mirror of
https://github.com/holub/mame
synced 2025-05-25 23:35:26 +03:00
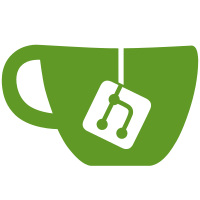
Use standard uint64_t, uint32_t, uint16_t or uint8_t instead of UINT64, UINT32, UINT16 or UINT8 also use standard int64_t, int32_t, int16_t or int8_t instead of INT64, INT32, INT16 or INT8
38 lines
886 B
C++
38 lines
886 B
C++
// license:BSD-3-Clause
|
|
// copyright-holders:Carl, Miodrag Milanovic
|
|
#ifndef __DINETWORK_H__
|
|
#define __DINETWORK_H__
|
|
|
|
class osd_netdev;
|
|
|
|
class device_network_interface : public device_interface
|
|
{
|
|
public:
|
|
device_network_interface(const machine_config &mconfig, device_t &device, float bandwidth);
|
|
virtual ~device_network_interface();
|
|
|
|
void set_interface(int id);
|
|
void set_promisc(bool promisc);
|
|
void set_mac(const char *mac);
|
|
|
|
const char *get_mac() const { return m_mac; }
|
|
bool get_promisc() const { return m_promisc; }
|
|
int get_interface() const { return m_intf; }
|
|
|
|
int send(uint8_t *buf, int len) const;
|
|
virtual void recv_cb(uint8_t *buf, int len);
|
|
|
|
protected:
|
|
bool m_promisc;
|
|
char m_mac[6];
|
|
float m_bandwidth;
|
|
std::unique_ptr<osd_netdev> m_dev;
|
|
int m_intf;
|
|
};
|
|
|
|
|
|
// iterator
|
|
typedef device_interface_iterator<device_network_interface> network_interface_iterator;
|
|
|
|
#endif
|