}
|
||||
|
||||
return res;
|
||||
return false;
|
||||
}
|
||||
|
||||
bool imageChannel(bgfx::TextureHandle _image, uint8_t _channel, float _lod, float _width, float _aspect, ImguiAlign::Enum _align, bool _enabled)
|
||||
@ -1687,8 +1721,96 @@ struct Imgui
|
||||
return imageChannel(_image, _channel, _lod, int32_t(width), int32_t(height), _align, _enabled);
|
||||
}
|
||||
|
||||
bool cubeMap(bgfx::TextureHandle _cubemap, float _lod, bool _cross, ImguiAlign::Enum _align, bool _enabled)
|
||||
bool latlong(bgfx::TextureHandle _cubemap, float _lod, ImguiAlign::Enum _align, bool _enabled)
|
||||
{
|
||||
const uint32_t id = getId();
|
||||
|
||||
Area& area = getCurrentArea();
|
||||
int32_t xx;
|
||||
int32_t width;
|
||||
if (ImguiAlign::Left == _align)
|
||||
{
|
||||
xx = area.m_contentX + SCROLL_AREA_PADDING;
|
||||
width = area.m_widgetW;
|
||||
}
|
||||
else if (ImguiAlign::LeftIndented == _align
|
||||
|| ImguiAlign::Right == _align)
|
||||
{
|
||||
xx = area.m_widgetX;
|
||||
width = area.m_widgetW;
|
||||
}
|
||||
else //if (ImguiAlign::Center == _align
|
||||
//|| ImguiAlign::CenterIndented == _align).
|
||||
{
|
||||
xx = area.m_widgetX;
|
||||
width = area.m_widgetW - (area.m_widgetX-area.m_scissorX);
|
||||
}
|
||||
|
||||
const int32_t height = width/2;
|
||||
const int32_t yy = area.m_widgetY;
|
||||
area.m_widgetY += height + DEFAULT_SPACING;
|
||||
|
||||
if (screenQuad(xx, yy, width, height, false) )
|
||||
{
|
||||
const bool enabled = _enabled && isEnabled(m_areaId);
|
||||
const bool over = enabled && inRect(xx, yy, width, height);
|
||||
const bool res = buttonLogic(id, over);
|
||||
|
||||
const float lodEnabled[4] = { _lod, float(enabled), 0.0f, 0.0f };
|
||||
bgfx::setUniform(u_imageLodEnabled, lodEnabled);
|
||||
|
||||
bgfx::setTexture(0, s_texColor, _cubemap);
|
||||
bgfx::setState(BGFX_STATE_RGB_WRITE
|
||||
|BGFX_STATE_ALPHA_WRITE
|
||||
|BGFX_STATE_BLEND_FUNC(BGFX_STATE_BLEND_SRC_ALPHA, BGFX_STATE_BLEND_INV_SRC_ALPHA)
|
||||
);
|
||||
bgfx::setProgram(m_latlongProgram);
|
||||
setCurrentScissor();
|
||||
bgfx::submit(m_view);
|
||||
|
||||
return res;
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
bool cubeMap(bgfx::TextureHandle _cubemap, float _lod, bool _cross, bool _sameHeight, ImguiAlign::Enum _align, bool _enabled)
|
||||
{
|
||||
const uint32_t id = getId();
|
||||
|
||||
Area& area = getCurrentArea();
|
||||
int32_t xx;
|
||||
int32_t width;
|
||||
if (ImguiAlign::Left == _align)
|
||||
{
|
||||
xx = area.m_contentX + SCROLL_AREA_PADDING;
|
||||
width = area.m_widgetW;
|
||||
}
|
||||
else if (ImguiAlign::LeftIndented == _align
|
||||
|| ImguiAlign::Right == _align)
|
||||
{
|
||||
xx = area.m_widgetX;
|
||||
width = area.m_widgetW;
|
||||
}
|
||||
else //if (ImguiAlign::Center == _align
|
||||
//|| ImguiAlign::CenterIndented == _align).
|
||||
{
|
||||
xx = area.m_widgetX;
|
||||
width = area.m_widgetW - (area.m_widgetX-area.m_scissorX);
|
||||
}
|
||||
|
||||
const bool adjustHeight = (_cross && _sameHeight);
|
||||
const bool fullHeight = (_cross && !_sameHeight);
|
||||
|
||||
if (adjustHeight)
|
||||
{
|
||||
xx += width/6;
|
||||
}
|
||||
|
||||
const int32_t height = fullHeight ? (width*3)/4 : (width/2);
|
||||
const int32_t yy = area.m_widgetY;
|
||||
area.m_widgetY += height + DEFAULT_SPACING;
|
||||
|
||||
const uint32_t numVertices = 14;
|
||||
const uint32_t numIndices = 36;
|
||||
if (bgfx::checkAvailTransientBuffers(numVertices, PosNormalVertex::ms_decl, numIndices) )
|
||||
@ -1760,38 +1882,12 @@ struct Imgui
|
||||
indices += addQuad(indices, 10, 12, 13, 11);
|
||||
}
|
||||
|
||||
const uint32_t id = getId();
|
||||
|
||||
Area& area = getCurrentArea();
|
||||
int32_t xx;
|
||||
int32_t width;
|
||||
if (ImguiAlign::Left == _align)
|
||||
{
|
||||
xx = area.m_contentX + SCROLL_AREA_PADDING;
|
||||
width = area.m_widgetW;
|
||||
}
|
||||
else if (ImguiAlign::LeftIndented == _align
|
||||
|| ImguiAlign::Right == _align)
|
||||
{
|
||||
xx = area.m_widgetX;
|
||||
width = area.m_widgetW;
|
||||
}
|
||||
else //if (ImguiAlign::Center == _align
|
||||
//|| ImguiAlign::CenterIndented == _align).
|
||||
{
|
||||
xx = area.m_widgetX;
|
||||
width = area.m_widgetW - (area.m_widgetX-area.m_scissorX);
|
||||
}
|
||||
|
||||
const uint32_t height = _cross ? (width*3)/4 : (width/2);
|
||||
const int32_t yy = area.m_widgetY;
|
||||
area.m_widgetY += height + DEFAULT_SPACING;
|
||||
|
||||
const bool enabled = _enabled && isEnabled(m_areaId);
|
||||
const bool over = enabled && inRect(xx, yy, width, height);
|
||||
const bool res = buttonLogic(id, over);
|
||||
|
||||
const float scale = float(width/2)+0.25f;
|
||||
const float widthf = float(width);
|
||||
const float scale = adjustHeight ? (widthf+0.5f)/3.0f : (widthf*0.5f + 0.25f);
|
||||
|
||||
float mtx[16];
|
||||
bx::mtxSRT(mtx, scale, scale, 1.0f, 0.0f, 0.0f, 0.0f, float(xx), float(yy), 0.0f);
|
||||
@ -1817,6 +1913,19 @@ struct Imgui
|
||||
return false;
|
||||
}
|
||||
|
||||
bool cubeMap(bgfx::TextureHandle _cubemap, float _lod, ImguiCubemap::Enum _display, bool _sameHeight, ImguiAlign::Enum _align, bool _enabled)
|
||||
{
|
||||
if (ImguiCubemap::Cross == _display
|
||||
|| ImguiCubemap::Hex == _display)
|
||||
{
|
||||
return cubeMap(_cubemap, _lod, (ImguiCubemap::Cross == _display), _sameHeight, _align, _enabled);
|
||||
}
|
||||
else //(ImguiCubemap::Latlong == _display).
|
||||
{
|
||||
return latlong(_cubemap, _lod, _align, _enabled);
|
||||
}
|
||||
}
|
||||
|
||||
bool collapse(const char* _text, const char* _subtext, bool _checked, bool _enabled)
|
||||
{
|
||||
const uint32_t id = getId();
|
||||
@ -2596,7 +2705,7 @@ struct Imgui
|
||||
#endif // USE_NANOVG_FONT
|
||||
}
|
||||
|
||||
void screenQuad(int32_t _x, int32_t _y, int32_t _width, uint32_t _height, bool _originBottomLeft = false)
|
||||
bool screenQuad(int32_t _x, int32_t _y, int32_t _width, uint32_t _height, bool _originBottomLeft = false)
|
||||
{
|
||||
if (bgfx::checkAvailTransientVertexBuffer(6, PosUvVertex::ms_decl) )
|
||||
{
|
||||
@ -2650,7 +2759,11 @@ struct Imgui
|
||||
vertex[5].m_v = minv;
|
||||
|
||||
bgfx::setVertexBuffer(&vb);
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
void colorWheelWidget(float _rgb[3], bool _respectIndentation, float _size, bool _enabled)
|
||||
@ -3054,6 +3167,7 @@ struct Imgui
|
||||
bgfx::ProgramHandle m_colorProgram;
|
||||
bgfx::ProgramHandle m_textureProgram;
|
||||
bgfx::ProgramHandle m_cubeMapProgram;
|
||||
bgfx::ProgramHandle m_latlongProgram;
|
||||
bgfx::ProgramHandle m_imageProgram;
|
||||
bgfx::ProgramHandle m_imageSwizzProgram;
|
||||
bgfx::TextureHandle m_missingTexture;
|
||||
@ -3348,9 +3462,9 @@ bool imguiImageChannel(bgfx::TextureHandle _image, uint8_t _channel, float _lod,
|
||||
return s_imgui.imageChannel(_image, _channel, _lod, _width, _aspect, _align, _enabled);
|
||||
}
|
||||
|
||||
bool imguiCube(bgfx::TextureHandle _cubemap, float _lod, bool _cross, ImguiAlign::Enum _align, bool _enabled)
|
||||
bool imguiCube(bgfx::TextureHandle _cubemap, float _lod, ImguiCubemap::Enum _display, bool _sameHeight, ImguiAlign::Enum _align, bool _enabled)
|
||||
{
|
||||
return s_imgui.cubeMap(_cubemap, _lod, _cross, _align, _enabled);
|
||||
return s_imgui.cubeMap(_cubemap, _lod, _display, _sameHeight, _align, _enabled);
|
||||
}
|
||||
|
||||
float imguiGetTextLength(const char* _text, ImguiFontHandle _handle)
|
||||
|
14
3rdparty/bgfx/examples/common/imgui/imgui.h
vendored
14
3rdparty/bgfx/examples/common/imgui/imgui.h
vendored
@ -94,6 +94,18 @@ struct ImguiAlign
|
||||
};
|
||||
};
|
||||
|
||||
struct ImguiCubemap
|
||||
{
|
||||
enum Enum
|
||||
{
|
||||
Cross,
|
||||
Latlong,
|
||||
Hex,
|
||||
|
||||
Count,
|
||||
};
|
||||
};
|
||||
|
||||
struct ImguiBorder
|
||||
{
|
||||
enum Enum
|
||||
@ -184,7 +196,7 @@ bool imguiImage(bgfx::TextureHandle _image, float _lod, int32_t _width, int32_t
|
||||
bool imguiImage(bgfx::TextureHandle _image, float _lod, float _scale, float _aspect, ImguiAlign::Enum _align = ImguiAlign::LeftIndented, bool _enabled = true, bool _originBottomLeft = false);
|
||||
bool imguiImageChannel(bgfx::TextureHandle _image, uint8_t _channel, float _lod, int32_t _width, int32_t _height, ImguiAlign::Enum _align = ImguiAlign::LeftIndented, bool _enabled = true);
|
||||
bool imguiImageChannel(bgfx::TextureHandle _image, uint8_t _channel, float _lod, float _scale, float _aspect, ImguiAlign::Enum _align = ImguiAlign::LeftIndented, bool _enabled = true);
|
||||
bool imguiCube(bgfx::TextureHandle _cubemap, float _lod = 0.0f, bool _cross = true, ImguiAlign::Enum _align = ImguiAlign::LeftIndented, bool _enabled = true);
|
||||
bool imguiCube(bgfx::TextureHandle _cubemap, float _lod = 0.0f, ImguiCubemap::Enum _display = ImguiCubemap::Cross, bool _sameHeight = false, ImguiAlign::Enum _align = ImguiAlign::LeftIndented, bool _enabled = true);
|
||||
|
||||
float imguiGetTextLength(const char* _text, ImguiFontHandle _handle);
|
||||
bool imguiMouseOverArea();
|
||||
|
84
3rdparty/bgfx/examples/common/imgui/vs_imgui_latlong.bin.h
vendored
Normal file
84
3rdparty/bgfx/examples/common/imgui/vs_imgui_latlong.bin.h
vendored
Normal file
@ -0,0 +1,84 @@
|
||||
static const uint8_t vs_imgui_latlong_glsl[337] =
|
||||
{
|
||||
0x56, 0x53, 0x48, 0x03, 0x6f, 0x1e, 0x3e, 0x3c, 0x01, 0x00, 0x0f, 0x75, 0x5f, 0x6d, 0x6f, 0x64, // VSH.o.><...u_mod
|
||||
0x65, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x50, 0x72, 0x6f, 0x6a, 0x09, 0x01, 0x00, 0x00, 0x01, 0x00, // elViewProj......
|
||||
0x2c, 0x01, 0x00, 0x00, 0x61, 0x74, 0x74, 0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x20, 0x68, 0x69, // ,...attribute hi
|
||||
0x67, 0x68, 0x70, 0x20, 0x76, 0x65, 0x63, 0x33, 0x20, 0x61, 0x5f, 0x70, 0x6f, 0x73, 0x69, 0x74, // ghp vec3 a_posit
|
||||
0x69, 0x6f, 0x6e, 0x3b, 0x0a, 0x61, 0x74, 0x74, 0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x20, 0x68, // ion;.attribute h
|
||||
0x69, 0x67, 0x68, 0x70, 0x20, 0x76, 0x65, 0x63, 0x32, 0x20, 0x61, 0x5f, 0x74, 0x65, 0x78, 0x63, // ighp vec2 a_texc
|
||||
0x6f, 0x6f, 0x72, 0x64, 0x30, 0x3b, 0x0a, 0x76, 0x61, 0x72, 0x79, 0x69, 0x6e, 0x67, 0x20, 0x68, // oord0;.varying h
|
||||
0x69, 0x67, 0x68, 0x70, 0x20, 0x76, 0x65, 0x63, 0x32, 0x20, 0x76, 0x5f, 0x74, 0x65, 0x78, 0x63, // ighp vec2 v_texc
|
||||
0x6f, 0x6f, 0x72, 0x64, 0x30, 0x3b, 0x0a, 0x75, 0x6e, 0x69, 0x66, 0x6f, 0x72, 0x6d, 0x20, 0x68, // oord0;.uniform h
|
||||
0x69, 0x67, 0x68, 0x70, 0x20, 0x6d, 0x61, 0x74, 0x34, 0x20, 0x75, 0x5f, 0x6d, 0x6f, 0x64, 0x65, // ighp mat4 u_mode
|
||||
0x6c, 0x56, 0x69, 0x65, 0x77, 0x50, 0x72, 0x6f, 0x6a, 0x3b, 0x0a, 0x76, 0x6f, 0x69, 0x64, 0x20, // lViewProj;.void
|
||||
0x6d, 0x61, 0x69, 0x6e, 0x20, 0x28, 0x29, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x68, 0x69, 0x67, 0x68, // main ().{. high
|
||||
0x70, 0x20, 0x76, 0x65, 0x63, 0x34, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x31, 0x3b, // p vec4 tmpvar_1;
|
||||
0x0a, 0x20, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x31, 0x2e, 0x77, 0x20, 0x3d, 0x20, // . tmpvar_1.w =
|
||||
0x31, 0x2e, 0x30, 0x3b, 0x0a, 0x20, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x31, 0x2e, // 1.0;. tmpvar_1.
|
||||
0x78, 0x79, 0x7a, 0x20, 0x3d, 0x20, 0x61, 0x5f, 0x70, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e, // xyz = a_position
|
||||
0x3b, 0x0a, 0x20, 0x20, 0x67, 0x6c, 0x5f, 0x50, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x20, // ;. gl_Position
|
||||
0x3d, 0x20, 0x28, 0x75, 0x5f, 0x6d, 0x6f, 0x64, 0x65, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x50, 0x72, // = (u_modelViewPr
|
||||
0x6f, 0x6a, 0x20, 0x2a, 0x20, 0x74, 0x6d, 0x70, 0x76, 0x61, 0x72, 0x5f, 0x31, 0x29, 0x3b, 0x0a, // oj * tmpvar_1);.
|
||||
0x20, 0x20, 0x76, 0x5f, 0x74, 0x65, 0x78, 0x63, 0x6f, 0x6f, 0x72, 0x64, 0x30, 0x20, 0x3d, 0x20, // v_texcoord0 =
|
||||
0x61, 0x5f, 0x74, 0x65, 0x78, 0x63, 0x6f, 0x6f, 0x72, 0x64, 0x30, 0x3b, 0x0a, 0x7d, 0x0a, 0x0a, // a_texcoord0;.}..
|
||||
0x00, // .
|
||||
};
|
||||
static const uint8_t vs_imgui_latlong_dx9[319] =
|
||||
{
|
||||
0x56, 0x53, 0x48, 0x03, 0x6f, 0x1e, 0x3e, 0x3c, 0x01, 0x00, 0x0f, 0x75, 0x5f, 0x6d, 0x6f, 0x64, // VSH.o.><...u_mod
|
||||
0x65, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x50, 0x72, 0x6f, 0x6a, 0x09, 0x01, 0x00, 0x00, 0x04, 0x00, // elViewProj......
|
||||
0x1c, 0x01, 0x00, 0x03, 0xfe, 0xff, 0xfe, 0xff, 0x23, 0x00, 0x43, 0x54, 0x41, 0x42, 0x1c, 0x00, // ........#.CTAB..
|
||||
0x00, 0x00, 0x57, 0x00, 0x00, 0x00, 0x00, 0x03, 0xfe, 0xff, 0x01, 0x00, 0x00, 0x00, 0x1c, 0x00, // ..W.............
|
||||
0x00, 0x00, 0x00, 0x81, 0x00, 0x00, 0x50, 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x02, 0x00, // ......P...0.....
|
||||
0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x40, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x75, 0x5f, // ......@.......u_
|
||||
0x6d, 0x6f, 0x64, 0x65, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x50, 0x72, 0x6f, 0x6a, 0x00, 0x03, 0x00, // modelViewProj...
|
||||
0x03, 0x00, 0x04, 0x00, 0x04, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x76, 0x73, // ..............vs
|
||||
0x5f, 0x33, 0x5f, 0x30, 0x00, 0x4d, 0x69, 0x63, 0x72, 0x6f, 0x73, 0x6f, 0x66, 0x74, 0x20, 0x28, // _3_0.Microsoft (
|
||||
0x52, 0x29, 0x20, 0x48, 0x4c, 0x53, 0x4c, 0x20, 0x53, 0x68, 0x61, 0x64, 0x65, 0x72, 0x20, 0x43, // R) HLSL Shader C
|
||||
0x6f, 0x6d, 0x70, 0x69, 0x6c, 0x65, 0x72, 0x20, 0x39, 0x2e, 0x32, 0x39, 0x2e, 0x39, 0x35, 0x32, // ompiler 9.29.952
|
||||
0x2e, 0x33, 0x31, 0x31, 0x31, 0x00, 0x1f, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, // .3111...........
|
||||
0x0f, 0x90, 0x1f, 0x00, 0x00, 0x02, 0x05, 0x00, 0x00, 0x80, 0x01, 0x00, 0x0f, 0x90, 0x1f, 0x00, // ................
|
||||
0x00, 0x02, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0x0f, 0xe0, 0x1f, 0x00, 0x00, 0x02, 0x05, 0x00, // ................
|
||||
0x00, 0x80, 0x01, 0x00, 0x03, 0xe0, 0x05, 0x00, 0x00, 0x03, 0x00, 0x00, 0x0f, 0x80, 0x01, 0x00, // ................
|
||||
0xe4, 0xa0, 0x00, 0x00, 0x55, 0x90, 0x04, 0x00, 0x00, 0x04, 0x00, 0x00, 0x0f, 0x80, 0x00, 0x00, // ....U...........
|
||||
0xe4, 0xa0, 0x00, 0x00, 0x00, 0x90, 0x00, 0x00, 0xe4, 0x80, 0x04, 0x00, 0x00, 0x04, 0x00, 0x00, // ................
|
||||
0x0f, 0x80, 0x02, 0x00, 0xe4, 0xa0, 0x00, 0x00, 0xaa, 0x90, 0x00, 0x00, 0xe4, 0x80, 0x02, 0x00, // ................
|
||||
0x00, 0x03, 0x00, 0x00, 0x0f, 0xe0, 0x00, 0x00, 0xe4, 0x80, 0x03, 0x00, 0xe4, 0xa0, 0x01, 0x00, // ................
|
||||
0x00, 0x02, 0x01, 0x00, 0x03, 0xe0, 0x01, 0x00, 0xe4, 0x90, 0xff, 0xff, 0x00, 0x00, 0x00, // ...............
|
||||
};
|
||||
static const uint8_t vs_imgui_latlong_dx11[518] =
|
||||
{
|
||||
0x56, 0x53, 0x48, 0x03, 0x6f, 0x1e, 0x3e, 0x3c, 0x01, 0x00, 0x0f, 0x75, 0x5f, 0x6d, 0x6f, 0x64, // VSH.o.><...u_mod
|
||||
0x65, 0x6c, 0x56, 0x69, 0x65, 0x77, 0x50, 0x72, 0x6f, 0x6a, 0x09, 0x00, 0xe0, 0x09, 0x04, 0x00, // elViewProj......
|
||||
0xdc, 0x01, 0x44, 0x58, 0x42, 0x43, 0xb0, 0x50, 0xdf, 0x0d, 0x1e, 0x85, 0x4e, 0xbe, 0xdd, 0xef, // ..DXBC.P....N...
|
||||
0x92, 0x24, 0x45, 0x46, 0x54, 0x49, 0x01, 0x00, 0x00, 0x00, 0xdc, 0x01, 0x00, 0x00, 0x03, 0x00, // .$EFTI..........
|
||||
0x00, 0x00, 0x2c, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0x00, 0xd8, 0x00, 0x00, 0x00, 0x49, 0x53, // ..,...........IS
|
||||
0x47, 0x4e, 0x4c, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x38, 0x00, // GNL...........8.
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, // ................
|
||||
0x00, 0x00, 0x07, 0x07, 0x00, 0x00, 0x41, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, // ......A.........
|
||||
0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x03, 0x03, 0x00, 0x00, 0x50, 0x4f, // ..............PO
|
||||
0x53, 0x49, 0x54, 0x49, 0x4f, 0x4e, 0x00, 0x54, 0x45, 0x58, 0x43, 0x4f, 0x4f, 0x52, 0x44, 0x00, // SITION.TEXCOORD.
|
||||
0xab, 0xab, 0x4f, 0x53, 0x47, 0x4e, 0x50, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x08, 0x00, // ..OSGNP.........
|
||||
0x00, 0x00, 0x38, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x03, 0x00, // ..8.............
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0f, 0x00, 0x00, 0x00, 0x44, 0x00, 0x00, 0x00, 0x00, 0x00, // ..........D.....
|
||||
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x03, 0x0c, // ................
|
||||
0x00, 0x00, 0x53, 0x56, 0x5f, 0x50, 0x4f, 0x53, 0x49, 0x54, 0x49, 0x4f, 0x4e, 0x00, 0x54, 0x45, // ..SV_POSITION.TE
|
||||
0x58, 0x43, 0x4f, 0x4f, 0x52, 0x44, 0x00, 0xab, 0xab, 0xab, 0x53, 0x48, 0x44, 0x52, 0xfc, 0x00, // XCOORD....SHDR..
|
||||
0x00, 0x00, 0x40, 0x00, 0x01, 0x00, 0x3f, 0x00, 0x00, 0x00, 0x59, 0x00, 0x00, 0x04, 0x46, 0x8e, // ..@...?...Y...F.
|
||||
0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0xa2, 0x00, 0x00, 0x00, 0x5f, 0x00, 0x00, 0x03, 0x72, 0x10, // ........._...r.
|
||||
0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x5f, 0x00, 0x00, 0x03, 0x32, 0x10, 0x10, 0x00, 0x01, 0x00, // ......_...2.....
|
||||
0x00, 0x00, 0x67, 0x00, 0x00, 0x04, 0xf2, 0x20, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, // ..g.... ........
|
||||
0x00, 0x00, 0x65, 0x00, 0x00, 0x03, 0x32, 0x20, 0x10, 0x00, 0x01, 0x00, 0x00, 0x00, 0x68, 0x00, // ..e...2 ......h.
|
||||
0x00, 0x02, 0x01, 0x00, 0x00, 0x00, 0x38, 0x00, 0x00, 0x08, 0xf2, 0x00, 0x10, 0x00, 0x00, 0x00, // ......8.........
|
||||
0x00, 0x00, 0x56, 0x15, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x46, 0x8e, 0x20, 0x00, 0x00, 0x00, // ..V.......F. ...
|
||||
0x00, 0x00, 0x9f, 0x00, 0x00, 0x00, 0x32, 0x00, 0x00, 0x0a, 0xf2, 0x00, 0x10, 0x00, 0x00, 0x00, // ......2.........
|
||||
0x00, 0x00, 0x46, 0x8e, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0x9e, 0x00, 0x00, 0x00, 0x06, 0x10, // ..F. ...........
|
||||
0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x46, 0x0e, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x32, 0x00, // ......F.......2.
|
||||
0x00, 0x0a, 0xf2, 0x00, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x46, 0x8e, 0x20, 0x00, 0x00, 0x00, // ..........F. ...
|
||||
0x00, 0x00, 0xa0, 0x00, 0x00, 0x00, 0xa6, 0x1a, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x46, 0x0e, // ..............F.
|
||||
0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x08, 0xf2, 0x20, 0x10, 0x00, 0x00, 0x00, // ........... ....
|
||||
0x00, 0x00, 0x46, 0x0e, 0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x46, 0x8e, 0x20, 0x00, 0x00, 0x00, // ..F.......F. ...
|
||||
0x00, 0x00, 0xa1, 0x00, 0x00, 0x00, 0x36, 0x00, 0x00, 0x05, 0x32, 0x20, 0x10, 0x00, 0x01, 0x00, // ......6...2 ....
|
||||
0x00, 0x00, 0x46, 0x10, 0x10, 0x00, 0x01, 0x00, 0x00, 0x00, 0x3e, 0x00, 0x00, 0x01, 0x00, 0x02, // ..F.......>.....
|
||||
0x01, 0x00, 0x10, 0x00, 0x30, 0x0a, // ....0.
|
||||
};
|
15
3rdparty/bgfx/examples/common/imgui/vs_imgui_latlong.sc
vendored
Normal file
15
3rdparty/bgfx/examples/common/imgui/vs_imgui_latlong.sc
vendored
Normal file
@ -0,0 +1,15 @@
|
||||
$input a_position, a_texcoord0
|
||||
$output v_texcoord0
|
||||
|
||||
/*
|
||||
* Copyright 2015 Dario Manesku. All rights reserved.
|
||||
* License: http://www.opensource.org/licenses/BSD-2-Clause
|
||||
*/
|
||||
|
||||
#include <bgfx_shader.sh>
|
||||
|
||||
void main()
|
||||
{
|
||||
gl_Position = mul(u_modelViewProj, vec4(a_position, 1.0) );
|
||||
v_texcoord0 = a_texcoord0;
|
||||
}
|
3
3rdparty/bgfx/include/bgfx.c99.h
vendored
3
3rdparty/bgfx/include/bgfx.c99.h
vendored
@ -265,6 +265,7 @@ typedef struct bgfx_texture_info
|
||||
uint16_t depth;
|
||||
uint8_t numMips;
|
||||
uint8_t bitsPerPixel;
|
||||
bool cubeMap;
|
||||
|
||||
} bgfx_texture_info_t;
|
||||
|
||||
@ -870,7 +871,7 @@ BGFX_C_API void bgfx_destroy_program(bgfx_program_handle_t _handle);
|
||||
/**
|
||||
* Calculate amount of memory required for texture.
|
||||
*/
|
||||
BGFX_C_API void bgfx_calc_texture_size(bgfx_texture_info_t* _info, uint16_t _width, uint16_t _height, uint16_t _depth, uint8_t _numMips, bgfx_texture_format_t _format);
|
||||
BGFX_C_API void bgfx_calc_texture_size(bgfx_texture_info_t* _info, uint16_t _width, uint16_t _height, uint16_t _depth, bool _cubeMap, uint8_t _numMips, bgfx_texture_format_t _format);
|
||||
|
||||
/**
|
||||
* Create texture from memory buffer.
|
||||
|
3
3rdparty/bgfx/include/bgfx.h
vendored
3
3rdparty/bgfx/include/bgfx.h
vendored
@ -360,6 +360,7 @@ namespace bgfx
|
||||
uint16_t depth; //!< Texture depth.
|
||||
uint8_t numMips; //!< Number of MIP maps.
|
||||
uint8_t bitsPerPixel; //!< Format bits per pixel.
|
||||
bool cubeMap; //!< Texture is cubemap.
|
||||
};
|
||||
|
||||
///
|
||||
@ -817,7 +818,7 @@ namespace bgfx
|
||||
void destroyProgram(ProgramHandle _handle);
|
||||
|
||||
/// Calculate amount of memory required for texture.
|
||||
void calcTextureSize(TextureInfo& _info, uint16_t _width, uint16_t _height, uint16_t _depth, uint8_t _numMips, TextureFormat::Enum _format);
|
||||
void calcTextureSize(TextureInfo& _info, uint16_t _width, uint16_t _height, uint16_t _depth, bool _cubeMap, uint8_t _numMips, TextureFormat::Enum _format);
|
||||
|
||||
/// Create texture from memory buffer.
|
||||
///
|
||||
|
3
3rdparty/bgfx/include/bgfxplatform.h
vendored
3
3rdparty/bgfx/include/bgfxplatform.h
vendored
@ -51,12 +51,11 @@ namespace bgfx
|
||||
} // namespace bgfx
|
||||
|
||||
#elif BX_PLATFORM_FREEBSD || BX_PLATFORM_LINUX || BX_PLATFORM_RPI
|
||||
# include <X11/Xlib.h>
|
||||
|
||||
namespace bgfx
|
||||
{
|
||||
///
|
||||
void x11SetDisplayWindow(::Display* _display, ::Window _window);
|
||||
void x11SetDisplayWindow(void* _display, uint32_t _window);
|
||||
|
||||
} // namespace bgfx
|
||||
|
||||
|
9
3rdparty/bgfx/scripts/bgfx.lua
vendored
9
3rdparty/bgfx/scripts/bgfx.lua
vendored
@ -14,13 +14,16 @@ function bgfxProject(_name, _kind, _defines)
|
||||
"BGFX_SHARED_LIB_BUILD=1",
|
||||
}
|
||||
|
||||
configuration { "vs20* or mingw*" }
|
||||
links {
|
||||
"gdi32",
|
||||
"psapi",
|
||||
}
|
||||
|
||||
configuration { "mingw*" }
|
||||
linkoptions {
|
||||
"-shared",
|
||||
}
|
||||
links {
|
||||
"gdi32",
|
||||
}
|
||||
|
||||
configuration {}
|
||||
end
|
||||
|
59
3rdparty/bgfx/src/bgfx.cpp
vendored
59
3rdparty/bgfx/src/bgfx.cpp
vendored
@ -34,10 +34,10 @@ namespace bgfx
|
||||
g_bgfxEaglLayer = _layer;
|
||||
}
|
||||
#elif BX_PLATFORM_LINUX
|
||||
::Display* g_bgfxX11Display;
|
||||
::Window g_bgfxX11Window;
|
||||
void* g_bgfxX11Display;
|
||||
uint32_t g_bgfxX11Window;
|
||||
|
||||
void x11SetDisplayWindow(::Display* _display, ::Window _window)
|
||||
void x11SetDisplayWindow(void* _display, uint32_t _window)
|
||||
{
|
||||
g_bgfxX11Display = _display;
|
||||
g_bgfxX11Window = _window;
|
||||
@ -2309,27 +2309,33 @@ again:
|
||||
s_ctx->destroyProgram(_handle);
|
||||
}
|
||||
|
||||
void calcTextureSize(TextureInfo& _info, uint16_t _width, uint16_t _height, uint16_t _depth, uint8_t _numMips, TextureFormat::Enum _format)
|
||||
void calcTextureSize(TextureInfo& _info, uint16_t _width, uint16_t _height, uint16_t _depth, bool _cubeMap, uint8_t _numMips, TextureFormat::Enum _format)
|
||||
{
|
||||
_width = bx::uint32_max(1, _width);
|
||||
_height = bx::uint32_max(1, _height);
|
||||
const ImageBlockInfo& blockInfo = getBlockInfo(_format);
|
||||
const uint8_t bpp = blockInfo.bitsPerPixel;
|
||||
const uint32_t blockWidth = blockInfo.blockWidth;
|
||||
const uint32_t blockHeight = blockInfo.blockHeight;
|
||||
const uint32_t minBlockX = blockInfo.minBlockX;
|
||||
const uint32_t minBlockY = blockInfo.minBlockY;
|
||||
|
||||
_width = bx::uint32_max(blockWidth * minBlockX, ( (_width + blockWidth - 1) / blockWidth)*blockWidth);
|
||||
_height = bx::uint32_max(blockHeight * minBlockY, ( (_height + blockHeight - 1) / blockHeight)*blockHeight);
|
||||
_depth = bx::uint32_max(1, _depth);
|
||||
_numMips = bx::uint32_max(1, _numMips);
|
||||
|
||||
uint32_t width = _width;
|
||||
uint32_t height = _height;
|
||||
uint32_t depth = _depth;
|
||||
|
||||
uint32_t bpp = getBitsPerPixel(_format);
|
||||
uint32_t size = 0;
|
||||
uint32_t sides = _cubeMap ? 6 : 1;
|
||||
uint32_t size = 0;
|
||||
|
||||
for (uint32_t lod = 0; lod < _numMips; ++lod)
|
||||
{
|
||||
width = bx::uint32_max(1, width);
|
||||
height = bx::uint32_max(1, height);
|
||||
width = bx::uint32_max(blockWidth * minBlockX, ( (width + blockWidth - 1) / blockWidth )*blockWidth);
|
||||
height = bx::uint32_max(blockHeight * minBlockY, ( (height + blockHeight - 1) / blockHeight)*blockHeight);
|
||||
depth = bx::uint32_max(1, depth);
|
||||
|
||||
size += width*height*depth*bpp/8;
|
||||
size += width*height*depth*bpp/8 * sides;
|
||||
|
||||
width >>= 1;
|
||||
height >>= 1;
|
||||
@ -2341,6 +2347,7 @@ again:
|
||||
_info.height = _height;
|
||||
_info.depth = _depth;
|
||||
_info.numMips = _numMips;
|
||||
_info.cubeMap = _cubeMap;
|
||||
_info.storageSize = size;
|
||||
_info.bitsPerPixel = bpp;
|
||||
}
|
||||
@ -2362,7 +2369,7 @@ again:
|
||||
&& NULL != _mem)
|
||||
{
|
||||
TextureInfo ti;
|
||||
calcTextureSize(ti, _width, _height, 1, _numMips, _format);
|
||||
calcTextureSize(ti, _width, _height, 1, false, _numMips, _format);
|
||||
BX_CHECK(ti.storageSize == _mem->size
|
||||
, "createTexture2D: Texture storage size doesn't match passed memory size (storage size: %d, memory size: %d)"
|
||||
, ti.storageSize
|
||||
@ -2403,7 +2410,7 @@ again:
|
||||
&& NULL != _mem)
|
||||
{
|
||||
TextureInfo ti;
|
||||
calcTextureSize(ti, _width, _height, _depth, _numMips, _format);
|
||||
calcTextureSize(ti, _width, _height, _depth, false, _numMips, _format);
|
||||
BX_CHECK(ti.storageSize == _mem->size
|
||||
, "createTexture3D: Texture storage size doesn't match passed memory size (storage size: %d, memory size: %d)"
|
||||
, ti.storageSize
|
||||
@ -2443,10 +2450,10 @@ again:
|
||||
&& NULL != _mem)
|
||||
{
|
||||
TextureInfo ti;
|
||||
calcTextureSize(ti, _size, _size, 1, _numMips, _format);
|
||||
BX_CHECK(ti.storageSize*6 == _mem->size
|
||||
calcTextureSize(ti, _size, _size, 1, true, _numMips, _format);
|
||||
BX_CHECK(ti.storageSize == _mem->size
|
||||
, "createTextureCube: Texture storage size doesn't match passed memory size (storage size: %d, memory size: %d)"
|
||||
, ti.storageSize*6
|
||||
, ti.storageSize
|
||||
, _mem->size
|
||||
);
|
||||
}
|
||||
@ -2459,15 +2466,15 @@ again:
|
||||
bx::write(&writer, magic);
|
||||
|
||||
TextureCreate tc;
|
||||
tc.m_flags = _flags;
|
||||
tc.m_width = _size;
|
||||
tc.m_height = _size;
|
||||
tc.m_sides = 6;
|
||||
tc.m_depth = 0;
|
||||
tc.m_flags = _flags;
|
||||
tc.m_width = _size;
|
||||
tc.m_height = _size;
|
||||
tc.m_sides = 6;
|
||||
tc.m_depth = 0;
|
||||
tc.m_numMips = _numMips;
|
||||
tc.m_format = uint8_t(_format);
|
||||
tc.m_format = uint8_t(_format);
|
||||
tc.m_cubeMap = true;
|
||||
tc.m_mem = _mem;
|
||||
tc.m_mem = _mem;
|
||||
bx::write(&writer, tc);
|
||||
|
||||
return s_ctx->createTexture(mem, _flags, 0, NULL);
|
||||
@ -3194,10 +3201,10 @@ BGFX_C_API void bgfx_destroy_program(bgfx_program_handle_t _handle)
|
||||
bgfx::destroyProgram(handle.cpp);
|
||||
}
|
||||
|
||||
BGFX_C_API void bgfx_calc_texture_size(bgfx_texture_info_t* _info, uint16_t _width, uint16_t _height, uint16_t _depth, uint8_t _numMips, bgfx_texture_format_t _format)
|
||||
BGFX_C_API void bgfx_calc_texture_size(bgfx_texture_info_t* _info, uint16_t _width, uint16_t _height, uint16_t _depth, bool _cubeMap, uint8_t _numMips, bgfx_texture_format_t _format)
|
||||
{
|
||||
bgfx::TextureInfo& info = *(bgfx::TextureInfo*)_info;
|
||||
bgfx::calcTextureSize(info, _width, _height, _depth, _numMips, bgfx::TextureFormat::Enum(_format) );
|
||||
bgfx::calcTextureSize(info, _width, _height, _depth, _cubeMap, _numMips, bgfx::TextureFormat::Enum(_format) );
|
||||
}
|
||||
|
||||
BGFX_C_API bgfx_texture_handle_t bgfx_create_texture(const bgfx_memory_t* _mem, uint32_t _flags, uint8_t _skip, bgfx_texture_info_t* _info)
|
||||
|
31
3rdparty/bgfx/src/bgfx_compute.sh
vendored
31
3rdparty/bgfx/src/bgfx_compute.sh
vendored
@ -20,6 +20,10 @@ uint floatBitsToUint(float _x) { return asuint(_x); }
|
||||
uvec2 floatBitsToUint(vec2 _x) { return asuint(_x); }
|
||||
uvec3 floatBitsToUint(vec3 _x) { return asuint(_x); }
|
||||
uvec4 floatBitsToUint(vec4 _x) { return asuint(_x); }
|
||||
int floatBitsToInt(float _x) { return asint(_x); }
|
||||
ivec2 floatBitsToInt(vec2 _x) { return asint(_x); }
|
||||
ivec3 floatBitsToInt(vec3 _x) { return asint(_x); }
|
||||
ivec4 floatBitsToInt(vec4 _x) { return asint(_x); }
|
||||
|
||||
#define SHARED groupshared
|
||||
|
||||
@ -38,6 +42,16 @@ vec4 imageLoad(Texture2D _image, ivec2 _uv)
|
||||
return _image.Load(uint3(_uv.xy, 0) );
|
||||
}
|
||||
|
||||
uint imageLoad(Texture2D<uint> _image, ivec2 _uv)
|
||||
{
|
||||
return _image.Load(uint3(_uv.xy, 0) );
|
||||
}
|
||||
|
||||
uint imageLoad(RWTexture2D<uint> _image, ivec2 _uv)
|
||||
{
|
||||
return _image[_uv.xy];
|
||||
}
|
||||
|
||||
ivec2 imageSize(Texture2D _image)
|
||||
{
|
||||
ivec2 result;
|
||||
@ -45,11 +59,6 @@ ivec2 imageSize(Texture2D _image)
|
||||
return result;
|
||||
}
|
||||
|
||||
//vec4 imageLoad(RWTexture2D<float4> _image, ivec2 _uv)
|
||||
//{
|
||||
// return _image[_uv];
|
||||
//}
|
||||
|
||||
ivec2 imageSize(RWTexture2D<float4> _image)
|
||||
{
|
||||
ivec2 result;
|
||||
@ -57,11 +66,23 @@ ivec2 imageSize(RWTexture2D<float4> _image)
|
||||
return result;
|
||||
}
|
||||
|
||||
ivec2 imageSize(RWTexture2D<uint> _image)
|
||||
{
|
||||
ivec2 result;
|
||||
_image.GetDimensions(result.x, result.y);
|
||||
return result;
|
||||
}
|
||||
|
||||
void imageStore(RWTexture2D<float4> _image, ivec2 _uv, vec4 _rgba)
|
||||
{
|
||||
_image[_uv] = _rgba;
|
||||
}
|
||||
|
||||
void imageStore(RWTexture2D<uint> _image, ivec2 _uv, uvec4 _r)
|
||||
{
|
||||
_image[_uv] = _r.x;
|
||||
}
|
||||
|
||||
#define __ATOMIC_IMPL_TYPE(_genType, _glFunc, _dxFunc) \
|
||||
_genType _glFunc(_genType _mem, _genType _data) \
|
||||
{ \
|
||||
|
12
3rdparty/bgfx/src/bgfx_p.h
vendored
12
3rdparty/bgfx/src/bgfx_p.h
vendored
@ -201,8 +201,8 @@ namespace bgfx
|
||||
#elif BX_PLATFORM_IOS
|
||||
extern void* g_bgfxEaglLayer;
|
||||
#elif BX_PLATFORM_LINUX
|
||||
extern ::Display* g_bgfxX11Display;
|
||||
extern ::Window g_bgfxX11Window;
|
||||
extern void* g_bgfxX11Display;
|
||||
extern uint32_t g_bgfxX11Window;
|
||||
#elif BX_PLATFORM_OSX
|
||||
extern void* g_bgfxNSWindow;
|
||||
#elif BX_PLATFORM_WINDOWS
|
||||
@ -2681,6 +2681,7 @@ namespace bgfx
|
||||
, (uint16_t)imageContainer.m_width
|
||||
, (uint16_t)imageContainer.m_height
|
||||
, (uint16_t)imageContainer.m_depth
|
||||
, imageContainer.m_cubeMap
|
||||
, imageContainer.m_numMips
|
||||
, TextureFormat::Enum(imageContainer.m_format)
|
||||
);
|
||||
@ -2689,11 +2690,12 @@ namespace bgfx
|
||||
{
|
||||
_info->format = TextureFormat::Unknown;
|
||||
_info->storageSize = 0;
|
||||
_info->width = 0;
|
||||
_info->height = 0;
|
||||
_info->depth = 0;
|
||||
_info->width = 0;
|
||||
_info->height = 0;
|
||||
_info->depth = 0;
|
||||
_info->numMips = 0;
|
||||
_info->bitsPerPixel = 0;
|
||||
_info->cubeMap = false;
|
||||
}
|
||||
}
|
||||
|
||||
|
4
3rdparty/bgfx/src/glcontext_egl.cpp
vendored
4
3rdparty/bgfx/src/glcontext_egl.cpp
vendored
@ -185,8 +185,8 @@ EGL_IMPORT
|
||||
ndt = GetDC(g_bgfxHwnd);
|
||||
nwh = g_bgfxHwnd;
|
||||
# elif BX_PLATFORM_LINUX
|
||||
ndt = g_bgfxX11Display;
|
||||
nwh = g_bgfxX11Window;
|
||||
ndt = (EGLNativeDisplayType)g_bgfxX11Display;
|
||||
nwh = (EGLNativeWindowType)g_bgfxX11Window;
|
||||
# endif // BX_PLATFORM_
|
||||
m_display = eglGetDisplay(ndt);
|
||||
BGFX_FATAL(m_display != EGL_NO_DISPLAY, Fatal::UnableToInitialize, "Failed to create display %p", m_display);
|
||||
|
50
3rdparty/bgfx/src/glcontext_glx.cpp
vendored
50
3rdparty/bgfx/src/glcontext_glx.cpp
vendored
@ -29,23 +29,23 @@ namespace bgfx
|
||||
SwapChainGL(::Window _window, XVisualInfo* _visualInfo, GLXContext _context)
|
||||
: m_window(_window)
|
||||
{
|
||||
m_context = glXCreateContext(g_bgfxX11Display, _visualInfo, _context, GL_TRUE);
|
||||
m_context = glXCreateContext( (::Display*)g_bgfxX11Display, _visualInfo, _context, GL_TRUE);
|
||||
}
|
||||
|
||||
~SwapChainGL()
|
||||
{
|
||||
glXMakeCurrent(g_bgfxX11Display, 0, 0);
|
||||
glXDestroyContext(g_bgfxX11Display, m_context);
|
||||
glXMakeCurrent( (::Display*)g_bgfxX11Display, 0, 0);
|
||||
glXDestroyContext( (::Display*)g_bgfxX11Display, m_context);
|
||||
}
|
||||
|
||||
void makeCurrent()
|
||||
{
|
||||
glXMakeCurrent(g_bgfxX11Display, m_window, m_context);
|
||||
glXMakeCurrent( (::Display*)g_bgfxX11Display, m_window, m_context);
|
||||
}
|
||||
|
||||
void swapBuffers()
|
||||
{
|
||||
glXSwapBuffers(g_bgfxX11Display, m_window);
|
||||
glXSwapBuffers( (::Display*)g_bgfxX11Display, m_window);
|
||||
}
|
||||
|
||||
Window m_window;
|
||||
@ -55,10 +55,10 @@ namespace bgfx
|
||||
void GlContext::create(uint32_t _width, uint32_t _height)
|
||||
{
|
||||
BX_UNUSED(_width, _height);
|
||||
XLockDisplay(g_bgfxX11Display);
|
||||
XLockDisplay( (::Display*)g_bgfxX11Display);
|
||||
|
||||
int major, minor;
|
||||
bool version = glXQueryVersion(g_bgfxX11Display, &major, &minor);
|
||||
bool version = glXQueryVersion( (::Display*)g_bgfxX11Display, &major, &minor);
|
||||
BGFX_FATAL(version, Fatal::UnableToInitialize, "Failed to query GLX version");
|
||||
BGFX_FATAL( (major == 1 && minor >= 2) || major > 1
|
||||
, Fatal::UnableToInitialize
|
||||
@ -67,9 +67,9 @@ namespace bgfx
|
||||
, minor
|
||||
);
|
||||
|
||||
int32_t screen = DefaultScreen(g_bgfxX11Display);
|
||||
int32_t screen = DefaultScreen( (::Display*)g_bgfxX11Display);
|
||||
|
||||
const char* extensions = glXQueryExtensionsString(g_bgfxX11Display, screen);
|
||||
const char* extensions = glXQueryExtensionsString( (::Display*)g_bgfxX11Display, screen);
|
||||
BX_TRACE("GLX extensions:");
|
||||
dumpExtensions(extensions);
|
||||
|
||||
@ -91,13 +91,13 @@ namespace bgfx
|
||||
GLXFBConfig bestConfig = NULL;
|
||||
|
||||
int numConfigs;
|
||||
GLXFBConfig* configs = glXChooseFBConfig(g_bgfxX11Display, screen, attrsGlx, &numConfigs);
|
||||
GLXFBConfig* configs = glXChooseFBConfig( (::Display*)g_bgfxX11Display, screen, attrsGlx, &numConfigs);
|
||||
|
||||
BX_TRACE("glX num configs %d", numConfigs);
|
||||
|
||||
for (int ii = 0; ii < numConfigs; ++ii)
|
||||
{
|
||||
m_visualInfo = glXGetVisualFromFBConfig(g_bgfxX11Display, configs[ii]);
|
||||
m_visualInfo = glXGetVisualFromFBConfig( (::Display*)g_bgfxX11Display, configs[ii]);
|
||||
if (NULL != m_visualInfo)
|
||||
{
|
||||
BX_TRACE("---");
|
||||
@ -105,7 +105,7 @@ namespace bgfx
|
||||
for (uint32_t attr = 6; attr < BX_COUNTOF(attrsGlx)-1 && attrsGlx[attr] != None; attr += 2)
|
||||
{
|
||||
int value;
|
||||
glXGetFBConfigAttrib(g_bgfxX11Display, configs[ii], attrsGlx[attr], &value);
|
||||
glXGetFBConfigAttrib( (::Display*)g_bgfxX11Display, configs[ii], attrsGlx[attr], &value);
|
||||
BX_TRACE("glX %d/%d %2d: %4x, %8x (%8x%s)"
|
||||
, ii
|
||||
, numConfigs
|
||||
@ -141,7 +141,7 @@ namespace bgfx
|
||||
BGFX_FATAL(m_visualInfo, Fatal::UnableToInitialize, "Failed to find a suitable X11 display configuration.");
|
||||
|
||||
BX_TRACE("Create GL 2.1 context.");
|
||||
m_context = glXCreateContext(g_bgfxX11Display, m_visualInfo, 0, GL_TRUE);
|
||||
m_context = glXCreateContext( (::Display*)g_bgfxX11Display, m_visualInfo, 0, GL_TRUE);
|
||||
BGFX_FATAL(NULL != m_context, Fatal::UnableToInitialize, "Failed to create GL 2.1 context.");
|
||||
|
||||
#if BGFX_CONFIG_RENDERER_OPENGL >= 31
|
||||
@ -158,11 +158,11 @@ namespace bgfx
|
||||
0,
|
||||
};
|
||||
|
||||
GLXContext context = glXCreateContextAttribsARB(g_bgfxX11Display, bestConfig, 0, true, contextAttrs);
|
||||
GLXContext context = glXCreateContextAttribsARB( (::Display*)g_bgfxX11Display, bestConfig, 0, true, contextAttrs);
|
||||
|
||||
if (NULL != context)
|
||||
{
|
||||
glXDestroyContext(g_bgfxX11Display, m_context);
|
||||
glXDestroyContext( (::Display*)g_bgfxX11Display, m_context);
|
||||
m_context = context;
|
||||
}
|
||||
}
|
||||
@ -170,17 +170,17 @@ namespace bgfx
|
||||
BX_UNUSED(bestConfig);
|
||||
#endif // BGFX_CONFIG_RENDERER_OPENGL >= 31
|
||||
|
||||
XUnlockDisplay(g_bgfxX11Display);
|
||||
XUnlockDisplay( (::Display*)g_bgfxX11Display);
|
||||
|
||||
import();
|
||||
|
||||
glXMakeCurrent(g_bgfxX11Display, g_bgfxX11Window, m_context);
|
||||
glXMakeCurrent( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window, m_context);
|
||||
|
||||
glXSwapIntervalEXT = (PFNGLXSWAPINTERVALEXTPROC)glXGetProcAddress( (const GLubyte*)"glXSwapIntervalEXT");
|
||||
if (NULL != glXSwapIntervalEXT)
|
||||
{
|
||||
BX_TRACE("Using glXSwapIntervalEXT.");
|
||||
glXSwapIntervalEXT(g_bgfxX11Display, g_bgfxX11Window, 0);
|
||||
glXSwapIntervalEXT( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window, 0);
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -203,13 +203,13 @@ namespace bgfx
|
||||
|
||||
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
|
||||
glClear(GL_COLOR_BUFFER_BIT);
|
||||
glXSwapBuffers(g_bgfxX11Display, g_bgfxX11Window);
|
||||
glXSwapBuffers( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window);
|
||||
}
|
||||
|
||||
void GlContext::destroy()
|
||||
{
|
||||
glXMakeCurrent(g_bgfxX11Display, 0, 0);
|
||||
glXDestroyContext(g_bgfxX11Display, m_context);
|
||||
glXMakeCurrent( (::Display*)g_bgfxX11Display, 0, 0);
|
||||
glXDestroyContext( (::Display*)g_bgfxX11Display, m_context);
|
||||
XFree(m_visualInfo);
|
||||
}
|
||||
|
||||
@ -219,7 +219,7 @@ namespace bgfx
|
||||
|
||||
if (NULL != glXSwapIntervalEXT)
|
||||
{
|
||||
glXSwapIntervalEXT(g_bgfxX11Display, g_bgfxX11Window, interval);
|
||||
glXSwapIntervalEXT( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window, interval);
|
||||
}
|
||||
else if (NULL != glXSwapIntervalMESA)
|
||||
{
|
||||
@ -250,8 +250,8 @@ namespace bgfx
|
||||
{
|
||||
if (NULL == _swapChain)
|
||||
{
|
||||
glXMakeCurrent(g_bgfxX11Display, g_bgfxX11Window, m_context);
|
||||
glXSwapBuffers(g_bgfxX11Display, g_bgfxX11Window);
|
||||
glXMakeCurrent( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window, m_context);
|
||||
glXSwapBuffers( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window);
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -264,7 +264,7 @@ namespace bgfx
|
||||
{
|
||||
if (NULL == _swapChain)
|
||||
{
|
||||
glXMakeCurrent(g_bgfxX11Display, g_bgfxX11Window, m_context);
|
||||
glXMakeCurrent( (::Display*)g_bgfxX11Display, (::Window)g_bgfxX11Window, m_context);
|
||||
}
|
||||
else
|
||||
{
|
||||
|
2
3rdparty/bgfx/src/glimports.h
vendored
2
3rdparty/bgfx/src/glimports.h
vendored
@ -230,7 +230,7 @@ GL_IMPORT______(false, PFNGLBUFFERDATAPROC, glBufferData)
|
||||
GL_IMPORT______(false, PFNGLBUFFERSUBDATAPROC, glBufferSubData);
|
||||
GL_IMPORT______(true, PFNGLCHECKFRAMEBUFFERSTATUSPROC, glCheckFramebufferStatus);
|
||||
GL_IMPORT______(false, PFNGLCLEARPROC, glClear);
|
||||
GL_IMPORT______(false, PFNGLCLEARBUFFERFVPROC, glClearBufferfv);
|
||||
GL_IMPORT______(true, PFNGLCLEARBUFFERFVPROC, glClearBufferfv);
|
||||
GL_IMPORT______(false, PFNGLCLEARCOLORPROC, glClearColor);
|
||||
GL_IMPORT______(false, PFNGLCLEARSTENCILPROC, glClearStencil);
|
||||
GL_IMPORT______(false, PFNGLCOLORMASKPROC, glColorMask);
|
||||
|
174
3rdparty/bgfx/src/image.cpp
vendored
174
3rdparty/bgfx/src/image.cpp
vendored
@ -12,54 +12,61 @@ namespace bgfx
|
||||
{
|
||||
static const ImageBlockInfo s_imageBlockInfo[] =
|
||||
{
|
||||
{ 4, 4, 4, 8 }, // BC1
|
||||
{ 8, 4, 4, 16 }, // BC2
|
||||
{ 8, 4, 4, 16 }, // BC3
|
||||
{ 4, 4, 4, 8 }, // BC4
|
||||
{ 8, 4, 4, 16 }, // BC5
|
||||
{ 8, 4, 4, 16 }, // BC6H
|
||||
{ 8, 4, 4, 16 }, // BC7
|
||||
{ 4, 4, 4, 8 }, // ETC1
|
||||
{ 4, 4, 4, 8 }, // ETC2
|
||||
{ 8, 4, 4, 16 }, // ETC2A
|
||||
{ 4, 4, 4, 8 }, // ETC2A1
|
||||
{ 2, 8, 4, 8 }, // PTC12
|
||||
{ 4, 4, 4, 8 }, // PTC14
|
||||
{ 2, 8, 4, 8 }, // PTC12A
|
||||
{ 4, 4, 4, 8 }, // PTC14A
|
||||
{ 2, 8, 4, 8 }, // PTC22
|
||||
{ 4, 4, 4, 8 }, // PTC24
|
||||
{ 0, 0, 0, 0 }, // Unknown
|
||||
{ 1, 8, 1, 1 }, // R1
|
||||
{ 8, 1, 1, 1 }, // R8
|
||||
{ 16, 1, 1, 2 }, // R16
|
||||
{ 16, 1, 1, 2 }, // R16F
|
||||
{ 32, 1, 1, 4 }, // R32
|
||||
{ 32, 1, 1, 4 }, // R32F
|
||||
{ 16, 1, 1, 2 }, // RG8
|
||||
{ 32, 1, 1, 4 }, // RG16
|
||||
{ 32, 1, 1, 4 }, // RG16F
|
||||
{ 64, 1, 1, 8 }, // RG32
|
||||
{ 64, 1, 1, 8 }, // RG32F
|
||||
{ 32, 1, 1, 4 }, // BGRA8
|
||||
{ 64, 1, 1, 8 }, // RGBA16
|
||||
{ 64, 1, 1, 8 }, // RGBA16F
|
||||
{ 128, 1, 1, 16 }, // RGBA32
|
||||
{ 128, 1, 1, 16 }, // RGBA32F
|
||||
{ 16, 1, 1, 2 }, // R5G6B5
|
||||
{ 16, 1, 1, 2 }, // RGBA4
|
||||
{ 16, 1, 1, 2 }, // RGB5A1
|
||||
{ 32, 1, 1, 4 }, // RGB10A2
|
||||
{ 32, 1, 1, 4 }, // R11G11B10F
|
||||
{ 0, 0, 0, 0 }, // UnknownDepth
|
||||
{ 16, 1, 1, 2 }, // D16
|
||||
{ 24, 1, 1, 3 }, // D24
|
||||
{ 32, 1, 1, 4 }, // D24S8
|
||||
{ 32, 1, 1, 4 }, // D32
|
||||
{ 16, 1, 1, 2 }, // D16F
|
||||
{ 24, 1, 1, 3 }, // D24F
|
||||
{ 32, 1, 1, 4 }, // D32F
|
||||
{ 8, 1, 1, 1 }, // D0S8
|
||||
// +------------------ bits per pixel
|
||||
// | +--------------- block width
|
||||
// | | +------------ block height
|
||||
// | | | +-------- block size
|
||||
// | | | | +----- min blocks x
|
||||
// | | | | | +-- min blocks y
|
||||
// | | | | | |
|
||||
{ 4, 4, 4, 8, 1, 1 }, // BC1
|
||||
{ 8, 4, 4, 16, 1, 1 }, // BC2
|
||||
{ 8, 4, 4, 16, 1, 1 }, // BC3
|
||||
{ 4, 4, 4, 8, 1, 1 }, // BC4
|
||||
{ 8, 4, 4, 16, 1, 1 }, // BC5
|
||||
{ 8, 4, 4, 16, 1, 1 }, // BC6H
|
||||
{ 8, 4, 4, 16, 1, 1 }, // BC7
|
||||
{ 4, 4, 4, 8, 1, 1 }, // ETC1
|
||||
{ 4, 4, 4, 8, 1, 1 }, // ETC2
|
||||
{ 8, 4, 4, 16, 1, 1 }, // ETC2A
|
||||
{ 4, 4, 4, 8, 1, 1 }, // ETC2A1
|
||||
{ 2, 8, 4, 8, 2, 2 }, // PTC12
|
||||
{ 4, 4, 4, 8, 2, 2 }, // PTC14
|
||||
{ 2, 8, 4, 8, 2, 2 }, // PTC12A
|
||||
{ 4, 4, 4, 8, 2, 2 }, // PTC14A
|
||||
{ 2, 8, 4, 8, 2, 2 }, // PTC22
|
||||
{ 4, 4, 4, 8, 2, 2 }, // PTC24
|
||||
{ 0, 0, 0, 0, 1, 1 }, // Unknown
|
||||
{ 1, 8, 1, 1, 1, 1 }, // R1
|
||||
{ 8, 1, 1, 1, 1, 1 }, // R8
|
||||
{ 16, 1, 1, 2, 1, 1 }, // R16
|
||||
{ 16, 1, 1, 2, 1, 1 }, // R16F
|
||||
{ 32, 1, 1, 4, 1, 1 }, // R32
|
||||
{ 32, 1, 1, 4, 1, 1 }, // R32F
|
||||
{ 16, 1, 1, 2, 1, 1 }, // RG8
|
||||
{ 32, 1, 1, 4, 1, 1 }, // RG16
|
||||
{ 32, 1, 1, 4, 1, 1 }, // RG16F
|
||||
{ 64, 1, 1, 8, 1, 1 }, // RG32
|
||||
{ 64, 1, 1, 8, 1, 1 }, // RG32F
|
||||
{ 32, 1, 1, 4, 1, 1 }, // BGRA8
|
||||
{ 64, 1, 1, 8, 1, 1 }, // RGBA16
|
||||
{ 64, 1, 1, 8, 1, 1 }, // RGBA16F
|
||||
{ 128, 1, 1, 16, 1, 1 }, // RGBA32
|
||||
{ 128, 1, 1, 16, 1, 1 }, // RGBA32F
|
||||
{ 16, 1, 1, 2, 1, 1 }, // R5G6B5
|
||||
{ 16, 1, 1, 2, 1, 1 }, // RGBA4
|
||||
{ 16, 1, 1, 2, 1, 1 }, // RGB5A1
|
||||
{ 32, 1, 1, 4, 1, 1 }, // RGB10A2
|
||||
{ 32, 1, 1, 4, 1, 1 }, // R11G11B10F
|
||||
{ 0, 0, 0, 0, 1, 1 }, // UnknownDepth
|
||||
{ 16, 1, 1, 2, 1, 1 }, // D16
|
||||
{ 24, 1, 1, 3, 1, 1 }, // D24
|
||||
{ 32, 1, 1, 4, 1, 1 }, // D24S8
|
||||
{ 32, 1, 1, 4, 1, 1 }, // D32
|
||||
{ 16, 1, 1, 2, 1, 1 }, // D16F
|
||||
{ 24, 1, 1, 3, 1, 1 }, // D24F
|
||||
{ 32, 1, 1, 4, 1, 1 }, // D32F
|
||||
{ 8, 1, 1, 1, 1, 1 }, // D0S8
|
||||
};
|
||||
BX_STATIC_ASSERT(TextureFormat::Count == BX_COUNTOF(s_imageBlockInfo) );
|
||||
|
||||
@ -1309,10 +1316,9 @@ namespace bgfx
|
||||
{
|
||||
uint32_t m_format;
|
||||
TextureFormat::Enum m_textureFormat;
|
||||
|
||||
};
|
||||
|
||||
static TranslateDdsFormat s_translateDdsFormat[] =
|
||||
static TranslateDdsFormat s_translateDdsFourccFormat[] =
|
||||
{
|
||||
{ DDS_DXT1, TextureFormat::BC1 },
|
||||
{ DDS_DXT2, TextureFormat::BC2 },
|
||||
@ -1376,6 +1382,22 @@ namespace bgfx
|
||||
{ DXGI_FORMAT_R10G10B10A2_UNORM, TextureFormat::RGB10A2 },
|
||||
};
|
||||
|
||||
struct TranslateDdsPixelFormat
|
||||
{
|
||||
uint32_t m_bitCount;
|
||||
uint32_t m_bitmask[4];
|
||||
TextureFormat::Enum m_textureFormat;
|
||||
};
|
||||
|
||||
static TranslateDdsPixelFormat s_translateDdsPixelFormat[] =
|
||||
{
|
||||
{ 32, { 0x00ff0000, 0x0000ff00, 0x000000ff, 0xff000000 }, TextureFormat::BGRA8 },
|
||||
{ 32, { 0x00ff0000, 0x0000ff00, 0x000000ff, 0x00000000 }, TextureFormat::BGRA8 },
|
||||
{ 32, { 0x000003ff, 0x000ffc00, 0x3ff00000, 0xc0000000 }, TextureFormat::RGB10A2 },
|
||||
{ 32, { 0x0000ffff, 0xffff0000, 0x00000000, 0x00000000 }, TextureFormat::RG16 },
|
||||
{ 32, { 0xffffffff, 0x00000000, 0x00000000, 0x00000000 }, TextureFormat::R32 },
|
||||
};
|
||||
|
||||
bool imageParseDds(ImageContainer& _imageContainer, bx::ReaderSeekerI* _reader)
|
||||
{
|
||||
uint32_t headerSize;
|
||||
@ -1420,20 +1442,11 @@ namespace bgfx
|
||||
uint32_t fourcc;
|
||||
bx::read(_reader, fourcc);
|
||||
|
||||
uint32_t rgbCount;
|
||||
bx::read(_reader, rgbCount);
|
||||
uint32_t bitCount;
|
||||
bx::read(_reader, bitCount);
|
||||
|
||||
uint32_t rbitmask;
|
||||
bx::read(_reader, rbitmask);
|
||||
|
||||
uint32_t gbitmask;
|
||||
bx::read(_reader, gbitmask);
|
||||
|
||||
uint32_t bbitmask;
|
||||
bx::read(_reader, bbitmask);
|
||||
|
||||
uint32_t abitmask;
|
||||
bx::read(_reader, abitmask);
|
||||
uint32_t bitmask[4];
|
||||
bx::read(_reader, bitmask, sizeof(bitmask) );
|
||||
|
||||
uint32_t caps[4];
|
||||
bx::read(_reader, caps);
|
||||
@ -1479,14 +1492,31 @@ namespace bgfx
|
||||
|
||||
if (dxgiFormat == 0)
|
||||
{
|
||||
uint32_t ddsFormat = pixelFlags & DDPF_FOURCC ? fourcc : pixelFlags;
|
||||
|
||||
for (uint32_t ii = 0; ii < BX_COUNTOF(s_translateDdsFormat); ++ii)
|
||||
if (DDPF_FOURCC == (pixelFlags & DDPF_FOURCC) )
|
||||
{
|
||||
if (s_translateDdsFormat[ii].m_format == ddsFormat)
|
||||
for (uint32_t ii = 0; ii < BX_COUNTOF(s_translateDdsFourccFormat); ++ii)
|
||||
{
|
||||
format = s_translateDdsFormat[ii].m_textureFormat;
|
||||
break;
|
||||
if (s_translateDdsFourccFormat[ii].m_format == fourcc)
|
||||
{
|
||||
format = s_translateDdsFourccFormat[ii].m_textureFormat;
|
||||
break;
|
||||
}
|
||||
}
|
||||
}
|
||||
else
|
||||
{
|
||||
for (uint32_t ii = 0; ii < BX_COUNTOF(s_translateDdsPixelFormat); ++ii)
|
||||
{
|
||||
const TranslateDdsPixelFormat& pf = s_translateDdsPixelFormat[ii];
|
||||
if (pf.m_bitCount == bitCount
|
||||
&& pf.m_bitmask[0] == bitmask[0]
|
||||
&& pf.m_bitmask[1] == bitmask[1]
|
||||
&& pf.m_bitmask[2] == bitmask[2]
|
||||
&& pf.m_bitmask[3] == bitmask[3])
|
||||
{
|
||||
format = pf.m_textureFormat;
|
||||
break;
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
@ -2103,6 +2133,8 @@ namespace bgfx
|
||||
const uint32_t blockSize = blockInfo.blockSize;
|
||||
const uint32_t blockWidth = blockInfo.blockWidth;
|
||||
const uint32_t blockHeight = blockInfo.blockHeight;
|
||||
const uint32_t minBlockX = blockInfo.minBlockX;
|
||||
const uint32_t minBlockY = blockInfo.minBlockY;
|
||||
|
||||
if (UINT32_MAX == _imageContainer.m_offset)
|
||||
{
|
||||
@ -2127,8 +2159,8 @@ namespace bgfx
|
||||
// skip imageSize in KTX format.
|
||||
offset += _imageContainer.m_ktx ? sizeof(uint32_t) : 0;
|
||||
|
||||
width = bx::uint32_max(blockWidth, ( (width +blockWidth -1)/blockWidth )*blockWidth);
|
||||
height = bx::uint32_max(blockHeight, ( (height+blockHeight-1)/blockHeight)*blockHeight);
|
||||
width = bx::uint32_max(blockWidth * minBlockX, ( (width + blockWidth - 1) / blockWidth )*blockWidth);
|
||||
height = bx::uint32_max(blockHeight * minBlockY, ( (height + blockHeight - 1) / blockHeight)*blockHeight);
|
||||
depth = bx::uint32_max(1, depth);
|
||||
|
||||
uint32_t size = width*height*depth*bpp/8;
|
||||
|
2
3rdparty/bgfx/src/image.h
vendored
2
3rdparty/bgfx/src/image.h
vendored
@ -43,6 +43,8 @@ namespace bgfx
|
||||
uint8_t blockWidth;
|
||||
uint8_t blockHeight;
|
||||
uint8_t blockSize;
|
||||
uint8_t minBlockX;
|
||||
uint8_t minBlockY;
|
||||
};
|
||||
|
||||
///
|
||||
|
8
3rdparty/bgfx/src/renderer_d3d11.cpp
vendored
8
3rdparty/bgfx/src/renderer_d3d11.cpp
vendored
@ -345,7 +345,7 @@ namespace bgfx
|
||||
static const GUID IID_IDXGIDevice2 = { 0x05008617, 0xfbfd, 0x4051, { 0xa7, 0x90, 0x14, 0x48, 0x84, 0xb4, 0xf6, 0xa9 } };
|
||||
static const GUID IID_IDXGIDevice3 = { 0x6007896c, 0x3244, 0x4afd, { 0xbf, 0x18, 0xa6, 0xd3, 0xbe, 0xda, 0x50, 0x23 } };
|
||||
static const GUID IID_IDXGIAdapter = { 0x2411e7e1, 0x12ac, 0x4ccf, { 0xbd, 0x14, 0x97, 0x98, 0xe8, 0x53, 0x4d, 0xc0 } };
|
||||
static const GUID IID_ID3D11InfoQueue = { 0x6543dbb6, 0x1b48, 0x42f5, { 0xab, 0x82, 0xe9, 0x7e, 0xc7, 0x43, 0x26, 0xf6 } };
|
||||
static const GUID IID_ID3D11InfoQueue = { 0x6543dbb6, 0x1b48, 0x42f5, { 0xab, 0x82, 0xe9, 0x7e, 0xc7, 0x43, 0x26, 0xf6 } };
|
||||
|
||||
static const GUID s_deviceIIDs[] =
|
||||
{
|
||||
@ -2365,10 +2365,8 @@ namespace bgfx
|
||||
BX_CHECK(m_dynamic, "Must be dynamic!");
|
||||
|
||||
D3D11_MAPPED_SUBRESOURCE mapped;
|
||||
D3D11_MAP type = m_dynamic && ( (0 == _offset && m_size == _size) || _discard)
|
||||
? D3D11_MAP_WRITE_DISCARD
|
||||
: D3D11_MAP_WRITE_NO_OVERWRITE
|
||||
;
|
||||
BX_UNUSED(_discard);
|
||||
D3D11_MAP type = D3D11_MAP_WRITE_DISCARD;
|
||||
DX_CHECK(deviceCtx->Map(m_ptr, 0, type, 0, &mapped));
|
||||
memcpy((uint8_t*)mapped.pData + _offset, _data, _size);
|
||||
deviceCtx->Unmap(m_ptr, 0);
|
||||
|
6
3rdparty/bgfx/src/renderer_d3d12.cpp
vendored
6
3rdparty/bgfx/src/renderer_d3d12.cpp
vendored
@ -5,6 +5,10 @@
|
||||
|
||||
#include "bgfx_p.h"
|
||||
|
||||
#if BGFX_CONFIG_RENDERER_DIRECT3D12
|
||||
# include "../../d3d12/src/renderer_d3d12.cpp"
|
||||
#else
|
||||
|
||||
namespace bgfx
|
||||
{
|
||||
RendererContextI* rendererCreateD3D12()
|
||||
@ -16,3 +20,5 @@ namespace bgfx
|
||||
{
|
||||
}
|
||||
} // namespace bgfx
|
||||
|
||||
#endif // BGFX_CONFIG_RENDERER_DIRECT3D12
|
||||
|
13
3rdparty/bgfx/src/renderer_gl.cpp
vendored
13
3rdparty/bgfx/src/renderer_gl.cpp
vendored
@ -3759,7 +3759,9 @@ namespace bgfx
|
||||
}
|
||||
}
|
||||
|
||||
writeString(&writer, "precision mediump float;\n");
|
||||
writeStringf(&writer, "precision %s float;\n"
|
||||
, m_type == GL_FRAGMENT_SHADER ? "mediump" : "highp"
|
||||
);
|
||||
|
||||
bx::write(&writer, code, codeLen);
|
||||
bx::write(&writer, '\0');
|
||||
@ -4785,11 +4787,12 @@ namespace bgfx
|
||||
&& 0 == draw.m_instanceDataOffset)
|
||||
{
|
||||
if (programChanged
|
||||
|| currentState.m_vertexBuffer.idx != draw.m_vertexBuffer.idx
|
||||
|| currentState.m_indexBuffer.idx != draw.m_indexBuffer.idx
|
||||
|| currentState.m_instanceDataBuffer.idx != draw.m_instanceDataBuffer.idx
|
||||
|| baseVertex != draw.m_startVertex
|
||||
|| currentState.m_vertexBuffer.idx != draw.m_vertexBuffer.idx
|
||||
|| currentState.m_indexBuffer.idx != draw.m_indexBuffer.idx
|
||||
|| currentState.m_instanceDataOffset != draw.m_instanceDataOffset
|
||||
|| currentState.m_instanceDataStride != draw.m_instanceDataStride)
|
||||
|| currentState.m_instanceDataStride != draw.m_instanceDataStride
|
||||
|| currentState.m_instanceDataBuffer.idx != draw.m_instanceDataBuffer.idx)
|
||||
{
|
||||
bx::HashMurmur2A murmur;
|
||||
murmur.begin();
|
||||
|
BIN
3rdparty/bx/tools/bin/darwin/genie
vendored
BIN
3rdparty/bx/tools/bin/darwin/genie
vendored
Binary file not shown.
BIN
3rdparty/bx/tools/bin/linux/genie
vendored
BIN
3rdparty/bx/tools/bin/linux/genie
vendored
Binary file not shown.
BIN
3rdparty/bx/tools/bin/windows/genie.exe
vendored
BIN
3rdparty/bx/tools/bin/windows/genie.exe
vendored
Binary file not shown.
2
3rdparty/genie/README.md
vendored
2
3rdparty/genie/README.md
vendored
@ -14,7 +14,7 @@ Supported project generators:
|
||||
Download (stable)
|
||||
-----------------
|
||||
|
||||
version 172 (commit d5a8dc1e36d7120ad4b91bbc1d662c543aa8ba8d)
|
||||
version 178 (commit f9ef54d6a5fa3421d141b8fe53683fe1a0fbd9cc)
|
||||
|
||||
Linux:
|
||||
https://github.com/bkaradzic/bx/raw/master/tools/bin/linux/genie
|
||||
|
@ -134,8 +134,8 @@ OBJECTS := \
|
||||
|
||||
OBJDIRS := \
|
||||
$(OBJDIR) \
|
||||
$(OBJDIR)/src/host/lua-5.2.3/src \
|
||||
$(OBJDIR)/src/host \
|
||||
$(OBJDIR)/src/host/lua-5.2.3/src \
|
||||
|
||||
RESOURCES := \
|
||||
|
||||
@ -156,8 +156,8 @@ $(TARGETDIR):
|
||||
$(OBJDIRS):
|
||||
@echo Creating $(OBJDIR)
|
||||
-$(call MKDIR,$(OBJDIR))
|
||||
-$(call MKDIR,$(OBJDIR)/src/host/lua-5.2.3/src)
|
||||
-$(call MKDIR,$(OBJDIR)/src/host)
|
||||
-$(call MKDIR,$(OBJDIR)/src/host/lua-5.2.3/src)
|
||||
|
||||
clean:
|
||||
@echo Cleaning genie
|
||||
|
36
3rdparty/genie/src/actions/make/make_cpp.lua
vendored
36
3rdparty/genie/src/actions/make/make_cpp.lua
vendored
@ -21,21 +21,10 @@
|
||||
|
||||
for _, platform in ipairs(platforms) do
|
||||
for cfg in premake.eachconfig(prj, platform) do
|
||||
premake.gmake_cpp_config(cfg, cc)
|
||||
premake.gmake_cpp_config(prj, cfg, cc)
|
||||
end
|
||||
end
|
||||
|
||||
-- list intermediate files
|
||||
_p('OBJECTS := \\')
|
||||
for _, file in ipairs(prj.files) do
|
||||
if path.iscppfile(file) then
|
||||
_p('\t$(OBJDIR)/%s.o \\'
|
||||
, _MAKE.esc(path.trimdots(path.removeext(file)))
|
||||
)
|
||||
end
|
||||
end
|
||||
_p('')
|
||||
|
||||
-- list object directories
|
||||
local objdirs = {}
|
||||
for _, file in ipairs(prj.files) do
|
||||
@ -228,7 +217,7 @@
|
||||
-- Write a block of configuration settings.
|
||||
--
|
||||
|
||||
function premake.gmake_cpp_config(cfg, cc)
|
||||
function premake.gmake_cpp_config(prj, cfg, cc)
|
||||
|
||||
_p('ifeq ($(config),%s)', _MAKE.esc(cfg.shortname))
|
||||
|
||||
@ -250,6 +239,27 @@
|
||||
-- write out libraries, linker flags, and the link command
|
||||
cpp.linker(cfg, cc)
|
||||
|
||||
-- add objects for compilation, and remove any that are excluded per config.
|
||||
_p(' OBJECTS := \\')
|
||||
for _, file in ipairs(prj.files) do
|
||||
if path.iscppfile(file) then
|
||||
-- check if file is excluded.
|
||||
local excluded = false
|
||||
for _, exclude in ipairs(cfg.excludes) do
|
||||
excluded = (exclude == file)
|
||||
if (excluded) then break end
|
||||
end
|
||||
|
||||
-- if not excluded, add it.
|
||||
if excluded == false then
|
||||
_p('\t$(OBJDIR)/%s.o \\'
|
||||
, _MAKE.esc(path.trimdots(path.removeext(file)))
|
||||
)
|
||||
end
|
||||
end
|
||||
end
|
||||
_p('')
|
||||
|
||||
_p(' define PREBUILDCMDS')
|
||||
if #cfg.prebuildcommands > 0 then
|
||||
_p('\t@echo Running pre-build commands')
|
||||
|
20
3rdparty/genie/src/host/scripts.c
vendored
20
3rdparty/genie/src/host/scripts.c
vendored
@ -179,16 +179,16 @@ const char* builtin_scripts[] = {
|
||||
"ative(sln.location, prj.location)), _MAKE.esc(_MAKE.getmakefilename(prj, true)))\n_p('')\nend\n_p('clean:')\nfor _ ,prj in ipairs(sln.projects) do\n_p('\\t@${MAKE} --no-print-directory -C %s -f %s clean', _MAKE.esc(path.getrelative(sln.location, prj.location)), _MAKE.esc(_MAKE.getmakefilename(prj, true)))\nend\n_p('')\n_p('help:')\n_p(1,'@echo \"Usage: make [config=name] [target]\"')\n_p(1,'@echo \"\"')\n_p(1,'@echo \"CONFIGURATIONS:\"')\nlocal cfgpairs = { }\nfor _, platform in ipairs(platforms) do\nfor _, cfgname in ipairs(sln.configurations) do\n_p(1,'@echo \" %s\"', premake.getconfigname(cfgname, platform, true))\nend\nend\n_p(1,'@echo \"\"')\n_p(1,'@echo \"TARGETS:\"')\n_p(1,'@echo \" all (default)\"')\n_p(1,'@echo \" clean\"')\nfor _, prj in ipairs(sln.projects) do\n_p(1,'@echo \" %s\"', prj.name)\nend\n_p(1,'@echo \"\"')\n_p(1,'@echo \"For more information, see http://industriousone.com/premake/quick-start\"')\nend\n",
|
||||
|
||||
/* actions/make/make_cpp.lua */
|
||||
"premake.make.cpp = { }\nlocal cpp = premake.make.cpp\nlocal make = premake.make\nfunction premake.make_cpp(prj)\nlocal cc = premake.gettool(prj)\nlocal platforms = premake.filterplatforms(prj.solution, cc.platforms, \"Native\")\npremake.gmake_cpp_header(prj, cc, platforms)\nfor _, platform in ipairs(platforms) do\nfor cfg in premake.eachconfig(prj, platform) do\npremake.gmake_cpp_config(cfg, cc)\nend\nend\n_p('OBJECTS := \\\\')\nfor _, file in ipairs(prj.files) do\nif path.iscppfile(file) then\n_p('\\t$(OBJDIR)/%s.o \\\\'\n, _MAKE.esc(path.trimdots(path.removeext(file)))\n)\nend\nend\n_p('')\nlocal objdirs = {}\nfor _, file in ipairs(prj.files) do\nif path.iscppfile(file) then\nobjdirs[_MAKE.esc(path.getdirectory(path.trimdots(file)))] = 1\nend\nend\n_p('OBJDIRS := \\\\')\n_p('\\t$(OBJDIR) \\\\')\nfor dir, _ in pairs(objdirs) do\n_p('\\t$(OBJDIR)/%s \\\\', dir)\nend\n_p('')\n_p('RESOURCES := \\\\')\nfor _, file in ipairs(prj.files) do\nif path.isresourcefile(file) then\n_p('\\t$(OBJDIR)/%s.res \\\\', _MAKE.esc"
|
||||
"(path.getbasename(file)))\nend\nend\n_p('')\n_p('.PHONY: clean prebuild prelink')\n_p('')\nif os.is(\"MacOSX\") and prj.kind == \"WindowedApp\" then\n_p('all: $(TARGETDIR) $(OBJDIRS) prebuild prelink $(TARGET) $(dir $(TARGETDIR))PkgInfo $(dir $(TARGETDIR))Info.plist')\nelse\n_p('all: $(TARGETDIR) $(OBJDIRS) prebuild prelink $(TARGET)')\nend\n_p('\\t@:')\n_p('')\nif (prj.kind == \"StaticLib\" and prj.options.ArchiveSplit) then\n_p('define max_args')\n_p('\\t$(eval _args:=)')\n_p('\\t$(foreach obj,$3,$(eval _args+=$(obj))$(if $(word $2,$(_args)),$1$(_args)$(EOL)$(eval _args:=)))')\n_p('\\t$(if $(_args),$1$(_args))')\n_p('endef')\n_p('')\n_p('define EOL')\n_p('')\n_p('')\n_p('endef')\n_p('')\nend\n_p('$(TARGET): $(GCH) $(OBJECTS) $(LDDEPS) $(RESOURCES)')\nif prj.kind == \"StaticLib\" then\nif prj.msgarchiving then\n_p('\\t@echo ' .. prj.msgarchiving)\nelse\n_p('\\t@echo Archiving %s', prj.name)\nend\nif (not prj.archivesplit_size) then \nprj.archivesplit_size=200\nend\nif (not prj.options.ArchiveSplit) then\n_p('"
|
||||
"\\t$(SILENT) $(LINKCMD) $(OBJECTS)')\nelse\n_p('\\t@$(call max_args,$(LINKCMD),'.. prj.archivesplit_size ..',$(OBJECTS))')\nend\nelse\nif prj.msglinking then\n_p('\\t@echo ' .. prj.msglinking)\nelse\n_p('\\t@echo Linking %s', prj.name)\nend\n_p('\\t$(SILENT) $(LINKCMD)')\nend\n_p('\\t$(POSTBUILDCMDS)')\n_p('')\n_p('$(TARGETDIR):')\npremake.make_mkdirrule(\"$(TARGETDIR)\")\n_p('$(OBJDIRS):')\nif (not prj.solution.messageskip) or (not table.contains(prj.solution.messageskip, \"SkipCreatingMessage\")) then\n_p('\\t@echo Creating $(OBJDIR)')\nend\n_p('\\t-$(call MKDIR,$(OBJDIR))')\nfor dir, _ in pairs(objdirs) do\n_p('\\t-$(call MKDIR,$(OBJDIR)/%s)', dir)\nend\n_p('')\nif os.is(\"MacOSX\") and prj.kind == \"WindowedApp\" then\n_p('$(dir $(TARGETDIR))PkgInfo:')\n_p('$(dir $(TARGETDIR))Info.plist:')\n_p('')\nend\n_p('clean:')\nif (not prj.solution.messageskip) or (not table.contains(prj.solution.messageskip, \"SkipCleaningMessage\")) then\n_p('\\t@echo Cleaning %s', prj.name)\nend\n_p('ifeq (posix,$(SHELLTYPE))')\n_"
|
||||
"p('\\t$(SILENT) rm -f $(TARGET)')\n_p('\\t$(SILENT) rm -rf $(OBJDIR)')\n_p('else')\n_p('\\t$(SILENT) if exist $(subst /,\\\\\\\\,$(TARGET)) del $(subst /,\\\\\\\\,$(TARGET))')\n_p('\\t$(SILENT) if exist $(subst /,\\\\\\\\,$(OBJDIR)) rmdir /s /q $(subst /,\\\\\\\\,$(OBJDIR))')\n_p('endif')\n_p('')\n_p('prebuild:')\n_p('\\t$(PREBUILDCMDS)')\n_p('')\n_p('prelink:')\n_p('\\t$(PRELINKCMDS)')\n_p('')\ncpp.pchrules(prj)\ncpp.fileRules(prj)\n_p('-include $(OBJECTS:%%.o=%%.d)')\n_p('ifneq (,$(PCH))')\n_p(' -include $(OBJDIR)/$(notdir $(PCH)).d')\n_p('endif')\nend\nfunction premake.gmake_cpp_header(prj, cc, platforms)\n_p('# %s project makefile autogenerated by GENie', premake.action.current().shortname)\n_p('ifndef config')\n_p(' config=%s', _MAKE.esc(premake.getconfigname(prj.solution.configurations[1], platforms[1], true)))\n_p('endif')\n_p('')\n_p('ifndef verbose')\n_p(' SILENT = @')\n_p('endif')\n_p('')\n_p('SHELLTYPE := msdos')\n_p('ifeq (,$(ComSpec)$(COMSPEC))')\n_p(' SHELLTYPE := posix')\n_p('endif')\n_p('i"
|
||||
"feq (/bin,$(findstring /bin,$(SHELL)))')\n_p(' SHELLTYPE := posix')\n_p('endif')\n_p('')\n_p('ifeq (posix,$(SHELLTYPE))')\n_p(' MKDIR = $(SILENT) mkdir -p \"$(1)\"')\n_p(' COPY = $(SILENT) cp -fR \"$(1)\" \"$(2)\"')\n_p('else')\n_p(' MKDIR = $(SILENT) mkdir \"$(subst /,\\\\\\\\,$(1))\" 2> nul || true')\n_p(' COPY = $(SILENT) copy /Y \"$(subst /,\\\\\\\\,$(1))\" \"$(subst /,\\\\\\\\,$(2))\"')\n_p('endif')\n_p('')\n_p('CC = %s', cc.cc)\n_p('CXX = %s', cc.cxx)\n_p('AR = %s', cc.ar)\n_p('')\n_p('ifndef RESCOMP')\n_p(' ifdef WINDRES')\n_p(' RESCOMP = $(WINDRES)')\n_p(' else')\n_p(' RESCOMP = windres')\n_p(' endif')\n_p('endif')\n_p('')\nend\nfunction premake.gmake_cpp_config(cfg, cc)\n_p('ifeq ($(config),%s)', _MAKE.esc(cfg.shortname))\ncpp.platformtools(cfg, cc)\n_p(' OBJDIR = %s', _MAKE.esc(cfg.objectsdir))\n_p(' TARGETDIR = %s', _MAKE.esc(cfg.buildtarget.directory))\n_p(' TARGET = $(TARGETDIR)/%s', _MAKE.esc(cfg.buildtarget.name))\n_p(' DEFINES +=%s', make.list(cc.getdefines(cfg."
|
||||
"defines)))\n_p(' INCLUDES +=%s', make.list(cc.getincludedirs(cfg.includedirs)))\ncpp.pchconfig(cfg)\ncpp.flags(cfg, cc)\ncpp.linker(cfg, cc)\n_p(' define PREBUILDCMDS')\nif #cfg.prebuildcommands > 0 then\n_p('\\t@echo Running pre-build commands')\n_p('\\t%s', table.implode(cfg.prebuildcommands, \"\", \"\", \"\\n\\t\"))\nend\n_p(' endef')\n_p(' define PRELINKCMDS')\nif #cfg.prelinkcommands > 0 then\n_p('\\t@echo Running pre-link commands')\n_p('\\t%s', table.implode(cfg.prelinkcommands, \"\", \"\", \"\\n\\t\"))\nend\n_p(' endef')\n_p(' define POSTBUILDCMDS')\nif #cfg.postbuildcommands > 0 then\n_p('\\t@echo Running post-build commands')\n_p('\\t%s', table.implode(cfg.postbuildcommands, \"\", \"\", \"\\n\\t\"))\nend\n_p(' endef')\nmake.settings(cfg, cc)\n_p('endif')\n_p('')\nend\nfunction cpp.platformtools(cfg, cc)\nlocal platform = cc.platforms[cfg.platform]\nif platform.cc then\n_p(' CC = %s', platform.cc)\nend\nif platform.cxx then\n_p(' CXX = %s', platform.cxx)\nend\nif platform.ar t"
|
||||
"hen\n_p(' AR = %s', platform.ar)\nend\nend\nfunction cpp.flags(cfg, cc)\nif cfg.pchheader and not cfg.flags.NoPCH then\n_p(' FORCE_INCLUDE += -include $(OBJDIR)/$(notdir $(PCH))')\nend\nif #cfg.forcedincludes > 0 then\n_p(' FORCE_INCLUDE += -include %s'\n,premake.esc(table.concat(cfg.forcedincludes, \";\")))\nend\n_p(' ALL_CPPFLAGS += $(CPPFLAGS) %s $(DEFINES) $(INCLUDES)', table.concat(cc.getcppflags(cfg), \" \"))\n_p(' ALL_CFLAGS += $(CFLAGS) $(ALL_CPPFLAGS) $(ARCH)%s', make.list(table.join(cc.getcflags(cfg), cfg.buildoptions)))\n_p(' ALL_CXXFLAGS += $(CXXFLAGS) $(ALL_CFLAGS)%s', make.list(table.join(cc.getcxxflags(cfg), cfg.buildoptions_cpp)))\n_p(' ALL_RESFLAGS += $(RESFLAGS) $(DEFINES) $(INCLUDES)%s',\n make.list(table.join(cc.getdefines(cfg.resdefines),\n cc.getincludedirs(cfg.resincludedirs), cfg.resoptions)))\nend\nfunction cpp.linker(cfg, cc)\n_p(' ALL_LDFLAGS += $(LDFLAGS)%s', make.list(table.join(cc.getlibdirflags(cfg), cc.getldflags(cfg)"
|
||||
", cfg.linkoptions)))\n_p(' LDDEPS +=%s', make.list(_MAKE.esc(premake.getlinks(cfg, \"siblings\", \"fullpath\"))))\n_p(' LIBS += $(LDDEPS)%s', make.list(cc.getlinkflags(cfg)))\nif cfg.kind == \"StaticLib\" then\nif cfg.platform:startswith(\"Universal\") then\n_p(' LINKCMD = libtool -o $(TARGET)')\nelse\nif cc.llvm then\n_p(' LINKCMD = $(AR) rcs $(TARGET)')\nelse\n_p(' LINKCMD = $(AR) -rcs $(TARGET)')\nend\nend\nelse\nlocal tool = iif(cfg.language == \"C\", \"CC\", \"CXX\")\n_p(' LINKCMD = $(%s) -o $(TARGET) $(OBJECTS) $(RESOURCES) $(ARCH) $(ALL_LDFLAGS) $(LIBS)', tool)\nend\nend\nfunction cpp.pchconfig(cfg)\nif not cfg.pchheader or cfg.flags.NoPCH then\nreturn\nend\nlocal pch = cfg.pchheader\nfor _, incdir in ipairs(cfg.includedirs) do\nlocal abspath = path.getabsolute(path.join(cfg.project.location, incdir))\nlocal testname = path.join(abspath, pch)\nif os.isfile(testname) then\npch = path.getrelative(cfg.location, testname)\nbreak\nend\nend\n_p(' PCH = %s', _MAKE.esc(pch))\n_"
|
||||
"p(' GCH = $(OBJDIR)/$(notdir $(PCH)).gch')\nend\nfunction cpp.pchrules(prj)\n_p('ifneq (,$(PCH))')\n_p('$(GCH): $(PCH)')\n_p('\\t@echo $(notdir $<)')\nlocal cmd = iif(prj.language == \"C\", \"$(CC) -x c-header $(ALL_CFLAGS)\", \"$(CXX) -x c++-header $(ALL_CXXFLAGS)\")\n_p('\\t$(SILENT) %s -MMD -MP $(DEFINES) $(INCLUDES) -o \"$@\" -MF \"$(@:%%.gch=%%.d)\" -c \"$<\"', cmd)\n_p('endif')\n_p('')\nend\nfunction cpp.fileRules(prj)\nfor _, file in ipairs(prj.files or {}) do\nif path.iscppfile(file) then\n_p('$(OBJDIR)/%s.o: %s'\n, _MAKE.esc(path.trimdots(path.removeext(file)))\n, _MAKE.esc(file)\n)\nif prj.msgcompile then\n_p('\\t@echo ' .. prj.msgcompile)\nelse\n_p('\\t@echo $(notdir $<)')\nend\ncpp.buildcommand(path.iscfile(file) and not prj.options.ForceCPP, \"o\")\n_p('')\nelseif (path.getextension(file) == \".rc\") then\n_p('$(OBJDIR)/%s.res: %s', _MAKE.esc(path.getbasename(file)), _MAKE.esc(file))\nif prj.msgresource then\n_p('\\t@echo ' .. prj.msgresource)\nelse\n_p('\\t@echo $(notdir $<)')\nend\n_p('"
|
||||
"\\t$(SILENT) $(RESCOMP) $< -O coff -o \"$@\" $(ALL_RESFLAGS)')\n_p('')\nend\nend\nend\nfunction cpp.buildcommand(iscfile, objext)\nlocal flags = iif(iscfile, '$(CC) $(ALL_CFLAGS)', '$(CXX) $(ALL_CXXFLAGS)')\n_p('\\t$(SILENT) %s $(FORCE_INCLUDE) -o \"$@\" -MF $(@:%%.%s=%%.d) -c \"$<\"', flags, objext)\nend\n",
|
||||
"premake.make.cpp = { }\nlocal cpp = premake.make.cpp\nlocal make = premake.make\nfunction premake.make_cpp(prj)\nlocal cc = premake.gettool(prj)\nlocal platforms = premake.filterplatforms(prj.solution, cc.platforms, \"Native\")\npremake.gmake_cpp_header(prj, cc, platforms)\nfor _, platform in ipairs(platforms) do\nfor cfg in premake.eachconfig(prj, platform) do\npremake.gmake_cpp_config(prj, cfg, cc)\nend\nend\nlocal objdirs = {}\nfor _, file in ipairs(prj.files) do\nif path.iscppfile(file) then\nobjdirs[_MAKE.esc(path.getdirectory(path.trimdots(file)))] = 1\nend\nend\n_p('OBJDIRS := \\\\')\n_p('\\t$(OBJDIR) \\\\')\nfor dir, _ in pairs(objdirs) do\n_p('\\t$(OBJDIR)/%s \\\\', dir)\nend\n_p('')\n_p('RESOURCES := \\\\')\nfor _, file in ipairs(prj.files) do\nif path.isresourcefile(file) then\n_p('\\t$(OBJDIR)/%s.res \\\\', _MAKE.esc(path.getbasename(file)))\nend\nend\n_p('')\n_p('.PHONY: clean prebuild prelink')\n_p('')\nif os.is(\"MacOSX\") and prj.kind == \"WindowedApp\" then\n_p('all: $(TARGETDIR) $(OBJDIRS) pr"
|
||||
"ebuild prelink $(TARGET) $(dir $(TARGETDIR))PkgInfo $(dir $(TARGETDIR))Info.plist')\nelse\n_p('all: $(TARGETDIR) $(OBJDIRS) prebuild prelink $(TARGET)')\nend\n_p('\\t@:')\n_p('')\nif (prj.kind == \"StaticLib\" and prj.options.ArchiveSplit) then\n_p('define max_args')\n_p('\\t$(eval _args:=)')\n_p('\\t$(foreach obj,$3,$(eval _args+=$(obj))$(if $(word $2,$(_args)),$1$(_args)$(EOL)$(eval _args:=)))')\n_p('\\t$(if $(_args),$1$(_args))')\n_p('endef')\n_p('')\n_p('define EOL')\n_p('')\n_p('')\n_p('endef')\n_p('')\nend\n_p('$(TARGET): $(GCH) $(OBJECTS) $(LDDEPS) $(RESOURCES)')\nif prj.kind == \"StaticLib\" then\nif prj.msgarchiving then\n_p('\\t@echo ' .. prj.msgarchiving)\nelse\n_p('\\t@echo Archiving %s', prj.name)\nend\nif (not prj.archivesplit_size) then \nprj.archivesplit_size=200\nend\nif (not prj.options.ArchiveSplit) then\n_p('\\t$(SILENT) $(LINKCMD) $(OBJECTS)')\nelse\n_p('\\t@$(call max_args,$(LINKCMD),'.. prj.archivesplit_size ..',$(OBJECTS))')\nend\nelse\nif prj.msglinking then\n_p('\\t@echo ' .. prj.msgl"
|
||||
"inking)\nelse\n_p('\\t@echo Linking %s', prj.name)\nend\n_p('\\t$(SILENT) $(LINKCMD)')\nend\n_p('\\t$(POSTBUILDCMDS)')\n_p('')\n_p('$(TARGETDIR):')\npremake.make_mkdirrule(\"$(TARGETDIR)\")\n_p('$(OBJDIRS):')\nif (not prj.solution.messageskip) or (not table.contains(prj.solution.messageskip, \"SkipCreatingMessage\")) then\n_p('\\t@echo Creating $(OBJDIR)')\nend\n_p('\\t-$(call MKDIR,$(OBJDIR))')\nfor dir, _ in pairs(objdirs) do\n_p('\\t-$(call MKDIR,$(OBJDIR)/%s)', dir)\nend\n_p('')\nif os.is(\"MacOSX\") and prj.kind == \"WindowedApp\" then\n_p('$(dir $(TARGETDIR))PkgInfo:')\n_p('$(dir $(TARGETDIR))Info.plist:')\n_p('')\nend\n_p('clean:')\nif (not prj.solution.messageskip) or (not table.contains(prj.solution.messageskip, \"SkipCleaningMessage\")) then\n_p('\\t@echo Cleaning %s', prj.name)\nend\n_p('ifeq (posix,$(SHELLTYPE))')\n_p('\\t$(SILENT) rm -f $(TARGET)')\n_p('\\t$(SILENT) rm -rf $(OBJDIR)')\n_p('else')\n_p('\\t$(SILENT) if exist $(subst /,\\\\\\\\,$(TARGET)) del $(subst /,\\\\\\\\,$(TARGET))')\n_p('\\t"
|
||||
"$(SILENT) if exist $(subst /,\\\\\\\\,$(OBJDIR)) rmdir /s /q $(subst /,\\\\\\\\,$(OBJDIR))')\n_p('endif')\n_p('')\n_p('prebuild:')\n_p('\\t$(PREBUILDCMDS)')\n_p('')\n_p('prelink:')\n_p('\\t$(PRELINKCMDS)')\n_p('')\ncpp.pchrules(prj)\ncpp.fileRules(prj)\n_p('-include $(OBJECTS:%%.o=%%.d)')\n_p('ifneq (,$(PCH))')\n_p(' -include $(OBJDIR)/$(notdir $(PCH)).d')\n_p('endif')\nend\nfunction premake.gmake_cpp_header(prj, cc, platforms)\n_p('# %s project makefile autogenerated by GENie', premake.action.current().shortname)\n_p('ifndef config')\n_p(' config=%s', _MAKE.esc(premake.getconfigname(prj.solution.configurations[1], platforms[1], true)))\n_p('endif')\n_p('')\n_p('ifndef verbose')\n_p(' SILENT = @')\n_p('endif')\n_p('')\n_p('SHELLTYPE := msdos')\n_p('ifeq (,$(ComSpec)$(COMSPEC))')\n_p(' SHELLTYPE := posix')\n_p('endif')\n_p('ifeq (/bin,$(findstring /bin,$(SHELL)))')\n_p(' SHELLTYPE := posix')\n_p('endif')\n_p('')\n_p('ifeq (posix,$(SHELLTYPE))')\n_p(' MKDIR = $(SILENT) mkdir -p \"$(1)\"')\n_p(' COPY = $("
|
||||
"SILENT) cp -fR \"$(1)\" \"$(2)\"')\n_p('else')\n_p(' MKDIR = $(SILENT) mkdir \"$(subst /,\\\\\\\\,$(1))\" 2> nul || true')\n_p(' COPY = $(SILENT) copy /Y \"$(subst /,\\\\\\\\,$(1))\" \"$(subst /,\\\\\\\\,$(2))\"')\n_p('endif')\n_p('')\n_p('CC = %s', cc.cc)\n_p('CXX = %s', cc.cxx)\n_p('AR = %s', cc.ar)\n_p('')\n_p('ifndef RESCOMP')\n_p(' ifdef WINDRES')\n_p(' RESCOMP = $(WINDRES)')\n_p(' else')\n_p(' RESCOMP = windres')\n_p(' endif')\n_p('endif')\n_p('')\nend\nfunction premake.gmake_cpp_config(prj, cfg, cc)\n_p('ifeq ($(config),%s)', _MAKE.esc(cfg.shortname))\ncpp.platformtools(cfg, cc)\n_p(' OBJDIR = %s', _MAKE.esc(cfg.objectsdir))\n_p(' TARGETDIR = %s', _MAKE.esc(cfg.buildtarget.directory))\n_p(' TARGET = $(TARGETDIR)/%s', _MAKE.esc(cfg.buildtarget.name))\n_p(' DEFINES +=%s', make.list(cc.getdefines(cfg.defines)))\n_p(' INCLUDES +=%s', make.list(cc.getincludedirs(cfg.includedirs)))\ncpp.pchconfig(cfg)\ncpp.flags(cfg, cc)\ncpp.linker(cfg, cc)\n_p(' OBJECTS := \\\\')\nfor _, file"
|
||||
" in ipairs(prj.files) do\nif path.iscppfile(file) then\nlocal excluded = false\nfor _, exclude in ipairs(cfg.excludes) do\nexcluded = (exclude == file)\nif (excluded) then break end\nend\nif excluded == false then\n_p('\\t$(OBJDIR)/%s.o \\\\'\n, _MAKE.esc(path.trimdots(path.removeext(file)))\n)\nend\nend\nend\n_p('')\n_p(' define PREBUILDCMDS')\nif #cfg.prebuildcommands > 0 then\n_p('\\t@echo Running pre-build commands')\n_p('\\t%s', table.implode(cfg.prebuildcommands, \"\", \"\", \"\\n\\t\"))\nend\n_p(' endef')\n_p(' define PRELINKCMDS')\nif #cfg.prelinkcommands > 0 then\n_p('\\t@echo Running pre-link commands')\n_p('\\t%s', table.implode(cfg.prelinkcommands, \"\", \"\", \"\\n\\t\"))\nend\n_p(' endef')\n_p(' define POSTBUILDCMDS')\nif #cfg.postbuildcommands > 0 then\n_p('\\t@echo Running post-build commands')\n_p('\\t%s', table.implode(cfg.postbuildcommands, \"\", \"\", \"\\n\\t\"))\nend\n_p(' endef')\nmake.settings(cfg, cc)\n_p('endif')\n_p('')\nend\nfunction cpp.platformtools(cfg, cc)\nlocal platform "
|
||||
"= cc.platforms[cfg.platform]\nif platform.cc then\n_p(' CC = %s', platform.cc)\nend\nif platform.cxx then\n_p(' CXX = %s', platform.cxx)\nend\nif platform.ar then\n_p(' AR = %s', platform.ar)\nend\nend\nfunction cpp.flags(cfg, cc)\nif cfg.pchheader and not cfg.flags.NoPCH then\n_p(' FORCE_INCLUDE += -include $(OBJDIR)/$(notdir $(PCH))')\nend\nif #cfg.forcedincludes > 0 then\n_p(' FORCE_INCLUDE += -include %s'\n,premake.esc(table.concat(cfg.forcedincludes, \";\")))\nend\n_p(' ALL_CPPFLAGS += $(CPPFLAGS) %s $(DEFINES) $(INCLUDES)', table.concat(cc.getcppflags(cfg), \" \"))\n_p(' ALL_CFLAGS += $(CFLAGS) $(ALL_CPPFLAGS) $(ARCH)%s', make.list(table.join(cc.getcflags(cfg), cfg.buildoptions)))\n_p(' ALL_CXXFLAGS += $(CXXFLAGS) $(ALL_CFLAGS)%s', make.list(table.join(cc.getcxxflags(cfg), cfg.buildoptions_cpp)))\n_p(' ALL_RESFLAGS += $(RESFLAGS) $(DEFINES) $(INCLUDES)%s',\n make.list(table.join(cc.getdefines(cfg.resdefines),\n cc.getincludedirs("
|
||||
"cfg.resincludedirs), cfg.resoptions)))\nend\nfunction cpp.linker(cfg, cc)\n_p(' ALL_LDFLAGS += $(LDFLAGS)%s', make.list(table.join(cc.getlibdirflags(cfg), cc.getldflags(cfg), cfg.linkoptions)))\n_p(' LDDEPS +=%s', make.list(_MAKE.esc(premake.getlinks(cfg, \"siblings\", \"fullpath\"))))\n_p(' LIBS += $(LDDEPS)%s', make.list(cc.getlinkflags(cfg)))\nif cfg.kind == \"StaticLib\" then\nif cfg.platform:startswith(\"Universal\") then\n_p(' LINKCMD = libtool -o $(TARGET)')\nelse\nif cc.llvm then\n_p(' LINKCMD = $(AR) rcs $(TARGET)')\nelse\n_p(' LINKCMD = $(AR) -rcs $(TARGET)')\nend\nend\nelse\nlocal tool = iif(cfg.language == \"C\", \"CC\", \"CXX\")\n_p(' LINKCMD = $(%s) -o $(TARGET) $(OBJECTS) $(RESOURCES) $(ARCH) $(ALL_LDFLAGS) $(LIBS)', tool)\nend\nend\nfunction cpp.pchconfig(cfg)\nif not cfg.pchheader or cfg.flags.NoPCH then\nreturn\nend\nlocal pch = cfg.pchheader\nfor _, incdir in ipairs(cfg.includedirs) do\nlocal abspath = path.getabsolute(path.join(cfg.project.location, incdir))\nlo"
|
||||
"cal testname = path.join(abspath, pch)\nif os.isfile(testname) then\npch = path.getrelative(cfg.location, testname)\nbreak\nend\nend\n_p(' PCH = %s', _MAKE.esc(pch))\n_p(' GCH = $(OBJDIR)/$(notdir $(PCH)).gch')\nend\nfunction cpp.pchrules(prj)\n_p('ifneq (,$(PCH))')\n_p('$(GCH): $(PCH)')\n_p('\\t@echo $(notdir $<)')\nlocal cmd = iif(prj.language == \"C\", \"$(CC) -x c-header $(ALL_CFLAGS)\", \"$(CXX) -x c++-header $(ALL_CXXFLAGS)\")\n_p('\\t$(SILENT) %s -MMD -MP $(DEFINES) $(INCLUDES) -o \"$@\" -MF \"$(@:%%.gch=%%.d)\" -c \"$<\"', cmd)\n_p('endif')\n_p('')\nend\nfunction cpp.fileRules(prj)\nfor _, file in ipairs(prj.files or {}) do\nif path.iscppfile(file) then\n_p('$(OBJDIR)/%s.o: %s'\n, _MAKE.esc(path.trimdots(path.removeext(file)))\n, _MAKE.esc(file)\n)\nif prj.msgcompile then\n_p('\\t@echo ' .. prj.msgcompile)\nelse\n_p('\\t@echo $(notdir $<)')\nend\ncpp.buildcommand(path.iscfile(file) and not prj.options.ForceCPP, \"o\")\n_p('')\nelseif (path.getextension(file) == \".rc\") then\n_p('$(OBJD"
|
||||
"IR)/%s.res: %s', _MAKE.esc(path.getbasename(file)), _MAKE.esc(file))\nif prj.msgresource then\n_p('\\t@echo ' .. prj.msgresource)\nelse\n_p('\\t@echo $(notdir $<)')\nend\n_p('\\t$(SILENT) $(RESCOMP) $< -O coff -o \"$@\" $(ALL_RESFLAGS)')\n_p('')\nend\nend\nend\nfunction cpp.buildcommand(iscfile, objext)\nlocal flags = iif(iscfile, '$(CC) $(ALL_CFLAGS)', '$(CXX) $(ALL_CXXFLAGS)')\n_p('\\t$(SILENT) %s $(FORCE_INCLUDE) -o \"$@\" -MF $(@:%%.%s=%%.d) -c \"$<\"', flags, objext)\nend\n",
|
||||
|
||||
/* actions/make/make_csharp.lua */
|
||||
"local function getresourcefilename(cfg, fname)\nif path.getextension(fname) == \".resx\" then\n local name = cfg.buildtarget.basename .. \".\"\n local dir = path.getdirectory(fname)\n if dir ~= \".\" then \nname = name .. path.translate(dir, \".\") .. \".\"\nend\nreturn \"$(OBJDIR)/\" .. _MAKE.esc(name .. path.getbasename(fname)) .. \".resources\"\nelse\nreturn fname\nend\nend\nfunction premake.make_csharp(prj)\nlocal csc = premake.dotnet\nlocal cfglibs = { }\nlocal cfgpairs = { }\nlocal anycfg\nfor cfg in premake.eachconfig(prj) do\nanycfg = cfg\ncfglibs[cfg] = premake.getlinks(cfg, \"siblings\", \"fullpath\")\ncfgpairs[cfg] = { }\nfor _, fname in ipairs(cfglibs[cfg]) do\nif path.getdirectory(fname) ~= cfg.buildtarget.directory then\ncfgpairs[cfg][\"$(TARGETDIR)/\" .. _MAKE.esc(path.getname(fname))] = _MAKE.esc(fname)\nend\nend\nend\nlocal sources = {}\nlocal embedded = { }\nlocal copypairs = { }\nfor fcfg in premake.project.eachfile(prj) do\nlocal action = csc.getbuildaction(fcfg)\nif action == \"Co"
|
||||
|
Loading…
Reference in New Issue
Block a user