mirror of
https://github.com/holub/mame
synced 2025-05-08 23:31:54 +03:00
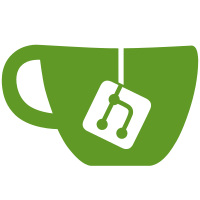
osd_free(). They take the same parameters as malloc() and free(). Renamed mamecore.h -> emucore.h. New C++-aware memory manager, implemented in emualloc.*. This is a simple manager that allows you to add any type of object to a resource pool. Most commonly, allocated objects are added, and so a set of allocation macros is provided to allow you to manage objects in a particular pool: pool_alloc(p, t) = allocate object of type 't' and add to pool 'p' pool_alloc_clear(p, t) = same as above, but clear the memory first pool_alloc_array(p, t, c) = allocate an array of 'c' objects of type 't' and add to pool 'p' pool_alloc_array_clear(p, t, c) = same, but with clearing pool_free(p, v) = free object 'v' and remove it from the pool Note that pool_alloc[_clear] is roughly equivalent to "new t" and pool_alloc_array[_clear] is roughly equivalent to "new t[c]". Also note that pool_free works for single objects and arrays. There is a single global_resource_pool defined which should be used for any global allocations. It has equivalent macros to the pool_* macros above that automatically target the global pool. In addition, the memory module defines global new/delete overrides that access file and line number parameters so that allocations can be tracked. Currently this tracking is only done if MAME_DEBUG is enabled. In debug builds, any unfreed memory will be printed at the end of the session. emualloc.h also has #defines to disable malloc/free/realloc/calloc. Since emualloc.h is included by emucore.h, this means pretty much all code within the emulator is forced to use the new allocators. Although straight new/delete do work, their use is discouraged, as any allocations made with them will not be tracked. Changed the familar auto_alloc_* macros to map to the resource pool model described above. The running_machine is now a class and contains a resource pool which is automatically destructed upon deletion. If you are a driver writer, all your allocations should be done with auto_alloc_*. Changed all drivers and files in the core using malloc/realloc or the old alloc_*_or_die macros to use (preferably) the auto_alloc_* macros instead, or the global_alloc_* macros if necessary. Added simple C++ wrappers for astring and bitmap_t, as these need proper constructors/destructors to be used for auto_alloc_astring and auto_alloc_bitmap. Removed references to the winalloc prefix file. Most of its functionality has moved into the core, save for the guard page allocations, which are now implemented in osd_alloc and osd_free.
103 lines
2.6 KiB
C
103 lines
2.6 KiB
C
/***************************************************************************
|
|
|
|
audit.h
|
|
|
|
ROM, disk, and sample auditing functions.
|
|
|
|
Copyright Nicola Salmoria and the MAME Team.
|
|
Visit http://mamedev.org for licensing and usage restrictions.
|
|
|
|
***************************************************************************/
|
|
|
|
#pragma once
|
|
|
|
#ifndef __AUDIT_H__
|
|
#define __AUDIT_H__
|
|
|
|
#include "emucore.h"
|
|
#include "hash.h"
|
|
|
|
|
|
|
|
/***************************************************************************
|
|
CONSTANTS
|
|
***************************************************************************/
|
|
|
|
/* hashes to use for validation */
|
|
#define AUDIT_VALIDATE_FAST (HASH_CRC)
|
|
#define AUDIT_VALIDATE_FULL (HASH_CRC | HASH_SHA)
|
|
|
|
/* return values from audit_verify_roms and audit_verify_samples */
|
|
enum
|
|
{
|
|
CORRECT = 0,
|
|
BEST_AVAILABLE,
|
|
INCORRECT,
|
|
NOTFOUND
|
|
};
|
|
|
|
/* image types for audit_record.type */
|
|
enum
|
|
{
|
|
AUDIT_FILE_ROM = 0,
|
|
AUDIT_FILE_DISK,
|
|
AUDIT_FILE_SAMPLE
|
|
};
|
|
|
|
/* status values for audit_record.status */
|
|
enum
|
|
{
|
|
AUDIT_STATUS_GOOD = 0,
|
|
AUDIT_STATUS_FOUND_INVALID,
|
|
AUDIT_STATUS_NOT_FOUND,
|
|
AUDIT_STATUS_ERROR
|
|
};
|
|
|
|
/* substatus values for audit_record.substatus */
|
|
enum
|
|
{
|
|
SUBSTATUS_GOOD = 0,
|
|
SUBSTATUS_GOOD_NEEDS_REDUMP,
|
|
SUBSTATUS_FOUND_NODUMP,
|
|
SUBSTATUS_FOUND_BAD_CHECKSUM,
|
|
SUBSTATUS_FOUND_WRONG_LENGTH,
|
|
SUBSTATUS_NOT_FOUND,
|
|
SUBSTATUS_NOT_FOUND_NODUMP,
|
|
SUBSTATUS_NOT_FOUND_OPTIONAL,
|
|
SUBSTATUS_NOT_FOUND_PARENT,
|
|
SUBSTATUS_NOT_FOUND_BIOS,
|
|
SUBSTATUS_ERROR = 100
|
|
};
|
|
|
|
|
|
|
|
/***************************************************************************
|
|
TYPE DEFINITIONS
|
|
***************************************************************************/
|
|
|
|
typedef struct _audit_record audit_record;
|
|
struct _audit_record
|
|
{
|
|
UINT8 type; /* type of item that was audited */
|
|
UINT8 status; /* status of audit on this item */
|
|
UINT8 substatus; /* finer-detail status */
|
|
const char * name; /* name of item */
|
|
UINT32 explength; /* expected length of item */
|
|
UINT32 length; /* actual length of item */
|
|
const char * exphash; /* expected hash data */
|
|
char hash[HASH_BUF_SIZE]; /* actual hash information */
|
|
};
|
|
|
|
|
|
|
|
/***************************************************************************
|
|
FUNCTION PROTOTYPES
|
|
***************************************************************************/
|
|
|
|
int audit_images(core_options *options, const game_driver *gamedrv, UINT32 validation, audit_record **audit);
|
|
int audit_samples(core_options *options, const game_driver *gamedrv, audit_record **audit);
|
|
int audit_summary(const game_driver *gamedrv, int count, const audit_record *records, int output);
|
|
|
|
|
|
#endif /* __AUDIT_H__ */
|