mirror of
https://github.com/holub/mame
synced 2025-07-11 12:44:54 +03:00
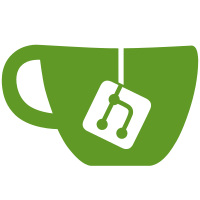
* Got rid of some more simple_list in core debugger code * Fixed a buffer overrun in wavwrite (buffer half requried size) * Slightly reduced dependencies and overhead in wavwrite * Made new disassembly windows in Qt debugger default to current CPU
81 lines
1.7 KiB
C++
81 lines
1.7 KiB
C++
// license:BSD-3-Clause
|
|
// copyright-holders:Andrew Gardner
|
|
#ifndef __DEBUG_QT_DASM_WINDOW_H__
|
|
#define __DEBUG_QT_DASM_WINDOW_H__
|
|
|
|
#include <QtWidgets/QLineEdit>
|
|
#include <QtWidgets/QComboBox>
|
|
|
|
#include "debuggerview.h"
|
|
#include "windowqt.h"
|
|
|
|
|
|
//============================================================
|
|
// The Disassembly Window.
|
|
//============================================================
|
|
class DasmWindow : public WindowQt
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
DasmWindow(running_machine* machine, QWidget* parent=nullptr);
|
|
virtual ~DasmWindow();
|
|
|
|
|
|
private slots:
|
|
void cpuChanged(int index);
|
|
void expressionSubmitted();
|
|
|
|
void toggleBreakpointAtCursor(bool changedTo);
|
|
void enableBreakpointAtCursor(bool changedTo);
|
|
void runToCursor(bool changedTo);
|
|
void rightBarChanged(QAction* changedTo);
|
|
|
|
void dasmViewUpdated();
|
|
|
|
|
|
private:
|
|
void populateComboBox();
|
|
void setToCurrentCpu();
|
|
|
|
|
|
// Widgets
|
|
QLineEdit* m_inputEdit;
|
|
QComboBox* m_cpuComboBox;
|
|
DebuggerView* m_dasmView;
|
|
|
|
// Menu items
|
|
QAction* m_breakpointToggleAct;
|
|
QAction* m_breakpointEnableAct;
|
|
QAction* m_runToCursorAct;
|
|
};
|
|
|
|
|
|
//=========================================================================
|
|
// A way to store the configuration of a window long enough to read/write.
|
|
//=========================================================================
|
|
class DasmWindowQtConfig : public WindowQtConfig
|
|
{
|
|
public:
|
|
DasmWindowQtConfig() :
|
|
WindowQtConfig(WIN_TYPE_DASM),
|
|
m_cpu(0),
|
|
m_rightBar(0)
|
|
{
|
|
}
|
|
|
|
~DasmWindowQtConfig() {}
|
|
|
|
// Settings
|
|
int m_cpu;
|
|
int m_rightBar;
|
|
|
|
void buildFromQWidget(QWidget* widget);
|
|
void applyToQWidget(QWidget* widget);
|
|
void addToXmlDataNode(util::xml::data_node &node) const;
|
|
void recoverFromXmlNode(util::xml::data_node const &node);
|
|
};
|
|
|
|
|
|
#endif
|