mirror of
https://github.com/holub/mame
synced 2025-04-25 17:56:43 +03:00
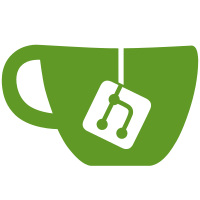
* Fixed bugs related to reloading roms Soft resets would reload autofire settings without saving them first, causing the settings to be lost. This commit adds a check to only reload from the settings file if loading a different rom than before. Hard resets would leave bad references lying around, causing MAME to crash under certain circumstances (i.e. resetting while in the edit menu and entering the menu again). This commit makes sure to properly clean up and reinitialize menu and button states when resetting. * Used set_folder to avoid hardcoding plugin name in settings path * Bumped autofire plugin version
93 lines
2.1 KiB
Lua
93 lines
2.1 KiB
Lua
local lib = {}
|
|
|
|
local plugin_path = ''
|
|
|
|
local function get_settings_path()
|
|
return plugin_path .. '/cfg/'
|
|
end
|
|
|
|
local function get_settings_filename()
|
|
return emu.romname() .. '.cfg'
|
|
end
|
|
|
|
local function initialize_button(settings)
|
|
if settings.port and settings.field and settings.key and settings.on_frames and settings.off_frames then
|
|
local new_button = {
|
|
port = settings.port,
|
|
field = settings.field,
|
|
key = manager:machine():input():code_from_token(settings.key),
|
|
on_frames = settings.on_frames,
|
|
off_frames = settings.off_frames,
|
|
counter = 0
|
|
}
|
|
local port = manager:machine():ioport().ports[settings.port]
|
|
if port then
|
|
local field = port.fields[settings.field]
|
|
if field then
|
|
new_button.button = field
|
|
return new_button
|
|
end
|
|
end
|
|
end
|
|
return nil
|
|
end
|
|
|
|
local function serialize_settings(button_list)
|
|
local settings = {}
|
|
for index, button in ipairs(button_list) do
|
|
setting = {
|
|
port = button.port,
|
|
field = button.field,
|
|
key = manager:machine():input():code_to_token(button.key),
|
|
on_frames = button.on_frames,
|
|
off_frames = button.off_frames
|
|
}
|
|
settings[#settings + 1] = setting
|
|
end
|
|
return settings
|
|
end
|
|
|
|
function lib:set_plugin_path(path)
|
|
plugin_path = path
|
|
end
|
|
|
|
function lib:load_settings()
|
|
local buttons = {}
|
|
local json = require('json')
|
|
local file = io.open(get_settings_path() .. get_settings_filename(), 'r')
|
|
if not file then
|
|
return buttons
|
|
end
|
|
local loaded_settings = json.parse(file:read('a'))
|
|
file:close()
|
|
if not loaded_settings then
|
|
return buttons
|
|
end
|
|
for index, button_settings in ipairs(loaded_settings) do
|
|
local new_button = initialize_button(button_settings)
|
|
if new_button then
|
|
buttons[#buttons + 1] = new_button
|
|
end
|
|
end
|
|
return buttons
|
|
end
|
|
|
|
function lib:save_settings(buttons)
|
|
local path = get_settings_path()
|
|
local attr = lfs.attributes(path)
|
|
if not attr then
|
|
lfs.mkdir(path)
|
|
elseif attr.mode ~= 'directory' then
|
|
return
|
|
end
|
|
local json = require('json')
|
|
local settings = serialize_settings(buttons)
|
|
local file = io.open(path .. get_settings_filename(), 'w')
|
|
if file then
|
|
file:write(json.stringify(settings, {indent = true}))
|
|
file:close()
|
|
end
|
|
end
|
|
|
|
return lib
|