mirror of
https://github.com/holub/mame
synced 2025-07-03 09:06:08 +03:00
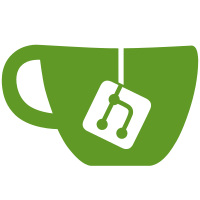
[Aaron Giles] * these classes now no longer take a resource_pool; everything is managed globally -- this means that objects added to lists must be allocated with global_alloc * added new auto_pointer<> template which wraps a pointer and auto-frees it upon destruction; it also defaults to NULL so it doesn't need to be explicitly initialized * moved tagged_list template to tagmap.h Redo of the low-level memory tracking system: [Aaron Giles] * moved low-level tracking out of emu\emualloc into lib\util\corealloc so it can be shared among all components and used by core libraries * global_alloc and friends no longer use a resource pool to track allocations; turns out this was a wholly redundant system that wasted a lot of memory * removed global_resource_pool entirely * added global_free_array to delete arrays allocated with global_alloc_array * added tracking of object versus array allocation; we will now error if you use global_free on an array, or global_free_array on an object Added new utility helper const_string_pool which can be used to efficiently accumulate strings that are not intended to be modified. Used by updated makelist and software list code. [Aaron Giles] Updated png2bdc and makelist tools to not leak memory and use more modern techniques (no more MAX_DRIVERS in makelist, for example). [Aaron Giles] Deprecated auto_strdup and removed all uses by way of caller-managed astrings and the software list rewrite. [Aaron Giles] Rewrote software list management: [Aaron Giles] * removed the notion of a software_list that is separate from a software_list_device; they are one and the same now * moved several functions into device_image_interface since they really didn't belong in the core software list class * lots of simplification as a result of the above changes Additional notes (no whatsnew): Moved definition of FPTR to osdcomm.h. Some changes happened in the OSD code to fix issues, especially regarding freeing arrays. SDL folks may need to fix up some of these. The following devices still are using tokens and should be modernized (I found them because they kept their token as void * and tried to delete it, which you can't): namco_52xx_device (mame/audio/namco52.c) namco_54xx_device (mame/audio/namco54.c) namco_06xx_device (mame/machine/namco06.c) namco_50xx_device (mame/machine/namco50.c) namco_51xx_device (mame/machine/namco51.c) namco_53xx_device (mame/machine/namco53.c) voodoo_device (emu/video/voodoo.c) mos6581_device (emu/sound/mos6581.c) aica_device (emu/sound/aica.c) scsp_device (emu/sound/scsp.c) dmadac_sound_device (emu/sound/dmadac.c) s3c2440_device (emu/machine/s3c2440.c) wd1770_device (emu/machine/wd17xx.c) latch8_device (emu/machine/latch8.c) duart68681_device (emu/machine/68681.c) s3c2400_device (emu/machine/s3c2400.c) s3c2410_device (emu/machine/s3c2410.c) strataflash_device (mess/machine/strata.c) hd63450_device (mess/machine/hd63450.c) tap_990_device (mess/machine/ti99/990_tap.c) omti8621_device (mess/machine/omti8621.c) vdt911_device (mess/video/911_vdt.c) apollo_graphics_15i (mess/video/apollo.c) asr733_device (mess/video/733_asr.c)
601 lines
18 KiB
C
601 lines
18 KiB
C
// license:BSD-3-Clause
|
|
// copyright-holders:Aaron Giles
|
|
/***************************************************************************
|
|
|
|
audit.c
|
|
|
|
ROM set auditing functions.
|
|
|
|
***************************************************************************/
|
|
|
|
#include "emu.h"
|
|
#include "emuopts.h"
|
|
#include "audit.h"
|
|
#include "harddisk.h"
|
|
#include "sound/samples.h"
|
|
|
|
|
|
//**************************************************************************
|
|
// CORE FUNCTIONS
|
|
//**************************************************************************
|
|
|
|
//-------------------------------------------------
|
|
// media_auditor - constructor
|
|
//-------------------------------------------------
|
|
|
|
media_auditor::media_auditor(const driver_enumerator &enumerator)
|
|
: m_enumerator(enumerator),
|
|
m_validation(AUDIT_VALIDATE_FULL),
|
|
m_searchpath(NULL)
|
|
{
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_media - audit the media described by the
|
|
// currently-enumerated driver
|
|
//-------------------------------------------------
|
|
|
|
media_auditor::summary media_auditor::audit_media(const char *validation)
|
|
{
|
|
// start fresh
|
|
m_record_list.reset();
|
|
|
|
// store validation for later
|
|
m_validation = validation;
|
|
|
|
// temporary hack until romload is update: get the driver path and support it for
|
|
// all searches
|
|
const char *driverpath = m_enumerator.config().root_device().searchpath();
|
|
|
|
int found = 0;
|
|
int required = 0;
|
|
int shared_found = 0;
|
|
int shared_required = 0;
|
|
|
|
// iterate over devices and regions
|
|
device_iterator deviter(m_enumerator.config().root_device());
|
|
for (device_t *device = deviter.first(); device != NULL; device = deviter.next())
|
|
{
|
|
// determine the search path for this source and iterate through the regions
|
|
m_searchpath = device->searchpath();
|
|
|
|
// now iterate over regions and ROMs within
|
|
for (const rom_entry *region = rom_first_region(*device); region != NULL; region = rom_next_region(region))
|
|
{
|
|
// temporary hack: add the driver path & region name
|
|
astring combinedpath(device->searchpath(), ";", driverpath);
|
|
if (device->shortname())
|
|
combinedpath.cat(";").cat(device->shortname());
|
|
m_searchpath = combinedpath;
|
|
|
|
for (const rom_entry *rom = rom_first_file(region); rom; rom = rom_next_file(rom))
|
|
{
|
|
const char *name = ROM_GETNAME(rom);
|
|
hash_collection hashes(ROM_GETHASHDATA(rom));
|
|
device_t *shared_device = find_shared_device(*device, name, hashes, ROM_GETLENGTH(rom));
|
|
|
|
// count the number of files with hashes
|
|
if (!hashes.flag(hash_collection::FLAG_NO_DUMP) && !ROM_ISOPTIONAL(rom))
|
|
{
|
|
required++;
|
|
if (shared_device != NULL)
|
|
shared_required++;
|
|
}
|
|
|
|
// audit a file
|
|
audit_record *record = NULL;
|
|
if (ROMREGION_ISROMDATA(region))
|
|
record = audit_one_rom(rom);
|
|
|
|
// audit a disk
|
|
else if (ROMREGION_ISDISKDATA(region))
|
|
record = audit_one_disk(rom);
|
|
|
|
if (record != NULL)
|
|
{
|
|
// count the number of files that are found.
|
|
if (record->status() == audit_record::STATUS_GOOD || (record->status() == audit_record::STATUS_FOUND_INVALID && find_shared_device(*device, name, record->actual_hashes(), record->actual_length()) == NULL))
|
|
{
|
|
found++;
|
|
if (shared_device != NULL)
|
|
shared_found++;
|
|
}
|
|
|
|
record->set_shared_device(shared_device);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
// if we only find files that are in the parent & either the set has no unique files or the parent is not found, then assume we don't have the set at all
|
|
if (found == shared_found && required > 0 && (required != shared_required || shared_found == 0))
|
|
{
|
|
m_record_list.reset();
|
|
return NOTFOUND;
|
|
}
|
|
|
|
// return a summary
|
|
return summarize(m_enumerator.driver().name);
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_device - audit the device
|
|
//-------------------------------------------------
|
|
|
|
media_auditor::summary media_auditor::audit_device(device_t *device, const char *validation)
|
|
{
|
|
// start fresh
|
|
m_record_list.reset();
|
|
|
|
// store validation for later
|
|
m_validation = validation;
|
|
m_searchpath = device->shortname();
|
|
|
|
int found = 0;
|
|
int required = 0;
|
|
|
|
// now iterate over regions and ROMs within
|
|
for (const rom_entry *region = rom_first_region(*device); region != NULL; region = rom_next_region(region))
|
|
{
|
|
for (const rom_entry *rom = rom_first_file(region); rom; rom = rom_next_file(rom))
|
|
{
|
|
hash_collection hashes(ROM_GETHASHDATA(rom));
|
|
|
|
// count the number of files with hashes
|
|
if (!hashes.flag(hash_collection::FLAG_NO_DUMP) && !ROM_ISOPTIONAL(rom))
|
|
{
|
|
required++;
|
|
}
|
|
|
|
// audit a file
|
|
audit_record *record = NULL;
|
|
if (ROMREGION_ISROMDATA(region))
|
|
record = audit_one_rom(rom);
|
|
|
|
// audit a disk
|
|
else if (ROMREGION_ISDISKDATA(region))
|
|
record = audit_one_disk(rom);
|
|
|
|
// count the number of files that are found.
|
|
if (record != NULL && (record->status() == audit_record::STATUS_GOOD || record->status() == audit_record::STATUS_FOUND_INVALID))
|
|
{
|
|
found++;
|
|
}
|
|
}
|
|
}
|
|
|
|
if (found == 0 && required > 0)
|
|
{
|
|
m_record_list.reset();
|
|
return NOTFOUND;
|
|
}
|
|
|
|
// return a summary
|
|
return summarize(device->shortname());
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_software
|
|
//-------------------------------------------------
|
|
media_auditor::summary media_auditor::audit_software(const char *list_name, software_info *swinfo, const char *validation)
|
|
{
|
|
// start fresh
|
|
m_record_list.reset();
|
|
|
|
// store validation for later
|
|
m_validation = validation;
|
|
|
|
astring combinedpath(swinfo->shortname(), ";", list_name, PATH_SEPARATOR, swinfo->shortname());
|
|
astring locationtag(list_name, "%", swinfo->shortname(), "%");
|
|
if (swinfo->parentname() != NULL)
|
|
{
|
|
locationtag.cat(swinfo->parentname());
|
|
combinedpath.cat(";").cat(swinfo->parentname()).cat(";").cat(list_name).cat(PATH_SEPARATOR).cat(swinfo->parentname());
|
|
}
|
|
m_searchpath = combinedpath;
|
|
|
|
int found = 0;
|
|
int required = 0;
|
|
|
|
// now iterate over software parts
|
|
for ( software_part *part = swinfo->first_part(); part != NULL; part = part->next() )
|
|
{
|
|
// now iterate over regions
|
|
for ( const rom_entry *region = part->romdata(); region; region = rom_next_region( region ) )
|
|
{
|
|
// now iterate over rom definitions
|
|
for (const rom_entry *rom = rom_first_file(region); rom; rom = rom_next_file(rom))
|
|
{
|
|
hash_collection hashes(ROM_GETHASHDATA(rom));
|
|
|
|
// count the number of files with hashes
|
|
if (!hashes.flag(hash_collection::FLAG_NO_DUMP) && !ROM_ISOPTIONAL(rom))
|
|
{
|
|
required++;
|
|
}
|
|
|
|
// audit a file
|
|
audit_record *record = NULL;
|
|
if (ROMREGION_ISROMDATA(region))
|
|
{
|
|
record = audit_one_rom(rom);
|
|
}
|
|
// audit a disk
|
|
else if (ROMREGION_ISDISKDATA(region))
|
|
{
|
|
record = audit_one_disk(rom, (const char *)locationtag);
|
|
}
|
|
|
|
// count the number of files that are found.
|
|
if (record != NULL && (record->status() == audit_record::STATUS_GOOD || record->status() == audit_record::STATUS_FOUND_INVALID))
|
|
{
|
|
found++;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
if (found == 0 && required > 0)
|
|
{
|
|
m_record_list.reset();
|
|
return NOTFOUND;
|
|
}
|
|
|
|
// return a summary
|
|
return summarize(list_name);
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_samples - validate the samples for the
|
|
// currently-enumerated driver
|
|
//-------------------------------------------------
|
|
|
|
media_auditor::summary media_auditor::audit_samples()
|
|
{
|
|
// start fresh
|
|
m_record_list.reset();
|
|
|
|
int required = 0;
|
|
int found = 0;
|
|
|
|
// iterate over sample entries
|
|
samples_device_iterator iter(m_enumerator.config().root_device());
|
|
for (samples_device *device = iter.first(); device != NULL; device = iter.next())
|
|
{
|
|
// by default we just search using the driver name
|
|
astring searchpath(m_enumerator.driver().name);
|
|
|
|
// add the alternate path if present
|
|
samples_iterator iter(*device);
|
|
if (iter.altbasename() != NULL)
|
|
searchpath.cat(";").cat(iter.altbasename());
|
|
|
|
// iterate over samples in this entry
|
|
for (const char *samplename = iter.first(); samplename != NULL; samplename = iter.next())
|
|
{
|
|
required++;
|
|
|
|
// create a new record
|
|
audit_record &record = m_record_list.append(*global_alloc(audit_record(samplename, audit_record::MEDIA_SAMPLE)));
|
|
|
|
// look for the files
|
|
emu_file file(m_enumerator.options().sample_path(), OPEN_FLAG_READ | OPEN_FLAG_NO_PRELOAD);
|
|
path_iterator path(searchpath);
|
|
astring curpath;
|
|
while (path.next(curpath, samplename))
|
|
{
|
|
// attempt to access the file (.flac) or (.wav)
|
|
file_error filerr = file.open(curpath, ".flac");
|
|
if (filerr != FILERR_NONE)
|
|
filerr = file.open(curpath, ".wav");
|
|
|
|
if (filerr == FILERR_NONE)
|
|
{
|
|
record.set_status(audit_record::STATUS_GOOD, audit_record::SUBSTATUS_GOOD);
|
|
found++;
|
|
}
|
|
else
|
|
record.set_status(audit_record::STATUS_NOT_FOUND, audit_record::SUBSTATUS_NOT_FOUND);
|
|
}
|
|
}
|
|
}
|
|
|
|
if (found == 0 && required > 0)
|
|
{
|
|
m_record_list.reset();
|
|
return NOTFOUND;
|
|
}
|
|
|
|
// return a summary
|
|
return summarize(m_enumerator.driver().name);
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// summary - generate a summary, with an optional
|
|
// string format
|
|
//-------------------------------------------------
|
|
|
|
media_auditor::summary media_auditor::summarize(const char *name, astring *string)
|
|
{
|
|
if (m_record_list.count() == 0)
|
|
{
|
|
return NONE_NEEDED;
|
|
}
|
|
|
|
// loop over records
|
|
summary overall_status = CORRECT;
|
|
for (audit_record *record = m_record_list.first(); record != NULL; record = record->next())
|
|
{
|
|
summary best_new_status = INCORRECT;
|
|
|
|
// skip anything that's fine
|
|
if (record->substatus() == audit_record::SUBSTATUS_GOOD)
|
|
continue;
|
|
|
|
// output the game name, file name, and length (if applicable)
|
|
if (string != NULL)
|
|
{
|
|
string->catprintf("%-12s: %s", name, record->name());
|
|
if (record->expected_length() > 0)
|
|
string->catprintf(" (%" I64FMT "d bytes)", record->expected_length());
|
|
string->catprintf(" - ");
|
|
}
|
|
|
|
// use the substatus for finer details
|
|
switch (record->substatus())
|
|
{
|
|
case audit_record::SUBSTATUS_GOOD_NEEDS_REDUMP:
|
|
if (string != NULL) string->catprintf("NEEDS REDUMP\n");
|
|
best_new_status = BEST_AVAILABLE;
|
|
break;
|
|
|
|
case audit_record::SUBSTATUS_FOUND_NODUMP:
|
|
if (string != NULL) string->catprintf("NO GOOD DUMP KNOWN\n");
|
|
best_new_status = BEST_AVAILABLE;
|
|
break;
|
|
|
|
case audit_record::SUBSTATUS_FOUND_BAD_CHECKSUM:
|
|
if (string != NULL)
|
|
{
|
|
astring tempstr;
|
|
string->catprintf("INCORRECT CHECKSUM:\n");
|
|
string->catprintf("EXPECTED: %s\n", record->expected_hashes().macro_string(tempstr));
|
|
string->catprintf(" FOUND: %s\n", record->actual_hashes().macro_string(tempstr));
|
|
}
|
|
break;
|
|
|
|
case audit_record::SUBSTATUS_FOUND_WRONG_LENGTH:
|
|
if (string != NULL) string->catprintf("INCORRECT LENGTH: %" I64FMT "d bytes\n", record->actual_length());
|
|
break;
|
|
|
|
case audit_record::SUBSTATUS_NOT_FOUND:
|
|
if (string != NULL)
|
|
{
|
|
device_t *shared_device = record->shared_device();
|
|
if (shared_device == NULL)
|
|
string->catprintf("NOT FOUND\n");
|
|
else
|
|
string->catprintf("NOT FOUND (%s)\n", shared_device->shortname());
|
|
}
|
|
break;
|
|
|
|
case audit_record::SUBSTATUS_NOT_FOUND_NODUMP:
|
|
if (string != NULL) string->catprintf("NOT FOUND - NO GOOD DUMP KNOWN\n");
|
|
best_new_status = BEST_AVAILABLE;
|
|
break;
|
|
|
|
case audit_record::SUBSTATUS_NOT_FOUND_OPTIONAL:
|
|
if (string != NULL) string->catprintf("NOT FOUND BUT OPTIONAL\n");
|
|
best_new_status = BEST_AVAILABLE;
|
|
break;
|
|
|
|
default:
|
|
assert(false);
|
|
}
|
|
|
|
// downgrade the overall status if necessary
|
|
overall_status = MAX(overall_status, best_new_status);
|
|
}
|
|
return overall_status;
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_one_rom - validate a single ROM entry
|
|
//-------------------------------------------------
|
|
|
|
audit_record *media_auditor::audit_one_rom(const rom_entry *rom)
|
|
{
|
|
// allocate and append a new record
|
|
audit_record &record = m_record_list.append(*global_alloc(audit_record(*rom, audit_record::MEDIA_ROM)));
|
|
|
|
// see if we have a CRC and extract it if so
|
|
UINT32 crc = 0;
|
|
bool has_crc = record.expected_hashes().crc(crc);
|
|
|
|
// find the file and checksum it, getting the file length along the way
|
|
emu_file file(m_enumerator.options().media_path(), OPEN_FLAG_READ | OPEN_FLAG_NO_PRELOAD);
|
|
path_iterator path(m_searchpath);
|
|
astring curpath;
|
|
while (path.next(curpath, record.name()))
|
|
{
|
|
// open the file if we can
|
|
file_error filerr;
|
|
if (has_crc)
|
|
filerr = file.open(curpath, crc);
|
|
else
|
|
filerr = file.open(curpath);
|
|
|
|
// if it worked, get the actual length and hashes, then stop
|
|
if (filerr == FILERR_NONE)
|
|
{
|
|
record.set_actual(file.hashes(m_validation), file.size());
|
|
break;
|
|
}
|
|
}
|
|
|
|
// compute the final status
|
|
compute_status(record, rom, record.actual_length() != 0);
|
|
return &record;
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_one_disk - validate a single disk entry
|
|
//-------------------------------------------------
|
|
|
|
audit_record *media_auditor::audit_one_disk(const rom_entry *rom, const char *locationtag)
|
|
{
|
|
// allocate and append a new record
|
|
audit_record &record = m_record_list.append(*global_alloc(audit_record(*rom, audit_record::MEDIA_DISK)));
|
|
|
|
// open the disk
|
|
chd_file source;
|
|
chd_error err = chd_error(open_disk_image(m_enumerator.options(), &m_enumerator.driver(), rom, source, locationtag));
|
|
|
|
// if we succeeded, get the hashes
|
|
if (err == CHDERR_NONE)
|
|
{
|
|
hash_collection hashes;
|
|
|
|
// if there's a SHA1 hash, add them to the output hash
|
|
if (source.sha1() != sha1_t::null)
|
|
hashes.add_sha1(source.sha1());
|
|
|
|
// update the actual values
|
|
record.set_actual(hashes);
|
|
}
|
|
|
|
// compute the final status
|
|
compute_status(record, rom, err == CHDERR_NONE);
|
|
return &record;
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// compute_status - compute a detailed status
|
|
// based on the information we have
|
|
//-------------------------------------------------
|
|
|
|
void media_auditor::compute_status(audit_record &record, const rom_entry *rom, bool found)
|
|
{
|
|
// if not found, provide more details
|
|
if (!found)
|
|
{
|
|
// no good dump
|
|
if (record.expected_hashes().flag(hash_collection::FLAG_NO_DUMP))
|
|
record.set_status(audit_record::STATUS_NOT_FOUND, audit_record::SUBSTATUS_NOT_FOUND_NODUMP);
|
|
|
|
// optional ROM
|
|
else if (ROM_ISOPTIONAL(rom))
|
|
record.set_status(audit_record::STATUS_NOT_FOUND, audit_record::SUBSTATUS_NOT_FOUND_OPTIONAL);
|
|
|
|
// just plain old not found
|
|
else
|
|
record.set_status(audit_record::STATUS_NOT_FOUND, audit_record::SUBSTATUS_NOT_FOUND);
|
|
}
|
|
|
|
// if found, provide more details
|
|
else
|
|
{
|
|
// length mismatch
|
|
if (record.expected_length() != record.actual_length())
|
|
record.set_status(audit_record::STATUS_FOUND_INVALID, audit_record::SUBSTATUS_FOUND_WRONG_LENGTH);
|
|
|
|
// found but needs a dump
|
|
else if (record.expected_hashes().flag(hash_collection::FLAG_NO_DUMP))
|
|
record.set_status(audit_record::STATUS_GOOD, audit_record::SUBSTATUS_FOUND_NODUMP);
|
|
|
|
// incorrect hash
|
|
else if (record.expected_hashes() != record.actual_hashes())
|
|
record.set_status(audit_record::STATUS_FOUND_INVALID, audit_record::SUBSTATUS_FOUND_BAD_CHECKSUM);
|
|
|
|
// correct hash but needs a redump
|
|
else if (record.expected_hashes().flag(hash_collection::FLAG_BAD_DUMP))
|
|
record.set_status(audit_record::STATUS_GOOD, audit_record::SUBSTATUS_GOOD_NEEDS_REDUMP);
|
|
|
|
// just plain old good
|
|
else
|
|
record.set_status(audit_record::STATUS_GOOD, audit_record::SUBSTATUS_GOOD);
|
|
}
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// find_shared_device - return the source that
|
|
// shares a media entry with the same hashes
|
|
//-------------------------------------------------
|
|
|
|
device_t *media_auditor::find_shared_device(device_t &device, const char *name, const hash_collection &romhashes, UINT64 romlength)
|
|
{
|
|
bool dumped = !romhashes.flag(hash_collection::FLAG_NO_DUMP);
|
|
|
|
// special case for non-root devices
|
|
device_t *highest_device = NULL;
|
|
if (device.owner() != NULL)
|
|
{
|
|
for (const rom_entry *region = rom_first_region(device); region != NULL; region = rom_next_region(region))
|
|
for (const rom_entry *rom = rom_first_file(region); rom != NULL; rom = rom_next_file(rom))
|
|
if (ROM_GETLENGTH(rom) == romlength)
|
|
{
|
|
hash_collection hashes(ROM_GETHASHDATA(rom));
|
|
if ((dumped && hashes == romhashes) || (!dumped && ROM_GETNAME(rom) == name))
|
|
highest_device = &device;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// iterate up the parent chain
|
|
for (int drvindex = m_enumerator.find(m_enumerator.driver().parent); drvindex != -1; drvindex = m_enumerator.find(m_enumerator.driver(drvindex).parent))
|
|
{
|
|
device_iterator deviter(m_enumerator.config(drvindex).root_device());
|
|
for (device_t *scandevice = deviter.first(); scandevice != NULL; scandevice = deviter.next())
|
|
for (const rom_entry *region = rom_first_region(*scandevice); region; region = rom_next_region(region))
|
|
for (const rom_entry *rom = rom_first_file(region); rom; rom = rom_next_file(rom))
|
|
if (ROM_GETLENGTH(rom) == romlength)
|
|
{
|
|
hash_collection hashes(ROM_GETHASHDATA(rom));
|
|
if ((dumped && hashes == romhashes) || (!dumped && ROM_GETNAME(rom) == name))
|
|
highest_device = scandevice;
|
|
}
|
|
}
|
|
}
|
|
|
|
return highest_device;
|
|
}
|
|
|
|
|
|
//-------------------------------------------------
|
|
// audit_record - constructor
|
|
//-------------------------------------------------
|
|
|
|
audit_record::audit_record(const rom_entry &media, media_type type)
|
|
: m_next(NULL),
|
|
m_type(type),
|
|
m_status(STATUS_ERROR),
|
|
m_substatus(SUBSTATUS_ERROR),
|
|
m_name(ROM_GETNAME(&media)),
|
|
m_explength(rom_file_size(&media)),
|
|
m_length(0),
|
|
m_shared_device(NULL)
|
|
{
|
|
m_exphashes.from_internal_string(ROM_GETHASHDATA(&media));
|
|
}
|
|
|
|
audit_record::audit_record(const char *name, media_type type)
|
|
: m_next(NULL),
|
|
m_type(type),
|
|
m_status(STATUS_ERROR),
|
|
m_substatus(SUBSTATUS_ERROR),
|
|
m_name(name),
|
|
m_explength(0),
|
|
m_length(0),
|
|
m_shared_device(NULL)
|
|
{
|
|
}
|