mirror of
https://github.com/holub/mame
synced 2025-05-22 05:38:52 +03:00
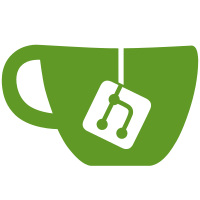
Use standard uint64_t, uint32_t, uint16_t or uint8_t instead of UINT64, UINT32, UINT16 or UINT8 also use standard int64_t, int32_t, int16_t or int8_t instead of INT64, INT32, INT16 or INT8
73 lines
1.8 KiB
C
73 lines
1.8 KiB
C
// license:BSD-3-Clause
|
|
// copyright-holders:Nathan Woods
|
|
/*********************************************************************
|
|
|
|
ioprocs.h
|
|
|
|
File IO abstraction layer
|
|
|
|
*********************************************************************/
|
|
|
|
#ifndef IOPROCS_H
|
|
#define IOPROCS_H
|
|
|
|
#include <stdlib.h>
|
|
|
|
|
|
|
|
/***************************************************************************
|
|
|
|
Type definitions
|
|
|
|
***************************************************************************/
|
|
|
|
struct io_procs
|
|
{
|
|
void (*closeproc)(void *file);
|
|
int (*seekproc)(void *file, int64_t offset, int whence);
|
|
size_t (*readproc)(void *file, void *buffer, size_t length);
|
|
size_t (*writeproc)(void *file, const void *buffer, size_t length);
|
|
uint64_t (*filesizeproc)(void *file);
|
|
};
|
|
|
|
|
|
|
|
struct io_generic
|
|
{
|
|
const struct io_procs *procs;
|
|
void *file;
|
|
uint8_t filler;
|
|
};
|
|
|
|
|
|
/***************************************************************************
|
|
|
|
Globals
|
|
|
|
***************************************************************************/
|
|
|
|
extern const struct io_procs stdio_ioprocs;
|
|
extern const struct io_procs stdio_ioprocs_noclose;
|
|
extern const struct io_procs corefile_ioprocs;
|
|
extern const struct io_procs corefile_ioprocs_noclose;
|
|
|
|
|
|
|
|
/***************************************************************************
|
|
|
|
Prototypes
|
|
|
|
***************************************************************************/
|
|
|
|
|
|
|
|
void io_generic_close(struct io_generic *genio);
|
|
void io_generic_read(struct io_generic *genio, void *buffer, uint64_t offset, size_t length);
|
|
void io_generic_write(struct io_generic *genio, const void *buffer, uint64_t offset, size_t length);
|
|
void io_generic_write_filler(struct io_generic *genio, uint8_t filler, uint64_t offset, size_t length);
|
|
uint64_t io_generic_size(struct io_generic *genio);
|
|
|
|
|
|
|
|
#endif /* IOPROCS_H */
|